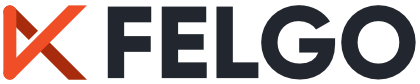
The GameStyle styles all Items which are derived from QtQuick Controls such as GameButton and GameSlider. If you want to change the style of the GUI elements, then you can create a CustomStyle and exchange the existing one. For small changes you can access the style directly and change the desired value. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
Note: Gaming controls are deprecated and will no longer receive updates and fixes. Instead, please use the Felgo App Controls.
You can access the currently active style via the settings.style
property. Following example changes the Background Color of the GUI Element.
import Felgo import QtQuick GameWindow { Scene { GameButton { text: "Random Color" onClicked: { settings.style.elementForegroundColor = utils.randomColor() } } } }
You can create a whole new style and override the GameStyle via the settings.style
property. Following example changes the complete style of the GUI elements and ItemEditor
back to the default QtQuick Controls Styles.
import Felgo import QtQuick GameWindow { // set custom style settings.style: CustomStyle { id: customStyle } Scene { Row { GameButton { onClicked: settings.style = customStyle text: "Change Style" } GameButton { onClicked: settings.style = settings.vplayStyle text: "Reset Style" } } } }
Content of the CustomStyle.qml
which uses the original QtQuick Controls Styles.
import Felgo import QtQuick import QtQuick.Controls.Styles Item { property alias buttonStyle:buttonStyleComponent property alias switchStyle:switchStyleComponent property alias sliderStyle:sliderStyleComponent property alias textFieldStyle:textFieldStyleComponent property alias scrollViewStyle:scrollViewStyleComponent property alias itemEditorStyle:itemEditorStyle Component { id: buttonStyleComponent ButtonStyle {} } Component { id: switchStyleComponent SwitchStyle {} } Component { id: sliderStyleComponent SliderStyle {} } Component { id: textFieldStyleComponent TextFieldStyle {} } Component { id: scrollViewStyleComponent ScrollViewStyle {} } ItemEditorStyle { id: itemEditorStyle } }
To work properly your custom style file needs to include all elements. Here is the GameStyle which can be used as reference.
Item { property alias buttonStyle:buttonStyleComponent property alias switchStyle:switchStyleComponent property alias sliderStyle:sliderStyleComponent property alias textFieldStyle:textFieldStyleComponent property alias scrollViewStyle:scrollViewStyleComponent property alias itemEditorStyle:itemEditorStyle property color windowBackgroundColor: "#212126" property color elementBackgroundColor: "#2c2b2a" property color elementForegroundColor: "#413d3c" property color elementHighlightColor: Qt.lighter("#468bb7", 1.2) property color elementNormalColor: Qt.darker("#468bb7", 1.4) property color buttonTextColor: "white" property int buttonTextPixelSize: 12 property color switchTextColor: "white" property int switchTextPixelSize: 11 property color textFieldTextColor: "white" property color textFieldPlaceholderTextColor: "grey" property int textFieldTextPixelSize: 14 property color itemEditorLabelTextColor: "white" property int itemEditorLabelTextPixelSize: 11 property alias itemEditorLabel: itemEditorLabel // Button Style Component { id: buttonStyleComponent ButtonStyle { id: buttonStyle background: Rectangle { id: buttonBackground implicitHeight: 30 color: control.pressed ? elementBackgroundColor : elementForegroundColor radius: height/4 Rectangle { anchors.fill: parent anchors.margins: 1 color: "transparent" border.color: control.hovered ? elementHighlightColor : elementNormalColor radius: height/4 } } label: Item { implicitWidth: buttonText.implicitWidth implicitHeight: buttonText.implicitHeight baselineOffset: buttonText.y + buttonText.baselineOffset Text { id: buttonText text: control.text anchors.centerIn: parent color: buttonTextColor font.pixelSize: buttonTextPixelSize // causes bad fonts and other problems http://qt-project.org/forums/viewthread/22158 //renderType: Text.NativeRendering renderType: Text.QtRendering } } } } // Switch Style Component { id: switchStyleComponent SwitchStyle { groove: Rectangle { implicitHeight: 25 implicitWidth: 76 radius: height/4 Rectangle { anchors.top: parent.top anchors.left: parent.left anchors.bottom: parent.bottom width: parent.width/2 - 1 height: 10 anchors.margins: 1 radius: height/4 color: control.checked ? elementHighlightColor : elementBackgroundColor Behavior on color {ColorAnimation {}} Text { font.pixelSize: switchTextPixelSize color: switchTextColor anchors.centerIn: parent text: "ON" } } Item { width: parent.width/2 height: parent.height anchors.right: parent.right Text { font.pixelSize: switchTextPixelSize color: switchTextColor anchors.centerIn: parent text: "OFF" } } Behavior on color {ColorAnimation {}} color: control.checked ? elementHighlightColor : elementBackgroundColor border.color: elementForegroundColor border.width: 1 } handle: Rectangle { width: parent.parent.width/2 height: control.height radius: height/4 color: control.pressed ? elementBackgroundColor : elementForegroundColor Rectangle { anchors.fill: parent anchors.margins: 1 color: "transparent" antialiasing: true border.color: control.checked ? elementHighlightColor : elementNormalColor radius: height/4 } } } } // Slider Style Component { id: sliderStyleComponent SliderStyle { handle: Rectangle { width: 30 height: 30 radius: height color: control.pressed ? elementBackgroundColor : elementForegroundColor Rectangle { anchors.fill: parent anchors.margins: 1 color: "transparent" antialiasing: true border.color: control.hovered ? elementHighlightColor : elementNormalColor radius: height/2 } } groove: Item { implicitHeight: 50 implicitWidth: 400 Rectangle { height: 8 width: parent.width anchors.verticalCenter: parent.verticalCenter color: elementBackgroundColor opacity: 0.8 Rectangle { antialiasing: true radius: 1 color: elementHighlightColor height: parent.height width: styleData.handlePosition } } } } } // TextField Style Component { id: textFieldStyleComponent TextFieldStyle { renderType: Text.QtRendering textColor: textFieldTextColor font.pixelSize: textFieldTextPixelSize placeholderTextColor: textFieldPlaceholderTextColor background: Rectangle { implicitWidth: Math.round(control.__contentHeight * 8) implicitHeight: Math.max(25, Math.round(control.__contentHeight * 1.2)) color: control.pressed ? elementBackgroundColor : elementForegroundColor radius: height/4 Rectangle { anchors.fill: parent anchors.margins: 1 color: "transparent" border.color: (control.hovered || control.activeFocus) ? elementHighlightColor : elementNormalColor radius: height/4 } } } } // ScrollView Style Component { id: scrollViewStyleComponent ScrollViewStyle { transientScrollBars: true handle: Item { implicitWidth: 7 implicitHeight: 13 Rectangle { color: elementBackgroundColor anchors.fill: parent anchors.topMargin: 3 anchors.leftMargin: 2 anchors.rightMargin: 2 anchors.bottomMargin: 3 radius: height/4 Rectangle { anchors.fill: parent anchors.margins: 1 color: "transparent" antialiasing: true border.color: elementHighlightColor radius: height/4 } } } scrollBarBackground: Item { implicitWidth: 7 implicitHeight: 13 } } } // ItemEditor Style ItemEditorStyle { id: itemEditorStyle contentDelegateBackground: Rectangle { color: windowBackgroundColor anchors.fill: parent radius: 2 } contentDelegateTypeList: Rectangle { color: windowBackgroundColor anchors.fill: parent radius: 2 } label: Text { id: itemEditorLabel color: itemEditorLabelTextColor font.pixelSize: itemEditorLabelTextPixelSize } } }
See also ItemEditor, GameButton, GameSlider, GameSwitch, GameTextField, and GameScrollView.
buttonStyle : alias |
This alias is used to access the buttonStyle. Used for instance in GameButton to assign the GameStyle to the QtQuick Controls Button element.
See also GameButton.
buttonTextColor : color |
This property is used to change the color of the GameButton text.
buttonTextPixelSize : int |
This property is used to change the pixel size of the GameButton text.
elementBackgroundColor : color |
This property is used to change the color of the background in GUI elements.
elementForegroundColor : color |
This property is used to change the color of the foreground in GUI elements.
elementHighlightColor : color |
This property is used to change the color of the highlight effects in GUI elements.
elementNormalColor : color |
This property is used to change the color of the highlight effects when the element is not hovered or pressed in GUI elements.
itemEditorLabel : alias |
This alias is used to change the font of the itemEditor label.
itemEditorLabelTextColor : color |
This property is used to change the color of the itemEditor label text.
itemEditorLabelTextPixelSize : int |
This property is used to change the pixel size of the itemEditor label text.
itemEditorStyle : alias |
This alias is used to access the itemEditorStyle. Used for instance in ItemEditor to assign the GameStyle to the QtQuick GUI elements.
See also ItemEditor and ItemEditorStyle.
scrollViewStyle : alias |
This alias is used to access the scrollViewStyle. Used for instance in GameScrollView to assign the GameStyle to the QtQuick ScrollView element.
See also GameScrollView.
sliderStyle : alias |
This alias is used to access the sliderStyle. Used for instance in GameSlider to assign the GameStyle to the QtQuick Controls Slider element.
See also GameSlider.
switchStyle : alias |
This alias is used to access the switchStyle. Used for instance in GameSwitch to assign the GameStyle to the QtQuick Controls Switch element.
See also GameSwitch.
switchTextColor : color |
This property is used to change the color of the GameSwitch text.
switchTextPixelSize : int |
This property is used to change the pixel size of the GameSwitch text.
textFieldPlaceholderTextColor : color |
This property is used to change the color of the placeholder text of the GameTextField.
textFieldStyle : alias |
This alias is used to access the textFieldStyle. Used for instance in GameTextField to assign the GameStyle to the QtQuick Controls TextField element.
See also GameTextField.
textFieldTextColor : color |
This property is used to change the color of the GameTextField text.
textFieldTextPixelSize : int |
This property is used to change the pixel size of the GameTextField text.
windowBackgroundColor : color |
This property is used to change the color of the window background in GUI elements and in the ItemEditor.
See also ItemEditor and ItemEditorStyle.