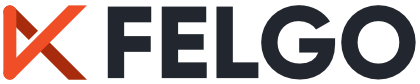
Allows to implement networking features based on the SuperAgent library from VisionMedia. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.18.1 |
The Felgo HttpRequest type integrates DuperAgent, a QML clone of the fantastic SuperAgent library from VisionMedia. The library is implemented in Qt/C++ and designed as an alternative to QML's builtin implementation of XmlHttpRequest.
The component is available as a singleton item and usable from all QML components that import Felgo via import Felgo
.
The currently used Duperagent version is v1.5.0
.
The QML API is almost identical to the JS API of SuperAgent. See the SuperAgent documentation for more API examples.
The following functions are not yet available with the DuperAgent QML port:
Note: One main difference to SuperAgent is that the returned error object has an additional property for the QNetworkReply::error() code. This error code can be checked against the documented Qt NetworkError types.
Use the HttpRequest::get() method to create a new request object. The returned object then allows to specify additional request settings or send the request. For example, use
the timeout function to specify the maximum request timeout. With a call of the then
or end
function you can send the request and
handle the result.
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .get("http://httpbin.org/get") .timeout(5000) .then(function(res) { console.log(res.status); console.log(JSON.stringify(res.header, null, 4)); console.log(JSON.stringify(res.body, null, 4)); }) .catch(function(err) { console.log(err.code) }); } }
This is how the same code looks like using the end()
function:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .get("http://httpbin.org/get") .timeout(5000) .end(function(err, res) { if(res.ok) { console.log(res.status); console.log(JSON.stringify(res.header, null, 4)); console.log(JSON.stringify(res.body, null, 4)); } else { console.log(err.message) console.log(err.response.text) } }); } }
DELETE
, HEAD
, PATCH
, POST
, and PUT
requests are also available with a simple change of the method name.
A typical JSON POST request might look a little like the following, where we set the Content-Type
header field appropriately, and "write" some data, in this case just a JSON string:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .post("https://jsonplaceholder.typicode.com/posts") .set('Content-Type', 'application/json') .send({ title: "post title", body: "post body" }) .then(function(res) { console.log(res.status); console.log(JSON.stringify(res.header, null, 4)); console.log(JSON.stringify(res.body, null, 4)); }) .catch(function(err) { console.log(err.code) }); } }
Since JSON is undoubtedly the most common, it's the default Content-Type
setting. You can also split the data into multiple send()
calls:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .post("https://jsonplaceholder.typicode.com/posts") .send({ title: "post title" }) .send({ body: "post body" }) .then(function(res) { console.log(res.status); console.log(JSON.stringify(res.header, null, 4)); console.log(JSON.stringify(res.body, null, 4)); }) .catch(function(err) { console.log(err.code) }); } }
For more information on the http features of SuperAgent, please see their documentation.
The HttpRequest methods like get() or post() present you with a request object. But the request is not sent right away. You can
specify additional settings for the request, before you send it and handle the result with the then
or end
function. You can use this to e.g specify a timeout or activate caching.
The caching API of HttpRequest is super useful to locally cache results of your requests as fallback in case of network or server issues.
To set up the cache, you can use the config() method:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest.config({ cache: true }); } }
By default the cache is active and not limited in size. To deactivate the cache, set a maximum size or change the cache location, see the config() options. You can also change the caching behavior of individual HttpRequest items with the following methods:
var cacheSave(boolean)
Overrides the cache save behavior for a single request. Setting it to true
will enable caching, while false
deactivates the cache.
var cacheLoad(HttpCacheControl)
This function is set to override the cache load behavior for a single request. It can have the following values:
You can use them as shown in this example:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .get("http://httpbin.org/get") .cacheSave(true) // cache the result of this request, regardless of global cache setting .cacheLoad(HttpCacheControl.PreferNetwork) // use network if possible, otherwise load from cache .then(function(res) { console.log(JSON.stringify(res.body)) }) .catch(function(err) { console.log(err.code) }); } }
Note: The cache is only used if there is a working network connection and the request fails. HttpRequest will not use the cache if your device has no connection to the internet at all or is in flight mode.
The following snippet shows how to attach files to the HttpRequest object.
Item { function uploadFile(serverUrl, fileName, fileUrl, callback) { HttpRequest.post(serverUrl) .attach("file", fileUrl, fileName) .end(function(err, res) { if(res.ok) { // upload successful let responseData = res.body } else { // upload failed console.error("Error: " + err.message) } }); } }
The request object you receive from e.g. HttpRequest::get or HttpRequest::post offers many functions for additional features
and configurations. For example, the cacheSave
, cacheLoad
, timeout
or attach
features that are used in the above code samples.
The API for the request object matches VisionMedia's SuperAgent library for JavaScript. The following methods are supported:
var use(var fn)
var timeout(int ms)
var clearTimeout()
var abort()
var set(var field, var val)
var unset(var field)
var type(var type)
var accept(var type)
var auth(string user, string password)
var redirects(int redirects)
var cacheSave(bool enabled)
var cacheLoad(int value)
var query(var query)
var field(var name, var value)
var attach(var name, var path, var filename)
var send(var data)
var withCredentials()
var on(var event, var handler)
var end(var callback)
var then(var onFulfilled, var onRejected)
Please see the SuperAgent documentation for more information and usage examples.
Similar to HttpRequest, which matches the DuperAgent Request
type, other DuperAgent components are also directly available in Felgo:
attach
function.
The DuperAgent package also contains an implementation of the Promises/A+ specification and offers an API similar to the Promises API in ES2017. For more information, please see MDN.
The Promise type works independently of DuperAgent's http features and does not require the Http
prefix. This is how you can create a Promise in
QML to handle the result of an HttpRequest:
import Felgo import QtQuick App { Component.onCompleted: { var p = Promise.create(function(resolve, reject) { // handle asynchronous code here // e.g. with asynchronous HttpRequest HttpRequest .get("http://httpbin.org/get") .timeout(5000) .end(function (err, res) { if(res.ok) resolve(res.body) else reject(err.message) }) }); // execute promise and handle result p.then(function(value) { // success console.log("Value: "+JSON.stringify(value)) }).catch(function(reason) { // failure console.log("Error: "+reason) }); } }
The following example combines multiple http requests with a promise, caching and an activity indicator:
import Felgo import QtQuick App { Component.onCompleted: { // remove activation delay to immediately show the indicator for requests // otherwise, requests that take less than the default 150 ms won't trigger the indicator HttpNetworkActivityIndicator.activationDelay = 0 // configure caching HttpRequest.config({ cache: { maxSize: 20000 } // max size of 200000 bytes }) } NavigationStack { AppPage { id: myPage title: "HttpRequest Example" rightBarItem: ActivityIndicatorBarItem { animating: HttpNetworkActivityIndicator.enabled visible: animating } // HttpNetworkActivityIndicator is not supported with Promises // this example thus uses a custom activity indicator handling property bool promisePending: false // function to send all requests and handle the result function sendAllRequests() { // trigger first request var p1 = HttpRequest .get("https://jsonplaceholder.typicode.com/todos/1") .cacheSave(false) // do not save response of first request to cache .cacheLoad(HttpCacheControl.AlwaysNetwork) // always load from network .then(function(res){ return res.body; }); // trigger second request var p2 = HttpRequest .get("https://jsonplaceholder.typicode.com/posts/1") .timeout(5000) .cacheLoad(HttpCacheControl.PreferCache) // use cache if possible, otherwise load from network .then(function(res){ return res.body; }); // create promise to check success of both requests var allRequests = Promise.all([p1, p2]) // handle promise result allRequests.then(function(values) { console.log("Both requests finished: "+JSON.stringify(values)) }).catch(function (err) { console.log("Error: "+err) }) } AppButton { text: "Send Get Requests" anchors.centerIn: parent onClicked: myPage.sendAllRequests() } } } }
The following example returns an object with error information in case the request fails and prints result:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .get("http://localhost:3002/test") .timeout(5000) .end(function(err, res) { if(res.ok) { console.log(res.status); console.log(JSON.stringify(res.header, null, 4)); console.log(JSON.stringify(res.body, null, 4)); } else { console.log("response", JSON.stringify(err.response)) } }); } }
cookie : var |
This property behaves similar to document.cookie
as implemented in browsers.
It returns all cookies in the cookie jar as a semi-colon separated string. Note that it does not return cookies that are marked as HttpOnly
.
Assigning a cookie to this property will add it to the cookie jar. If there is an existing cookie with the same identifying tuple, it will overwrite it. Note that it is not possible to overwrite cookies marked as
HttpOnly
. Attempts to overwrite these are silently ignored.
See also clearCookies.
void clearCookies() |
This function will clear all saved cookies including those marked as HttpOnly
.
See also cookie.
void config(var options) |
Allows to set global configuration options for the agent. This function should be called once before any requests are made. You can for example use the Component.onCompleted
signal of your App window to
call it on app start:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest.config({ cache: false }); } }
The following configuration options are supported:
This option controls the cache behavior of the agent. The default is to create a QNetworkDiskCache with a sensible path for the platform. If you wish to
disable this behavior, for example because you have your own cache, you can set this property to false
. The property can be further customized by passing an object.
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest.config({ cache: { maxSize: 20000, location: "/path/to/cache" } }); } }
The maxSize
option specifies the maximum size of the cache. To specify a custom directory for caching files, use the location
setting. The default location is
<CacheLocation>/duperagent
.
This option controls the cookie jar of the agent. The default is to create an instance of a QNetworkCookieJar that additionally persists the cookies to
disk. If you wish to disable this behavior, for example because you have your own cookie jar, you can set this property to false
. This property can be further customized by passing an object.
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest.config({ cookieJar: { location: "/path/to/cookies.txt" } }); } }
The location
option specifies the full path to the file to use for the disk storage. The default is <AppDataLocation>/duperagent_cookies.txt
.
This option controls the proxy settings used by agent. By default Qt does not use a proxy, however you can use the system proxy by setting this value to "system".
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest.config({ proxy: "system" }); } }
Creates an http GET
request for the given url. The data and callback parameters are optional. In case a callback
function is specified, the data
parameter may be
skipped.
The function returns a request object, which in turn allows to specify additional request settings or send the request. For example, you can use timeout
to specify the maximum request timeout. Then call the
end
function to send the request and handle the result.
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .get("http://httpbin.org/get") .timeout(5000) .end(function(err, res) { if(res.ok) { console.log(res.status); console.log(JSON.stringify(res.header, null, 4)); console.log(JSON.stringify(res.body, null, 4)); } else { console.log(err.message) console.log(err.response.text) } }); } }
Creates an http HEAD
request for the given url. The data and callback parameters are optional. In case a callback
function is specified, the data
parameter may be
skipped.
Similar to the HttpRequest::get() function, the returned request object allows to specify additional request settings or send the request.
Creates an http PATCH
request for the given url. The data and callback parameters are optional. In case a callback
function is specified, the data
parameter may
be skipped.
Similar to the HttpRequest::get() function, the returned request object allows to specify additional request settings or send the request.
Creates an http POST
request for the given url. The data and callback parameters are optional. In case a callback
function is specified, the data
parameter may be
skipped.
Similar to the HttpRequest::get() function, the returned request object allows to specify additional request settings or send the request.
Creates an http PUT
request for the given url. The data and callback parameters are optional. In case a callback
function is specified, the data
parameter may be
skipped.
Similar to the HttpRequest::get() function, the returned request object allows to specify additional request settings or send the request.