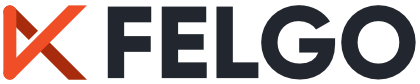
Qt is a great cross-platform framework for developing applications on Desktop, Mobile, Embedded and the Web. All the available C++ and Qt Quick components can run on each supported target platform. Still, certain features of the native platforms are only available with platform-specific APIs and frameworks.
The QML Native Code Components give you full native access, out of the box! They allow working with all native iOS and Android platform APIs directly from QML and provide a bridge between Qt Quick and the underlying native platform.
For example: To determine whether the iOS device that runs your app currently operates in a dark or light UI style (dark mode vs light mode), you only need few lines of code.
Note: There is also a more sophisticated example how to query and use dark mode on both iOS and Android in this blog post: Dark Mode with Qt on iOS and Android using Felgo Native Code Components
import Felgo import QtQuick App { NavigationStack { AppPage { title: qsTr("iOS Dark Mode Check") AppButton { anchors.centerIn: parent text: "Check iOS UI Style" onClicked: { // 1 is light mode, 2 is dark mode text = "UI Style: "+getDarkMode() } } } } function getDarkMode() { if(Qt.platform.os === "ios") { var uiApplicationClass = NativeObjectUtils.getClass("UIApplication") var application = uiApplicationClass.getStaticProperty("sharedApplication") var uiStyle = application.getProperty("keyWindow").getProperty("rootViewController").getProperty("traitCollection").getProperty("userInterfaceStyle") return uiStyle } else { // This example only includes iOS, to see both iOS and Android visit this example: // https://blog.felgo.com/qt-dark-mode-with-ios-and-android-native-components return 0 } } }
Creating native integrations is usually a complex task that involves different programming languages and implementations for each platform, as well as C++ wrapper types to provide the native features for usage with Qt Quick.
Felgo thus provides important platform features with the NativeUtils component and also offers Felgo Plugins for direct usage of 3rd party frameworks in QML. But there may be certain use-cases in your application that still require custom integrations of native code, for example to:
For implementing such integrations that would only be available with custom Java or Objective-C code, use the NativeObject item to call native APIs with QML and JavaScript. You no longer need to provide your own wrappers in C++ to translate between QML and native languages.
It is also possible to embed a native view as part of your Qt Quick scene. The NativeView type can render an Android or iOS view within your QML application. In contrast to Native App Integration for embedding Qt views in native apps, Native Components go the other direction and allow you to interact with native APIs and views directly from QML, using JavaScript. Felgo thus offers full flexibility to mix technologies and choose which part of your application uses Qt cross-platform code or platform-specific native code.
For example, you can add a native Button, ImageView or WebView to your Felgo app. This screenshot shows a sample app with these three components on Android and iOS:
Native components are a game-changer for applications that integrate tightly with the underlying target platform and bring many benefits for mobile app development with Qt:
See the QML Native Code Components documentation for more information and usage examples.
Represents a platform-native class |
|
Represents a platform-native object |
|
Allows you to interact with objects native to the mobile platform |
|
Allows to instantiate and display platform-specific views and widgets |
|
Defines the platform specifics for NativeView |