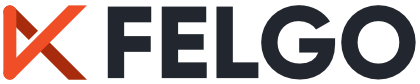
The TexturePackerSpriteSequence contains a list of TexturePackerSprite elements and allows switching between them with only one active at a time. It has an increased performance thanks to its support for sprite sheets created with TexturePacker. More...
Import Statement: | import Felgo 3.0 |
Inherits: |
The TexturePackerSpriteSequence component lets you control multiple sprite animations like the SpriteSequence. It supports sprite sheet created with TexturePacker. The TexturePackerSpriteSequence component contains a list of TexturePackerSprite and allows easy switching with only one active at a time. It also has an improved performance compared to the SpriteSequence and SpriteSequence components.
Read the guide How to use TexturePacker with Felgo to know why sprite sheets reduce the memory consumption and improve the performance of your game.
The TexturePackerSpriteSequence is a stochastic state machine. This means its states are the different sprites and a randomly chosen transition happens when a sprite finishes one animation cycle. The states are defined by the TexturePackerSprite::name property and the transitions are defined through the TexturePackerSprite::to property.
Here is an example of how to use two TexturePackerSprite in a TexturePackerSpriteSequence:
import Felgo 3.0 import QtQuick 2.0 GameWindow { Scene { TexturePackerSpriteSequence { TexturePackerSprite { name: "jump" source: "../assets/img/squaby.json" frameNames: ["squ1-jump-1.png", "squ1-jump-2.png", "squ1-jump-3.png", "squ1-jump-4.png"] frameRate: 3 to: {"jump": 1, "walk": 3} } TexturePackerSprite { name: "walk" source: "../assets/img/squaby.json" frameNames: ["squ1-walk-1.png", "squ1-walk-2.png", "squ1-walk-3.png", "squ1-walk-4.png"] frameRate: 3 to: {"jump": 1, "walk": 1} } } } }
This example will start with the first sprite called "jump". When this sprite has finished "walk" will be the next sprite with a probability of 3/4. The probability to stay in the "jump" sprite for one more cycle is the remaining 1/4. When the "walk" sprite finishes to probabilities for "jump" and "walk" are 50:50.
To change the active animation manually, use the jumpTo() function like in this example:
import Felgo 3.0 import QtQuick 2.0 GameWindow { Scene { TexturePackerSpriteSequence { id: squabySprite anchors.centerIn: parent TexturePackerSprite { name: "walk" source: "../assets/img/squaby.json" frameNames: ["squ1-walk-1.png", "squ1-walk-2.png", "squ1-walk-3.png", "squ1-walk-4.png"] frameRate: 3 } TexturePackerSprite { name: "jump" source: "../assets/img/squaby.json" frameNames: ["squ1-jump-1.png", "squ1-jump-2.png", "squ1-jump-3.png", "squ1-jump-4.png"] to: {"jump": 1, "walk": 3} frameRate: 3 } }// SpriteSequenceFromFile Row { anchors.bottom: parent.bottom // put on the bottom GameButton { text: "Jump" onClicked: { squabySprite.jumpTo("jump") } }// GameButton GameButton { text: "Walk" onClicked: { squabySprite.jumpTo("walk") } }// GameButton }// Row } }
This component supports content scaling to pick the best image quality for your screen density.
currentSprite : string |
The name of the TexturePackerSprite, which is currently animating.
mirror : bool |
This property holds whether the image should be horizontally mirrored.
The default value is false.
mirrorY : bool |
This property holds whether the image should be vertically mirrored.
Note: Mirroring on the vertical axis is internally achieved by setting the Item::transform property. Thus if you set the transform property explicitly, you need to apply the y-scaling manually.
The default value is false.
running : bool |
Whether the sprite is animating or not.
Default is true.
sprites : var |
A read-only list of TexturePackerSprite, which are the children of this TexturePackerSpriteSequence. These sprites will be scaled to the size of this item.
This function causes the TexturePackerSpriteSequence to jump to the specified sprite immediately. The sprite argument is the TexturePackerSprite::name of the sprite you wish to jump to.