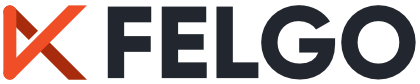
The WebStorage item syncs key-value-data with the cloud across devices and platforms with the FelgoGameNetwork. More...
Import Statement: | import Felgo 3.0 |
Inherits: |
The WebStorage component is part of the Felgo Game Network and can be used to
The WebStorage item is an extended version of the Storage item, with the addition that the key-value data is stored in the cloud. The API of WebStorage is the same as for Storage, which makes it easy to transition a previous code base using local data to cloud data and vice versa.
To sync data with the cloud across devices, each device must be connected with the same Facebook user. This connection can be done from the ProfileView in GameNetworkView.
Any data stored with setValue() is stored persistently to a local database and instantly available with getValue(). The data is then sent in the background to the server and if the last known client data is the same as on the server, onWriteValueFinished is called.
If the device has no Internet connection, all write requests are stored in a queue and sent automatically when network connection gets established again.
After connecting your player data with multiple devices and a sync between them, there might be conflicting data on the devices. Imagine the following scenario:
Device1 writes the value "Device1" for key "myKey". Device2 writes the value "Device2" for key "myKey". In such a conflict scenario, the data on each device and on the server depends on the set dataConflictStrategy. By default, the server will always contain the data of the last device, so in that case "Device2" will be stored on the server and "Device1" for "myKey" will be overwritten with "Device2" the next time the WebStorage syncs. A sync is initiated at every app start, and at every write request.
WebStorage has the huge benefit that it automatically merges conflicting data. By default, the server data will be merged with the client data. If the data cannot be merged as in the example above, the server data will be used
because of the default dataConflictStrategy set to "serverDataMergesClientData"
. See the property documentation of dataConflictStrategy for a detailed explanation of the different modes.
There are two use cases for WebStorage:
true
. Use this mode to synchronize user-based WebStorage data across devices. Example data are user settings like the chosen language,
or the music or vibration settings. Or save the date when the last app start was or how many credits a user has left for in-app purchases with the Store plugin.
This example shows how to sync the number of application starts across devices.
import Felgo 3.0 import QtQuick 2.0 GameWindow { WebStorage { id: myWebStorage // this can be read in the Text element below property int appStartedCounter onInitiallyInServerSyncOrErrorChanged: { // also increase the app counter, if there is no internet connection if(initiallyInServerSyncOrError) { increaseAppStartedCounter() } } function increaseAppStartedCounter() { var appStarts = myWebStorage.getValue("numAppStarts") // if the app was started for the first time, this will be undefined; in that case set it to 1 if(appStarts === undefined) { appStarts = 1 } else { appStarts++ } myWebStorage.setValue("numAppStarts", appStarts) // set the property to the stored value appStartedCounter = appStarts } } Facebook { // the user will automatically connect with facebook // once "connect" is pressed in the ProfileView in the GameNetworkView id: facebook // this is the Facebook App Id received from the settings in developers.facebook.com/apps appId: "489472511074353" readPermissions: ["email", "user_friends"] publishPermissions: ["publish_actions"] }// Facebook FelgoGameNetwork { id: gameNetwork // created in the Felgo Web Dashboard gameId: 5 secret: "abcdefg1234567890" gameNetworkView: myGameNetworkView facebookItem: facebook Component.onCompleted: { } }// FelgoGameNetwork Scene { id: scene // add your game functionality here // for this simple example we simulate the game logic with buttons // place the buttons below each other in a Column Column { SimpleButton { text: "Show ProfileView" onClicked: { // open the ProfileView of the GameNetworkView myGameNetworkView.show("profile") } } SimpleButton { // this shows the current appStartedCounter value in the button text: "Increase AppStartedCounter from current value " + myWebStorage.appStartedCounter onClicked: { // increase the current appcounter value by 1 myWebStorage.increaseAppStartedCounter() } } }// Column // the GameNetworkView will be shown on top of the buttons initially GameNetworkView { id: myGameNetworkView // use the whole GameWindow without borders on different aspect ratios anchors.fill: scene.gameWindowAnchorItem onShowCalled: { myGameNetworkView.visible = true } onBackClicked: { myGameNetworkView.visible = false } }// GameNetworkView }// Scene }// GameWindow
For a more detailed example, see the GameNetworkExample, in particular the KeyValueTest.
See also Storage.
clearAllAtStartup : bool |
Set this property temporarily to true
to clear all local data and also all web data at app startup. All previously stored web data is also removed, so use this property carefully.
This is only useful during testing, if you have faulty local and web data.
See also clearAll().
clearAllLocallyAtStartup : bool |
Set this property temporarily to true
to clear all local data at app startup. The storage will then be synced with the web contents and all local changes are lost.
This is only useful during testing, if you have faulty local data or want to simulate a fresh connection from a device.
See also clearAllLocally().
dataConflictStrategy : string |
This property can either have the value "serverDataMergesClientData"
, "clientDataMergesServerData"
, "serverDataOverwritesClientData"
, "clientDataOverwritesServerData"
, or
"userResolve"
. The default value is "serverDataMergesClientData"
, so the data from the server takes precedence over the client data.
Based on the dataConflictStrategy, these are the results for different scenarios:
Case 1: Values
Case 2: Maps
Case 3: Arrays
Case 4: Complex structure (mix of all above)
databaseName : string |
Set this property to store the key-value pairs to a database file with this name. By default, the databaseName is "defaultDBName"
. Multiple instances of the Storage element with the same databaseName have
access to the same data set.
Note: The GameWindow::settings property uses the databaseName "settings" internally, so do not use this value.
See also Storage::databaseName.
gameNetworkItem : variant |
Set this property to the id of Felgo Game Network.
By default, it will be set to the id gameNetwork
if one is found in the project, or it is undefined if no such id is found.
Note: It is required that a Felgo Game Network component exists for this component to synchronize the data with the web server.
This is an example how to use it:
// ... FelgoGameNetwork { id: myGameNetwork // other gameNetwork code here } WebStorage { id: myGameNetworkView gameNetworkItem: myGameNetwork } // ...
ignoredKeysForServerSyncMap : variant |
A map of keys that should not be synced with the server. These keys are not synced with the cloud and thus the WebStorage behaves like a normal Storage component without syncing them.
An example value is: {"ignoredKey1", "ignoredKey2"}
inServerSync : bool |
This read-only property gets true
when all read or write requests are handled and all data is in sync. It stays at the initial value false
when a connection error occurs and the write requests are
stored for later sending. It also changes to false
when data is written with setValue() or when a server value is synced initially at app startup, and also
after initiallyInServerSync is true
.
initiallyInServerSync : bool |
This read-only property gets true
the first time all the keys are synced with the server. At app start it is set to false
and stays false
if a connection error occurs.
This property is similar to inServerSync because it is also set to true
when all properties are in sync with server. The difference is that it does not
get set to false
at a further call of setValue(), but stays true
. It only gets false
when when the logged in player changes (e.g. at
a call of FelgoGameNetwork::connectFacebookUser() or FelgoGameNetwork::disconnectFacebookUser()).
It is also similar to initiallyInServerSyncOrError, with the difference it is only set to true
when no connection error occurred and all
key-value pairs are in sync.
See also initiallyInServerSyncOrError and inServerSync.
initiallyInServerSyncOrError : bool |
This read-only property gets true
the first time all the keys are synced with the server or there is no Internet connection.
See also initiallyInServerSync and inServerSync.
perUserStore : bool |
This property holds if it should sync across all devices of the same user (the default value true
), or sync across all users and all devices (set it to false
in that case).
See also WebStorage Per User or Per Application.
This handler is called when a value was successfully cleared from the server after an explicit call of clearValue().
The data argument has the following format:
{ "key": "testKey" }
Note: The corresponding handler is onClearValueFinished
.
This handler is called if a connection error occurred and thus the key-value pairs are not in sync with the server. You can for example show a dialog to the user if that happens.
Note: The corresponding handler is onErrorAndNotInitiallySynced
.
This handler is called when a value is read from the server either after an explicit call of getValue() or after syncing all keys at app start.
The data argument has the following format:
{ "key": "testKey", "value": "serverValue" }
Note: The corresponding handler is onReadValueFinished
.
This handler is called when a call of setValue() was not successful, because the server data got modified from another device compared to the (old) local data. If
dataConflictStrategy is set to "userResolve"
, the mergedData
is undefined but conflict
is true
.
Example value for data property:
{ "key": "testKey", "value": "serverValue", "updated_at": "2013-06-12T14:26:10Z", "client_value": "clientValue", "client_updated_at": "2013-06-11T14:26:10Z", "conflict": true, "mergedData": "serverValue" }
In this handler, you can call setValue() with your custom merged value if dataConflictStrategy is set to
"userResolve"
.
Note: The corresponding handler is onWriteConflict
.
This handler is called when a value was successfully written to the server after an explicit call of setValue().
The data argument has the following format:
{ "key": "testKey", "value": "serverValue" }
Note: The corresponding handler is onWriteValueFinished
.
Clears all values for all keys of this WebStorage locally and on the server. To only clear the local data but not on the server, call clearAllLocally().
Note: This clears all data locally and on the server, so if you provide this functionality in your game add at least a security check if the user really wants to do that.
Internally, this function calls clearValue() for all keys, so for each key a onClearValueFinished is called.
See also clearAllLocally(), Storage::clearAll(), and clearAllAtStartup.
Clears all the local data stored with WebStorage, without clearing the values on the server.
This is useful if you want to provide a logout functionality because the user for example sells the device, and does not want to keep his played data on the device. The server data, however, should not be deleted because the player might continue to play from another device and by connecting with Facebook he can then continue playing with the same server data.
The suggested workflow contains a messagebox to ask if the user wants to remove all his local player progress after a logout, which most of the time he will want to do. Otherwise the local data will be written and merged to the newly logged in user. Make sure to call the clearAllLocally() before the new user is logged in again, otherwise the local data was already written to the server.
Note: Highscores and achievements of FelgoGameNetwork are never stored locally and always get overwritten by the server data once another user logs in. So no dedicated removal of client data is required for higshcores and achievements.
See also clearAll(), Storage::clearAll(), and clearAllLocallyAtStartup.
Clears the value for the provided key so its value is undefined
.
If a callback function is provided, it is called after the data was cleared at the server.
The handler onClearValueFinished is also called after a successful write to the server.
See also getValue(), setValue(), clearValueFinished, writeConflict, and Storage::clearValue().
Returns the previously stored value with setValue() for key from the WebStorage or undefined
if nothing was
stored for this key before.
If a callback function is provided, it is called after the data was synced and possibly merged with the server.
The handler onReadValueFinished is also called after a successful write to the server.
See also setValue(), readValueFinished, and Storage::getValue().
Stores the value with the provided key permanently to the WebStorage. It is stored to the local database immediately and is accessible with getValue().
If a callback function is provided, it is called after the data was synced and possibly merged with the server.
The handler onWriteValueFinished is also called after a successful write to the server.
See also getValue(), writeValueFinished, writeConflict, and Storage::setValue().
Call this function to synchronize all local values with the server and auto-merge them based on the dataConflictStrategy.