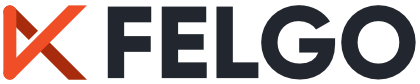
The ZeroConf singleton allows discovering network services via Bonjour/ZeroConf. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 4.0.0 |
This object is available as a singleton type from all QML components when Felgo is imported via import Felgo
. It is accessible with the name ZeroConf
. The ZeroConf
singleton allows
to discover network services in the local network via Bonjour/ZeroConf.
To start discovering services on supported devices on Android, iOS, and macOS, call startNetworkServiceDiscovery().
ZeroConf.startNetworkServiceDiscovery("_companion_link._tcp.", "local")
After the discovery starts, networkServiceDiscoveryActive is true
. Any additional calls to this method will fail until it is stopped with a
call to stopNetworkServiceDiscovery(). If the discovery fails to start it emits networkServiceDiscoveryFailed().
The following example discovers and lists services for all found types:
import QtQuick import Felgo App { id: app readonly property string serviceTypeAllServices: "_services._dns-sd._udp." readonly property bool searching: ZeroConf.networkServiceDiscoveryActive property var foundServices: ({}) Component.onCompleted: ZeroConf.startNetworkServiceDiscovery(serviceTypeAllServices) NavigationStack { id: stack ListPage { title: "Service types" emptyText.text: "Searching for service types..." model: Object.values(foundServices).filter(service => !service.resolved) delegate: AppListItem { text: modelData.name detailText: modelData.type onSelected: showServiceType(modelData) } } } Component { id: servicesPage ListPage { title: "Services for: " + serviceType required property string serviceType emptyText.text: "Searching for services..." onPushed: { console.log("Start search for type:", serviceType) ZeroConf.stopNetworkServiceDiscovery() ZeroConf.startNetworkServiceDiscovery(serviceType) } onPopped: { ZeroConf.stopNetworkServiceDiscovery() ZeroConf.startNetworkServiceDiscovery(serviceTypeAllServices) } model: Object.values(foundServices).filter(service => service.type === serviceType) delegate: AppListItem { text: modelData.name detailText: "Type: %1\nDomain: %2\nHost: %3\nPort: %4\nAddresses: %5\nAttributes: %6" .arg(modelData.type) .arg(modelData.domain) .arg(modelData.hostName) .arg(modelData.port) .arg(JSON.stringify(modelData.addresses, null, " ")) .arg(JSON.stringify(modelData.attributes, null, " ")) } } } Connections { target: ZeroConf function onNetworkServiceDiscovered(serviceData) { console.log("Network service discovered:", JSON.stringify(serviceData, null, " ")) foundServices[serviceData.name] = serviceData foundServicesChanged() } function onNetworkServiceRemoved(serviceData) { console.log("Network service removed:", JSON.stringify(serviceData, null, " ")) delete foundServices[serviceData.name] foundServicesChanged() } function onNetworkServiceDiscoveryFailed(errorData) { console.log("Network service search failed:", JSON.stringify(errorData, null, " ")) } } function showServiceType(serviceModel) { // the service search returns the type property like "_udp.local.", containing protocol and domain // split it to get the protocol, and add it to the service name var elems = serviceModel.type.split(".") var serviceType = "%1.%2.".arg(serviceModel.name).arg(elems[0]) stack.push(servicesPage, { serviceType: serviceType }) } }
Some platforms require additional integration steps to use network service discovery.
Using this method requires additional entries in your ios/Project-Info.plist
file. Add the NSLocalNetworkUsageDescription
with a description of how your app uses the local network. Add all service
types your app uses to the array NSBonjourServices
. If you search for services that are not listed, the discovery fails and emits networkServiceDiscoveryFailed(). Example:
<key>NSLocalNetworkUsageDescription</key> <string>Uses Bonjour to discover local network services.</string> <key>NSBonjourServices</key> <array> <string>_dns-sd._udp.</string> <string>_http._tcp.</string> <string>_http._udp.</string> <string>_companion-link._tcp.</string> <string>_mi-connect._udp.</string> </array>
No additional steps are required on Android.
No additional steps are required on macOS.
networkServiceDiscoveryActive : bool |
Readonly property to check if a network service discovery via Bonjour/ZeroConf is currently active.
You can start discovery with startNetworkServiceDiscovery(). Use stopNetworkServiceDiscovery() to stop or before starting a new discovery.
It emits new information with networkServiceDiscovered() and networkServiceRemoved().
See also startNetworkServiceDiscovery(), stopNetworkServiceDiscovery(), networkServiceDiscovered(), networkServiceRemoved(), and networkServiceDiscoveryFailed().
networkServiceDiscovered(var serviceData) |
Emitted after a new network service was discovered via Bonjour/ZeroConf.
You can start a search for local network services with startNetworkServiceDiscovery().
The parameter serviceData contains information about the discovered service. It is a JSON object with the following attributes:
name
- The service name.type
- The service type. This is usually the value supplied for the serviceType
parameter of startNetworkServiceDiscovery().
domain
- The service domain. This is only present on macOS and iOS and usually contains the string "local."
.resolved
- Contains true
if the service's host could be resolved.Resolved services will additionally contain these attributes:
hostName
- The service's host name. For example "My-Mac.local."
.port
- The service's port. For example 12345
.addresses
- A JSON array of the service's IP addresses. For example ["169.254.1.2", "fe80::424:abcd:1234:4628" ]
attributes
- A JSON map of the service's additional attributes. For example { "rpBA": "AB:CD:12:5E:8D:5D", "rpFl": "0x20000", "rpVr": "360.4"}
Note: The corresponding handler is onNetworkServiceDiscovered
.
See also networkServiceDiscoveryActive, startNetworkServiceDiscovery(), stopNetworkServiceDiscovery(), networkServiceRemoved(), and networkServiceDiscoveryFailed().
networkServiceDiscoveryFailed(var errorData) |
Emitted when network service discovery via Bonjour/ZeroConf fails to start.
This can happen if the parameters serviceType
and domain
are malformed. Note that a service type usually starts with underscore and ends with a trailing dot. Examples are
"_companion-link._tcp."
or "_services._dns-sd._udp."
.
The parameter errorData is a JSON object that contains information about the error that occured. It is passed through from the native platform. E.g. on macOS the error data could contain:
{ "NSNetServicesErrorCode": -72004, "NSNetServicesErrorDomain": 10 }
Note: The corresponding handler is onNetworkServiceDiscoveryFailed
.
See also networkServiceDiscoveryActive, startNetworkServiceDiscovery(), stopNetworkServiceDiscovery(), networkServiceDiscovered(), and networkServiceDiscoveryFailed().
networkServiceRemoved(var serviceData) |
Emitted after a previously discovered Bonjour/ZeroConf network service is no longer available.
You can start a search for local network services with startNetworkServiceDiscovery().
The parameter serviceData contains information about the removed service. It is a JSON object with the following attributes:
name
- The service name.type
- The service type. This is usually the value supplied for the serviceType
parameter of startNetworkServiceDiscovery().
domain
- The service domain. This is only present on macOS and iOS and usually contains the string "local."
.Note: The corresponding handler is onNetworkServiceRemoved
.
See also networkServiceDiscoveryActive, startNetworkServiceDiscovery(), stopNetworkServiceDiscovery(), networkServiceDiscovered(), and networkServiceDiscoveryFailed().
Starts discovering services in the local network via Bonjour/ZeroConf on supported devices on Android, iOS, and macOS.
ZeroConf.startNetworkServiceDiscovery("_companion_link._tcp.", "local")
It emits new information with networkServiceDiscovered() and networkServiceRemoved().
The parameter serviceType determines which type of service to discover. E.g, use "_companion_link._tcp."
to find local devices with the companion-link
TCP service. This would result in
calls to networkServiceDiscovered() with a JSON object like:
{ "addresses": [ "169.254.1.2", "fe80::424:abcd:1234:4628", "169.254.3.4", "fe80::18a1:abcd:1234:a81c" ], "attributes": { "rpAD": "8aa16bddcd51", "rpBA": "AB:CD:12:5E:8D:5D", "rpFl": "0x20000", "rpHN": "abcdefa1b11e", "rpMac": "0", "rpVr": "360.4" }, "domain": "local.", "hostName": "My-Mac.local.", "name": "My Mac", "port": 12345, "resolved": true, "type": "_companion-link._tcp." }
You can also use "_services._dns-sd._udp."
to search for all service types in the network. This would emit service types with JSON objects like the following:
{ "domain": ".", "errorData": { "NSNetServicesErrorCode": -72004, "NSNetServicesErrorDomain": 10 }, "name": "_remoted", "resolved": false, "type": "_tcp.local." }
The error is present because those are not actual resolved services. They give information about which service types are available in the network.
Note that the underscores and trailing dot in the service type are required.
The parameter domain determines the service search domain.
Note: This parameter is only relevant on macOS and iOS. You can omit it, it then uses the default "local"
domain on these operating systems.
After the discovery starts, networkServiceDiscoveryActive is true
. Any additional calls to this method will fail until it is stopped with a
call to stopNetworkServiceDiscovery(). If the discovery fails to start it emits networkServiceDiscoveryFailed().
See also networkServiceDiscoveryActive, stopNetworkServiceDiscovery(), networkServiceDiscovered(), networkServiceRemoved(), and networkServiceDiscoveryFailed().
void stopNetworkServiceDiscovery() |
Stop discovering services in the local network via Bonjour/ZeroConf.
This method fails unless a discovery is active after a call to startNetworkServiceDiscovery().
After stopping discovery, it sets networkServiceDiscoveryActive to false
.
See also networkServiceDiscoveryActive, startNetworkServiceDiscovery(), networkServiceDiscovered(), networkServiceRemoved(), and networkServiceDiscoveryFailed().