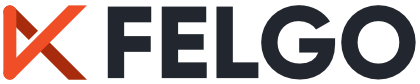
The section provides a comprehensive list of all new classes and functions introduced in Qt 6.0.
namespace | QNativeInterface |
namespace | QQuickOpenGLUtils |
Class QAbstractItemModel:
virtual bool | clearItemData(const QModelIndex &index) |
virtual void | multiData(const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const |
Class QAbstractItemView:
virtual void | initViewItemOption(QStyleOptionViewItem *option) const |
virtual QAbstractItemDelegate * | itemDelegateForIndex(const QModelIndex &index) const |
Class QAbstractProxyModel:
virtual bool | clearItemData(const QModelIndex &index) override |
Class QAction:
QList<QObject *> | associatedObjects() const |
Class QBitArray:
quint32 | toUInt32(QSysInfo::Endian endianness, bool *ok) const |
Class QBitmap:
QBitmap | fromPixmap(const QPixmap &pixmap) |
Class QBluetoothDeviceInfo:
QList<QBluetoothUuid> | serviceUuids() const |
Class QByteArray:
int | compare(QByteArrayView bv, Qt::CaseSensitivity cs) const |
bool | contains(QByteArrayView bv) const |
qsizetype | count(QByteArrayView bv) const |
bool | endsWith(QByteArrayView bv) const |
QByteArray | first(qsizetype n) const |
qsizetype | indexOf(QByteArrayView bv, qsizetype from) const |
QByteArray & | insert(qsizetype i, QByteArrayView data) |
QByteArray | last(qsizetype n) const |
qsizetype | lastIndexOf(QByteArrayView bv, qsizetype from) const |
void | push_back(QByteArrayView str) |
void | push_front(QByteArrayView str) |
QByteArray & | replace(QByteArrayView before, QByteArrayView after) |
QByteArray | sliced(qsizetype pos, qsizetype n) const |
QByteArray | sliced(qsizetype pos) const |
bool | startsWith(QByteArrayView bv) const |
Class QCanBusDeviceInfo:
QString | alias() const |
Class QChar:
Class QDataStream:
QDataStream & | operator<<(char16_t c) |
QDataStream & | operator<<(char32_t c) |
QDataStream & | operator<<(QDataStream &out, const std::pair<T1, T2> &pair) |
QDataStream & | operator>>(char16_t &c) |
QDataStream & | operator>>(char32_t &c) |
QDataStream & | operator>>(QDataStream &in, std::pair<T1, T2> &pair) |
Class QDate:
QDate | fromString(QStringView string, Qt::DateFormat format) |
QDate | fromString(QStringView string, QStringView format, QCalendar cal) |
QDate | fromString(const QString &string, QStringView format, QCalendar cal) |
Class QDateTime:
QDateTime | fromString(QStringView string, Qt::DateFormat format) |
QDateTime | fromString(QStringView string, QStringView format, QCalendar cal) |
QDateTime | fromString(const QString &string, QStringView format, QCalendar cal) |
Class QDebug:
QDebug & | operator<<(const char16_t *t) |
QDebug & | operator<<(QUtf8StringView s) |
QDebug & | operator<<(QByteArrayView t) |
QString | toString(T &&object) |
Class QDir:
void | addSearchPath(const QString &prefix, const std::filesystem::path &path) |
std::filesystem::path | filesystemAbsolutePath() const |
std::filesystem::path | filesystemCanonicalPath() const |
std::filesystem::path | filesystemPath() const |
void | setPath(const std::filesystem::path &path) |
Class QDropEvent:
Class QEvent:
virtual QEvent * | clone() const |
bool | isInputEvent() const |
bool | isPointerEvent() const |
bool | isSinglePointEvent() const |
Class QExplicitlySharedDataPointer:
Class QFile:
bool | copy(const std::filesystem::path &newName) |
std::filesystem::path | filesystemFileName() const |
bool | link(const std::filesystem::path &newName) |
QFileDevice::Permissions | permissions(const std::filesystem::path &filename) |
bool | rename(const std::filesystem::path &newName) |
void | setFileName(const std::filesystem::path &name) |
bool | setPermissions(const std::filesystem::path &filename, QFileDevice::Permissions permissionSpec) |
Class QFileInfo:
std::filesystem::path | filesystemAbsoluteFilePath() const |
std::filesystem::path | filesystemAbsolutePath() const |
std::filesystem::path | filesystemCanonicalFilePath() const |
std::filesystem::path | filesystemCanonicalPath() const |
std::filesystem::path | filesystemFilePath() const |
std::filesystem::path | filesystemPath() const |
std::filesystem::path | filesystemSymLinkTarget() const |
void | setFile(const std::filesystem::path &file) |
void | stat() |
Class QFuture:
bool | isSuspended() const |
bool | isSuspending() const |
bool | isValid() const |
QFuture<T> | onCanceled(Function &&handler) |
QFuture<T> | onFailed(Function &&handler) |
void | setSuspended(bool suspend) |
void | suspend() |
T | takeResult() |
QFuture<ResultType<Function>> | then(Function &&function) |
QFuture<ResultType<Function>> | then(QtFuture::Launch policy, Function &&function) |
QFuture<ResultType<Function>> | then(QThreadPool *pool, Function &&function) |
void | toggleSuspended() |
Class QFutureWatcher:
bool | isSuspended() const |
bool | isSuspending() const |
void | setSuspended(bool suspend) |
void | suspend() |
void | suspended() |
void | suspending() |
void | toggleSuspended() |
Class QGraphicsLayoutItem:
virtual bool | isEmpty() const |
Class QHash:
size_t | qHash(char8_t key, size_t seed) |
size_t | qHash(char16_t key, size_t seed) |
size_t | qHash(char32_t key, size_t seed) |
size_t | qHash(wchar_t key, size_t seed) |
size_t | qHash(std::nullptr_t key, size_t seed) |
size_t | qHash(QPoint key, size_t seed) |
size_t | qHash(const QTypeRevision &key, size_t seed) |
size_t | qHashMulti(size_t seed, const T &... args) |
size_t | qHashMultiCommutative(size_t seed, const T &... args) |
Class QHeaderView:
virtual void | initStyleOptionForIndex(QStyleOptionHeader *option, int logicalIndex) const |
Class QIODevice:
virtual qint64 | skipData(qint64 maxSize) |
Class QIcon:
QPixmap | pixmap(const QSize &size, qreal devicePixelRatio, QIcon::Mode mode, QIcon::State state) const |
Class QImage:
QImage | convertedTo(QImage::Format format, Qt::ImageConversionFlags flags) const & |
QImage | convertedTo(QImage::Format format, Qt::ImageConversionFlags flags) && |
QImage | fromHBITMAP(HBITMAP hbitmap) |
QImage | fromHICON(HICON icon) |
void | mirror(bool horizontal, bool vertical) |
void | rgbSwap() |
HBITMAP | toHBITMAP() const |
HICON | toHICON(const QImage &mask) const |
Class QImageIOHandler:
bool | allocateImage(QSize size, QImage::Format format, QImage *image) |
Class QImageReader:
int | allocationLimit() |
void | setAllocationLimit(int mbLimit) |
Class QInputEvent:
const QInputDevice * | device() const |
Class QJsonValue:
qint64 | toInteger(qint64 defaultValue) const |
Class QKeyEvent:
QKeyCombination | keyCombination() const |
Class QLabel:
QPicture | picture() const |
Class QLatin1StringView:
QLatin1StringView | first(qsizetype n) const |
QLatin1StringView | last(qsizetype n) const |
QLatin1StringView | sliced(qsizetype pos) const |
QLatin1StringView | sliced(qsizetype pos, qsizetype n) const |
QString | toString() const |
decltype(qTokenize(*this, std::forward<Needle>(needle), flags...)) | tokenize(Needle &&sep, Flags... flags) const |
Class QLibraryInfo:
QString | path(QLibraryInfo::LibraryPath p) |
Class QList:
void | append(QList<T> &&value) |
QList<T> | first(qsizetype n) const |
QList<T> | last(qsizetype n) const |
QList<T> | operator+(QList<T> &&other) const |
QList<T> & | operator+=(QList<T> &&other) |
QList<T> & | operator<<(QList<T> &&other) |
QList<T> | sliced(qsizetype pos, qsizetype n) const |
QList<T> | sliced(qsizetype pos) const |
Class QLocale:
QString | quoteString(QStringView str, QLocale::QuotationStyle style) const |
Class QMap:
QMap::iterator | erase(QMap::const_iterator first, QMap::const_iterator last) |
std::map<Key, T> | toStdMap() && |
Class QMargins:
QMargins | operator|(const QMargins &m1, const QMargins &m2) |
Class QMarginsF:
QMarginsF | operator|(const QMarginsF &m1, const QMarginsF &m2) |
Class QMetaMethod:
QMetaType | parameterMetaType(int index) const |
QByteArray | parameterTypeName(int index) const |
int | relativeMethodIndex() const |
QMetaType | returnMetaType() const |
Class QMetaProperty:
QUntypedBindable | bindable(QObject *object) const |
bool | isBindable() const |
QMetaType | metaType() const |
int | typeId() const |
Class QMetaSequence:
QMetaSequence | fromContainer() |
Class QMetaType:
qsizetype | alignOf() const |
QPartialOrdering | compare(const void *lhs, const void *rhs) const |
bool | equals(const void *lhs, const void *rhs) const |
bool | hasRegisteredDebugStreamOperator() const |
bool | hasRegisteredMutableViewFunction() |
bool | registerMutableView(To (From::*)() function) |
bool | registerMutableView(UnaryFunction function) |
bool | view(QMetaType fromType, void *from, QMetaType toType, void *to) |
Class QModbusReply:
QList<QModbusDevice::IntermediateError> | intermediateErrors() const |
Class QModelIndex:
void | multiData(QModelRoleDataSpan roleDataSpan) const |
Class QMultiHash:
QMultiHash<Key, T> & | unite(const QHash<Key, T> &other) |
Class QMultiMap:
QMultiMap::iterator | erase(QMultiMap::const_iterator first, QMultiMap::const_iterator last) |
Class QSGD3D11Texture:
QSGTexture * | fromNative(void *texture, QQuickWindow *window, const QSize &size, QQuickWindow::CreateTextureOptions options) |
Class QSGMetalTexture:
QSGTexture * | fromNative(int texture, QQuickWindow *window, const QSize &size, QQuickWindow::CreateTextureOptions options) |
Class QSGOpenGLTexture:
QSGTexture * | fromNative(GLuint textureId, QQuickWindow *window, const QSize &size, QQuickWindow::CreateTextureOptions options) |
Class QSGVulkanTexture:
QSGTexture * | fromNative(int image, int layout, QQuickWindow *window, const QSize &size, QQuickWindow::CreateTextureOptions options) |
Class QPagedPaintDevice:
QPageRanges | pageRanges() const |
virtual void | setPageRanges(const QPageRanges &ranges) |
Class QPersistentModelIndex:
void | multiData(QModelRoleDataSpan roleDataSpan) const |
Class QPointer:
T * | get() const |
Class QProcess:
std::function<void ()> | childProcessModifier() const |
void | setChildProcessModifier(const std::function<void ()> &modifier) |
void | startCommand(const QString &command, QIODeviceBase::OpenMode mode) |
Class QQmlApplicationEngine:
void | setExtraFileSelectors(const QStringList &extraFileSelectors) |
Class QQuickItem:
virtual void | geometryChange(const QRectF &newGeometry, const QRectF &oldGeometry) |
Class QQuickRenderControl:
void | beginFrame() |
void | endFrame() |
bool | initialize() |
int | samples() const |
void | setSamples(int sampleCount) |
QQuickWindow * | window() const |
Class QQuickWindow:
void | afterFrameEnd() |
void | beforeFrameBegin() |
QSGRendererInterface::GraphicsApi | graphicsApi() |
QQuickGraphicsConfiguration | graphicsConfiguration() const |
QQuickGraphicsDevice | graphicsDevice() const |
QQuickRenderTarget | renderTarget() const |
void | setGraphicsConfiguration(const QQuickGraphicsConfiguration &config) |
void | setGraphicsDevice(const QQuickGraphicsDevice &device) |
void | setRenderTarget(const QQuickRenderTarget &target) |
Class QRect:
QRect | span(const QPoint &p1, const QPoint &p2) |
Class QRegion:
Class QRegularExpression:
QRegularExpression | fromWildcard(QStringView pattern, Qt::CaseSensitivity cs, QRegularExpression::WildcardConversionOptions options) |
QRegularExpressionMatchIterator | globalMatch(QStringView subjectView, qsizetype offset, QRegularExpression::MatchType matchType, QRegularExpression::MatchOptions matchOptions) const |
QRegularExpressionMatch | match(QStringView subjectView, qsizetype offset, QRegularExpression::MatchType matchType, QRegularExpression::MatchOptions matchOptions) const |
Class QSGRenderNode:
virtual void | prepare() |
Class QSharedDataPointer:
Class QShortcut:
QList<QKeySequence> | keys() const |
void | setKeys(QKeySequence::StandardKey key) |
void | setKeys(const QList<QKeySequence> &keys) |
Class QSortFilterProxyModel:
void | autoAcceptChildRowsChanged(bool autoAcceptChildRows) |
void | invalidateColumnsFilter() |
void | invalidateRowsFilter() |
Class QSqlDriver:
virtual int | maximumIdentifierLength(QSqlDriver::IdentifierType type) const |
Class QSqlQuery:
QVariantList | boundValues() const |
Class QSslConfiguration:
bool | handshakeMustInterruptOnError() const |
bool | missingCertificateIsFatal() const |
void | setCiphers(const QString &ciphers) |
void | setHandshakeMustInterruptOnError(bool interrupt) |
void | setMissingCertificateIsFatal(bool cannotRecover) |
Class QSslSocket:
void | continueInterruptedHandshake() |
Class QStandardItem:
virtual void | multiData(QModelRoleDataSpan roleDataSpan) const |
Class QString:
QString & | append(QStringView v) |
qsizetype | count(QStringView str, Qt::CaseSensitivity cs) const |
QString | first(qsizetype n) const |
QString | fromLatin1(QByteArrayView str) |
QString | fromLocal8Bit(QByteArrayView str) |
QString | fromUtf8(QByteArrayView str) |
QString | fromUtf8(const char8_t *str, qsizetype size) |
QString & | insert(qsizetype position, QStringView str) |
QString | last(qsizetype n) const |
int | localeAwareCompare(QStringView other) const |
int | localeAwareCompare(QStringView s1, QStringView s2) |
QString & | operator+=(QStringView str) |
QString & | prepend(QStringView str) |
QString | sliced(qsizetype pos, qsizetype n) const |
QString | sliced(qsizetype pos) const |
decltype(qTokenize(*this, std::forward<Needle>(needle), flags...)) | tokenize(Needle &&sep, Flags... flags) const & |
decltype(qTokenize(std::move(*this), std::forward<Needle>(needle), flags...)) | tokenize(Needle &&sep, Flags... flags) const && |
decltype(qTokenize(std::move(*this), std::forward<Needle>(needle), flags...)) | tokenize(Needle &&sep, Flags... flags) && |
Class QStringListModel:
virtual bool | clearItemData(const QModelIndex &index) override |
Class QStringTokenizer:
decltype(QtPrivate::Tok::TokenizerResult<Haystack, Needle>({std::forward<Haystack>(h), std::forward<Needle>(n), flags...})) | qTokenize(Haystack &&haystack, Needle &&needle, Flags... flags) |
Class QStringView:
QStringView::const_pointer | constData() const |
qsizetype | count(QChar ch, Qt::CaseSensitivity cs) const |
qsizetype | count(QStringView str, Qt::CaseSensitivity cs) const |
QStringView | first(qsizetype n) const |
QStringView | last(qsizetype n) const |
QStringView | sliced(qsizetype pos, qsizetype n) const |
QStringView | sliced(qsizetype pos) const |
QList<QStringView> | split(QStringView sep, Qt::SplitBehavior behavior, Qt::CaseSensitivity cs) const |
QList<QStringView> | split(QChar sep, Qt::SplitBehavior behavior, Qt::CaseSensitivity cs) const |
QList<QStringView> | split(const QRegularExpression &re, Qt::SplitBehavior behavior) const |
CFStringRef | toCFString() const |
double | toDouble(bool *ok) const |
float | toFloat(bool *ok) const |
int | toInt(bool *ok, int base) const |
long | toLong(bool *ok, int base) const |
qlonglong | toLongLong(bool *ok, int base) const |
NSString * | toNSString() const |
short | toShort(bool *ok, int base) const |
uint | toUInt(bool *ok, int base) const |
ulong | toULong(bool *ok, int base) const |
qulonglong | toULongLong(bool *ok, int base) const |
ushort | toUShort(bool *ok, int base) const |
decltype(qTokenize(*this, std::forward<Needle>(needle), flags...)) | tokenize(Needle &&sep, Flags... flags) const |
Class QSurfaceFormat:
void | setColorSpace(const QColorSpace &colorSpace) |
Class QTextCharFormat:
qreal | baselineOffset() const |
void | setBaselineOffset(qreal baseline) |
void | setSubScriptBaseline(qreal baseline) |
void | setSuperScriptBaseline(qreal baseline) |
qreal | subScriptBaseline() const |
qreal | superScriptBaseline() const |
Class QTextDocument:
qreal | baselineOffset() const |
void | setBaselineOffset(qreal baseline) |
void | setSubScriptBaseline(qreal baseline) |
void | setSuperScriptBaseline(qreal baseline) |
qreal | subScriptBaseline() const |
qreal | superScriptBaseline() const |
Class QThreadPool:
bool | contains(const QThread *thread) const |
Class QTime:
QTime | fromString(QStringView string, Qt::DateFormat format) |
QTime | fromString(QStringView string, QStringView format) |
QTime | fromString(const QString &string, QStringView format) |
Class QTypeRevision:
QDataStream & | operator<<(QDataStream &out, const QTypeRevision &revision) |
QDataStream & | operator>>(QDataStream &in, QTypeRevision &revision) |
Class QUnhandledException:
Class QVarLengthArray:
QVarLengthArray<T, Prealloc> & | operator=(QVarLengthArray<T, Prealloc> &&other) |
Class QVariant:
bool | canConvert(QMetaType type) const |
QPartialOrdering | compare(const QVariant &lhs, const QVariant &rhs) |
bool | convert(QMetaType targetType) |
QMetaType | metaType() const |
Class QWidget:
QPointF | mapFrom(const QWidget *parent, const QPointF &pos) const |
QPointF | mapFromGlobal(const QPointF &pos) const |
QPointF | mapFromParent(const QPointF &pos) const |
QPointF | mapTo(const QWidget *parent, const QPointF &pos) const |
QPointF | mapToGlobal(const QPointF &pos) const |
QPointF | mapToParent(const QPointF &pos) const |
Class QWindow:
QPointF | mapFromGlobal(const QPointF &pos) const |
QPointF | mapToGlobal(const QPointF &pos) const |
virtual void | paintEvent(QPaintEvent *ev) |
void | resetOpenGLState() |
QTaskBuilder<Task> | task(Task &&task) |
long double | qDegreesToRadians(long double degrees) |
long double | qRadiansToDegrees(long double radians) |
QT_IMPLICIT_QCHAR_CONSTRUCTION | |
QT_IMPLICIT_QFILEINFO_CONSTRUCTION | |
Q_MOC_INCLUDE | |
Q_OBJECT_BINDABLE_PROPERTY(containingClass, type, name, signal) | |
Q_OBJECT_BINDABLE_PROPERTY_WITH_ARGS(containingClass, type, name, initialvalue, signal) | |
Q_OBJECT_COMPUTED_PROPERTY(containingClass, type, name, callback) |
enum | IntermediateError { ResponseCrcError, ResponseRequestMismatch } |
enum | WildcardConversionOption { DefaultWildcardConversion, UnanchoredWildcardConversion } |
enum class | AlertLevel { Warning, Fatal, Unknown } |
enum class | AlertType { CloseNotify, UnexpectedMessage, BadRecordMac, RecordOverflow, DecompressionFailure, …, UnknownAlertMessage } |
enum class | Launch { Sync, Async, Inherit } |
QML Type FontLoader:
font : font |
QML Type GeometryRenderer:
sortIndex : float |
QML Type Item:
palette : Palette |
QML Type SceneEnvironment:
tonemapMode : enumeration |
QML Type Window:
palette : Palette |