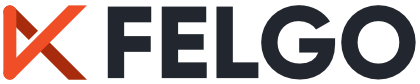
Encapsulates an HTTP request. More...
Header: | #include <QHttpServerRequest> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS HttpServer) target_link_libraries(mytarget PRIVATE Qt6::HttpServer) |
qmake: | QT += httpserver |
Since: | Qt 6.4 |
~QHttpServerRequest() | |
QByteArray | body() const |
QList<QPair<QByteArray, QByteArray>> | headers() const |
QHttpServerRequest::Method | method() const |
QUrlQuery | query() const |
QHostAddress | remoteAddress() const |
QUrl | url() const |
QByteArray | value(const QByteArray &key) const |
QDebug | operator<<(QDebug debug, const QHttpServerRequest &request) |
API for accessing the different parameters of an incoming request.
This enum type specifies an HTTP request method:
Constant | Value | Description |
---|---|---|
QHttpServerRequest::Method::Unknown |
0x0000 |
An unknown method. |
QHttpServerRequest::Method::Get |
0x0001 |
HTTP GET method. |
QHttpServerRequest::Method::Put |
0x0002 |
HTTP PUT method. |
QHttpServerRequest::Method::Delete |
0x0004 |
HTTP DELETE method. |
QHttpServerRequest::Method::Post |
0x0008 |
HTTP POST method. |
QHttpServerRequest::Method::Head |
0x0010 |
HTTP HEAD method. |
QHttpServerRequest::Method::Options |
0x0020 |
HTTP OPTIONS method. |
QHttpServerRequest::Method::Patch |
0x0040 |
HTTP PATCH method (RFC 5789). |
QHttpServerRequest::Method::Connect |
0x0080 |
HTTP CONNECT method. |
QHttpServerRequest::Method::Trace |
0x0100 |
HTTP TRACE method. |
QHttpServerRequest::Method::AnyKnown |
Get | Put | Delete | Post | Head | Options | Patch | Connect | Trace |
Combination of all known methods. |
The Methods type is a typedef for QFlags<Method>. It stores an OR combination of Method values.
Destroys a QHttpServerRequest
Returns the body of the request.
Returns all the request headers.
Returns the method of the request.
Returns the query in the request.
Returns the address of the origin host of the request.
Returns the URL the request asked for.
Returns the combined value of all headers with the named key.