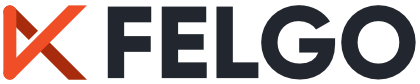
API for sending replies from an HTTP server. More...
Header: | #include <QHttpServerResponder> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS HttpServer) target_link_libraries(mytarget PRIVATE Qt6::HttpServer) |
qmake: | QT += httpserver |
Since: | Qt 6.4 |
HeaderList | |
enum class | StatusCode { Continue, SwitchingProtocols, Processing, Ok, Created, …, NetworkConnectTimeoutError } |
QHttpServerResponder(QHttpServerResponder &&other) | |
~QHttpServerResponder() | |
QTcpSocket * | socket() const |
void | write(QIODevice *data, QHttpServerResponder::HeaderList headers, QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | write(QIODevice *data, const QByteArray &mimeType, QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | write(const QJsonDocument &document, QHttpServerResponder::HeaderList headers, QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | write(const QJsonDocument &document, QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | write(const QByteArray &data, QHttpServerResponder::HeaderList headers, QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | write(const QByteArray &data, const QByteArray &mimeType, QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | write(QHttpServerResponder::HeaderList headers, QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | write(QHttpServerResponder::StatusCode status = StatusCode::Ok) |
void | writeBody(const char *body, qint64 size) |
void | writeBody(const char *body) |
void | writeBody(const QByteArray &body) |
void | writeHeader(const QByteArray &header, const QByteArray &value) |
void | writeHeaders(QHttpServerResponder::HeaderList headers) |
void | writeStatusLine(QHttpServerResponder::StatusCode status = StatusCode::Ok) |
Provides functions for writing back to an HTTP client with overloads for serializing JSON objects. It also has support for writing HTTP headers and status code. This class can be constructed by calling the
protected
static
function makeResponder()
in the QAbstractHttpServer class.
[alias]
QHttpServerResponder::HeaderListType alias for std::initializer_list<std::pair<QByteArray, QByteArray>>
HTTP status codes
Constant | Value |
---|---|
QHttpServerResponder::StatusCode::Continue |
100 |
QHttpServerResponder::StatusCode::SwitchingProtocols |
101 |
QHttpServerResponder::StatusCode::Processing |
102 |
QHttpServerResponder::StatusCode::Ok |
200 |
QHttpServerResponder::StatusCode::Created |
201 |
QHttpServerResponder::StatusCode::Accepted |
202 |
QHttpServerResponder::StatusCode::NonAuthoritativeInformation |
203 |
QHttpServerResponder::StatusCode::NoContent |
204 |
QHttpServerResponder::StatusCode::ResetContent |
205 |
QHttpServerResponder::StatusCode::PartialContent |
206 |
QHttpServerResponder::StatusCode::MultiStatus |
207 |
QHttpServerResponder::StatusCode::AlreadyReported |
208 |
QHttpServerResponder::StatusCode::IMUsed |
226 |
QHttpServerResponder::StatusCode::MultipleChoices |
300 |
QHttpServerResponder::StatusCode::MovedPermanently |
301 |
QHttpServerResponder::StatusCode::Found |
302 |
QHttpServerResponder::StatusCode::SeeOther |
303 |
QHttpServerResponder::StatusCode::NotModified |
304 |
QHttpServerResponder::StatusCode::UseProxy |
305 |
QHttpServerResponder::StatusCode::TemporaryRedirect |
307 |
QHttpServerResponder::StatusCode::PermanentRedirect |
308 |
QHttpServerResponder::StatusCode::BadRequest |
400 |
QHttpServerResponder::StatusCode::Unauthorized |
401 |
QHttpServerResponder::StatusCode::PaymentRequired |
402 |
QHttpServerResponder::StatusCode::Forbidden |
403 |
QHttpServerResponder::StatusCode::NotFound |
404 |
QHttpServerResponder::StatusCode::MethodNotAllowed |
405 |
QHttpServerResponder::StatusCode::NotAcceptable |
406 |
QHttpServerResponder::StatusCode::ProxyAuthenticationRequired |
407 |
QHttpServerResponder::StatusCode::RequestTimeout |
408 |
QHttpServerResponder::StatusCode::Conflict |
409 |
QHttpServerResponder::StatusCode::Gone |
410 |
QHttpServerResponder::StatusCode::LengthRequired |
411 |
QHttpServerResponder::StatusCode::PreconditionFailed |
412 |
QHttpServerResponder::StatusCode::PayloadTooLarge |
413 |
QHttpServerResponder::StatusCode::UriTooLong |
414 |
QHttpServerResponder::StatusCode::UnsupportedMediaType |
415 |
QHttpServerResponder::StatusCode::RequestRangeNotSatisfiable |
416 |
QHttpServerResponder::StatusCode::ExpectationFailed |
417 |
QHttpServerResponder::StatusCode::ImATeapot |
418 |
QHttpServerResponder::StatusCode::MisdirectedRequest |
421 |
QHttpServerResponder::StatusCode::UnprocessableEntity |
422 |
QHttpServerResponder::StatusCode::Locked |
423 |
QHttpServerResponder::StatusCode::FailedDependency |
424 |
QHttpServerResponder::StatusCode::UpgradeRequired |
426 |
QHttpServerResponder::StatusCode::PreconditionRequired |
428 |
QHttpServerResponder::StatusCode::TooManyRequests |
429 |
QHttpServerResponder::StatusCode::RequestHeaderFieldsTooLarge |
431 |
QHttpServerResponder::StatusCode::UnavailableForLegalReasons |
451 |
QHttpServerResponder::StatusCode::InternalServerError |
500 |
QHttpServerResponder::StatusCode::NotImplemented |
501 |
QHttpServerResponder::StatusCode::BadGateway |
502 |
QHttpServerResponder::StatusCode::ServiceUnavailable |
503 |
QHttpServerResponder::StatusCode::GatewayTimeout |
504 |
QHttpServerResponder::StatusCode::HttpVersionNotSupported |
505 |
QHttpServerResponder::StatusCode::VariantAlsoNegotiates |
506 |
QHttpServerResponder::StatusCode::InsufficientStorage |
507 |
QHttpServerResponder::StatusCode::LoopDetected |
508 |
QHttpServerResponder::StatusCode::NotExtended |
510 |
QHttpServerResponder::StatusCode::NetworkAuthenticationRequired |
511 |
QHttpServerResponder::StatusCode::NetworkConnectTimeoutError |
599 |
Move-constructs a QHttpServerResponder instance, making it point at the same object that other was pointing to.
Destroys a QHttpServerResponder.
Returns the socket used.
Answers a request with an HTTP status code status and HTTP headers headers. The I/O device data provides the body of the response. If data is sequential, the body of the message is sent in chunks: otherwise, the function assumes all the content is available and sends it all at once but the read is done in chunks.
Note: This function takes the ownership of data.
Answers a request with an HTTP status code status and a MIME type mimeType. The I/O device data provides the body of the response. If data is sequential, the body of the message is sent in chunks: otherwise, the function assumes all the content is available and sends it all at once but the read is done in chunks.
Note: This function takes the ownership of data.
Answers a request with an HTTP status code status, JSON document document and HTTP headers headers.
Note: This function sets HTTP Content-Type header as "application/json".
Answers a request with an HTTP status code status, and JSON document document.
Note: This function sets HTTP Content-Type header as "application/json".
Answers a request with an HTTP status code status, HTTP Headers headers and a body data.
Note: This function sets HTTP Content-Length header.
Answers a request with an HTTP status code status, a MIME type mimeType and a body data.
Answers a request with an HTTP status code status and HTTP Headers headers.
Answers a request with an HTTP status code status.
Note: This function sets HTTP Content-Type header as "application/x-empty".
This function writes HTTP body body with size size.
This function writes HTTP body body.
This function writes HTTP body body.
This function writes an HTTP header header with value.
This function writes HTTP headers headers.
This function writes HTTP status line with an HTTP status code status.