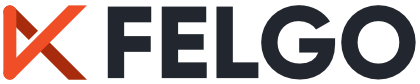
Contains miscellaneous identifiers used throughout the Qt MQTT module. More...
Header: | #include <QMqtt> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS Mqtt) target_link_libraries(mytarget PRIVATE Qt6::Mqtt) |
qmake: | QT += mqtt |
Since: | Qt 5.12 |
enum class | MessageStatus { Unknown, Published, Acknowledged, Received, Released, Completed } |
enum class | PayloadFormatIndicator { Unspecified, UTF8Encoded } |
enum class | ReasonCode { Success, SubscriptionQoSLevel0, SubscriptionQoSLevel1, SubscriptionQoSLevel2, NoMatchingSubscriber, …, WildCardSubscriptionsNotSupported } |
[since 5.12]
enum class QMqtt::MessageStatusThis enum type specifies the available states of a message. Depending on the QoS and role of the client, different message statuses are expected.
Constant | Value | Description |
---|---|---|
QMqtt::MessageStatus::Unknown |
0 |
The message status is unknown. |
QMqtt::MessageStatus::Published |
1 |
The client received a message for one of its subscriptions. This applies to QoS levels 1 and 2. |
QMqtt::MessageStatus::Acknowledged |
2 |
A message has been acknowledged. This applies to QoS 1 and states that the message handling has been finished from the client side. |
QMqtt::MessageStatus::Received |
3 |
A message has been received. This applies to QoS 2. |
QMqtt::MessageStatus::Released |
4 |
A message has been released. This applies to QoS 2. For a publisher the message handling has been finished. |
QMqtt::MessageStatus::Completed |
5 |
A message has been completed. This applies to QoS 2 and states that the message handling has been finished from the client side. |
This enum was introduced or modified in Qt 5.12.
[since 5.12]
enum class QMqtt::PayloadFormatIndicatorThe payload format provides information on the content of a message. This can help other clients to handle the message faster.
Constant | Value | Description |
---|---|---|
QMqtt::PayloadFormatIndicator::Unspecified |
0 |
The format is not specified. |
QMqtt::PayloadFormatIndicator::UTF8Encoded |
1 |
The payload of the message is formatted as UTF-8 Encoded Character Data. |
This enum was introduced or modified in Qt 5.12.
[since 5.12]
enum class QMqtt::ReasonCodeThis enum type specifies the available error codes.
Constant | Value | Description |
---|---|---|
QMqtt::ReasonCode::Success |
0 |
The specified action has succeeded. |
QMqtt::ReasonCode::SubscriptionQoSLevel0 |
0 |
A subscription with QoS level 0 has been created. |
QMqtt::ReasonCode::SubscriptionQoSLevel1 |
0x01 |
A subscription with QoS level 1 has been created. |
QMqtt::ReasonCode::SubscriptionQoSLevel2 |
0x02 |
A subscription with QoS level 2 has been created. |
QMqtt::ReasonCode::NoMatchingSubscriber |
0x10 |
The message has been accepted by the server, but there are no subscribers to receive this message. A broker may send this reason code instead of Success. |
QMqtt::ReasonCode::NoSubscriptionExisted (since Qt 5.15) |
0x11 |
No matching Topic Filter is being used by the Client. |
QMqtt::ReasonCode::ContinueAuthentication (since Qt 5.15) |
0x18 |
Continue the authentication with another step. |
QMqtt::ReasonCode::ReAuthenticate (since Qt 5.15) |
0x19 |
Initiate a re-authentication. |
QMqtt::ReasonCode::UnspecifiedError |
0x80 |
An unspecified error occurred. |
QMqtt::ReasonCode::MalformedPacket |
0x81 |
The packet sent to the server is invalid. |
QMqtt::ReasonCode::ProtocolError |
0x82 |
A protocol error has occurred. In most cases, this will cause the server to disconnect the client. |
QMqtt::ReasonCode::ImplementationSpecificError |
0x83 |
The packet is valid, but the recipient rejects it. |
QMqtt::ReasonCode::UnsupportedProtocolVersion |
0x84 |
The requested protocol version is not supported by the server. |
QMqtt::ReasonCode::InvalidClientId |
0x85 |
The client ID is invalid. |
QMqtt::ReasonCode::InvalidUserNameOrPassword |
0x86 |
The username or password specified is invalid. |
QMqtt::ReasonCode::NotAuthorized |
0x87 |
The client is not authorized for the specified action. |
QMqtt::ReasonCode::ServerNotAvailable |
0x88 |
The server to connect to is not available. |
QMqtt::ReasonCode::ServerBusy |
0x89 |
The server to connect to is not available. The client is asked to try at a later time. |
QMqtt::ReasonCode::ClientBanned |
0x8A |
The client has been banned from the server. |
QMqtt::ReasonCode::InvalidAuthenticationMethod |
0x8C |
The authentication method specified is invalid. |
QMqtt::ReasonCode::InvalidTopicFilter |
0x8F |
The topic filter specified is invalid. |
QMqtt::ReasonCode::InvalidTopicName |
0x90 |
The topic name specified is invalid. |
QMqtt::ReasonCode::MessageIdInUse |
0x91 |
The message ID used in the previous packet is already in use. |
QMqtt::ReasonCode::MessageIdNotFound |
0x92 |
The message ID used in the previous packet has not been found. |
QMqtt::ReasonCode::PacketTooLarge |
0x95 |
The packet received is too large. See also QMqttServerConnectionProperties::maximumPacketSize(). |
QMqtt::ReasonCode::QuotaExceeded |
0x97 |
An administratively imposed limit has been exceeded. |
QMqtt::ReasonCode::InvalidPayloadFormat |
0x99 |
The payload format is invalid. See also QMqttPublishProperties::payloadFormatIndicator(). |
QMqtt::ReasonCode::RetainNotSupported |
0x9A |
The server does not support retained messages. See also QMqttServerConnectionProperties::retainAvailable(). |
QMqtt::ReasonCode::QoSNotSupported |
0x9B |
The QoS level requested is not supported. See also QMqttServerConnectionProperties::maximumQoS(). |
QMqtt::ReasonCode::UseAnotherServer |
0x9C |
The server the client tries to connect to is not available. See also QMqttServerConnectionProperties::serverReference(). |
QMqtt::ReasonCode::ServerMoved |
0x9D |
The server the client tries to connect to has moved to a new address. See also QMqttServerConnectionProperties::serverReference(). |
QMqtt::ReasonCode::SharedSubscriptionsNotSupported |
0x9E |
Shared subscriptions are not supported. See also QMqttServerConnectionProperties::sharedSubscriptionSupported(). |
QMqtt::ReasonCode::ExceededConnectionRate |
0x9F |
The connection rate limit has been exceeded. |
QMqtt::ReasonCode::SubscriptionIdsNotSupported |
0xA1 |
Subscription IDs are not supported. See also QMqttServerConnectionProperties::subscriptionIdentifierSupported(). |
QMqtt::ReasonCode::WildCardSubscriptionsNotSupported |
0xA2 |
Subscriptions using wildcards are not supported by the server. See also QMqttServerConnectionProperties::wildcardSupported(). |
Not all values are available in every use case. Especially, some servers will reject a reason code not suited for a specific command. See below table to highlight expected reason codes for specific actions.
Reason Code | Connect Properties | Subscription Properties | Message Properties |
---|---|---|---|
Success | X | X | X |
SubscriptionQoSLevel0 | X | ||
SubscriptionQoSLevel1 | X | ||
SubscriptionQoSLevel2 | X | ||
NoMatchingSubscriber | X | ||
UnspecifiedError | X | X | X |
MalformedPacket | X | ||
ProtocolError | X | ||
ImplementationSpecificError | X | X | X |
UnsupportedProtocolVersion | X | ||
InvalidClientId | X | ||
InvalidUserNameOrPassword | X | ||
NotAuthorized | X | X | X |
ServerNotAvailable | X | ||
ServerBusy | X | ||
ClientBanned | X | ||
InvalidAuthenticationMethod | X | ||
InvalidTopicFilter | X | ||
InvalidTopicName | X | X | |
MessageIdInUse | X | X | |
MessageIdNotFound | X | ||
PacketTooLarge | X | ||
QuotaExceeded | X | X | X |
InvalidPayloadFormat | X | X | |
RetainNotSupported | X | ||
QoSNotSupported | X | ||
UseAnotherServer | X | ||
ServerMoved | X | ||
SharedSubscriptionsNotSupported | X | ||
ExceededConnectionRate | X | ||
SubscriptionIdsNotSupported | X | ||
WildCardSubscriptionsNotSupported | X |
This enum was introduced or modified in Qt 5.12.