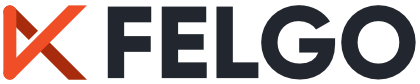
The OPC UA EndpointDescription. More...
Header: | #include <QOpcUaEndpointDescription> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS OpcUa) target_link_libraries(mytarget PRIVATE Qt6::OpcUa) |
qmake: | QT += opcua |
enum | MessageSecurityMode { Invalid, None, Sign, SignAndEncrypt } |
|
|
QOpcUaEndpointDescription(const QOpcUaEndpointDescription &rhs) | |
QOpcUaEndpointDescription & | operator=(const QOpcUaEndpointDescription &rhs) |
QString | endpointUrl() const |
quint8 | securityLevel() const |
QOpcUaEndpointDescription::MessageSecurityMode | securityMode() const |
QString | securityPolicy() const |
QOpcUaApplicationDescription | server() const |
QByteArray | serverCertificate() const |
QOpcUaApplicationDescription & | serverRef() |
void | setEndpointUrl(const QString &endpointUrl) |
void | setSecurityLevel(quint8 securityLevel) |
void | setSecurityMode(QOpcUaEndpointDescription::MessageSecurityMode securityMode) |
void | setSecurityPolicy(const QString &securityPolicy) |
void | setServer(const QOpcUaApplicationDescription &server) |
void | setServerCertificate(const QByteArray &serverCertificate) |
void | setTransportProfileUri(const QString &transportProfileUri) |
void | setUserIdentityTokens(const QList<QOpcUaUserTokenPolicy> &userIdentityTokens) |
QString | transportProfileUri() const |
QList<QOpcUaUserTokenPolicy> | userIdentityTokens() const |
QList<QOpcUaUserTokenPolicy> & | userIdentityTokensRef() |
bool | operator==(const QOpcUaEndpointDescription &rhs) const |
An endpoint description contains information about an endpoint and how to connect to it.
This enum type holds the security mode supported by the endpoint.
Constant | Value | Description |
---|---|---|
QOpcUaEndpointDescription::Invalid |
0 |
The default value, will be rejected by the server. |
QOpcUaEndpointDescription::None |
1 |
No security. |
QOpcUaEndpointDescription::Sign |
2 |
Messages are signed but not encrypted. |
QOpcUaEndpointDescription::SignAndEncrypt |
3 |
Messages are signed and encrypted. |
[read-only]
endpointUrl : const QStringThe URL for the endpoint.
Access functions:
QString | endpointUrl() const |
[read-only]
securityMode : const QOpcUaEndpointDescription::MessageSecurityModeSecurity mode supported by this endpoint.
Access functions:
QOpcUaEndpointDescription::MessageSecurityMode | securityMode() const |
[read-only]
securityPolicy : const QStringThe URI of the security policy.
Access functions:
QString | securityPolicy() const |
[read-only]
server : const QOpcUaApplicationDescriptionThe application description of the server.
Access functions:
QOpcUaApplicationDescription | server() const |
[read-only]
userIdentityTokens : const QList<QOpcUaUserTokenPolicy>List of user identity tokens the endpoint will accept.
Access functions:
QList<QOpcUaUserTokenPolicy> | userIdentityTokens() const |
Constructs an endpoint description from rhs.
Sets the values from rhs in this endpoint description.
Returns the URL for the endpoint.
Note: Getter function for property endpointUrl.
See also setEndpointUrl().
Returns a relative index assigned by the server. It describes how secure this endpoint is compared to other endpoints of the same server. An endpoint with strong security measures has a higher security level than one with weaker or no security measures.
Security level 0 indicates an endpoint for backward compatibility purposes which should only be used if the client does not support the security measures required by more secure endpoints.
See also setSecurityLevel().
Returns the security mode supported by this endpoint.
Note: Getter function for property securityMode.
See also setSecurityMode().
Returns the URI of the security policy.
Note: Getter function for property securityPolicy.
See also setSecurityPolicy().
Returns the application description of the server.
Note: Getter function for property server.
See also setServer().
Returns the application instance certificate of the server.
See also setServerCertificate().
Returns a reference to the application description of the server.
Sets the URL for the endpoint to endpointUrl.
See also endpointUrl().
Sets the security level to securityLevel.
See also securityLevel().
Sets the security mode supported by this endpoint to securityMode.
See also securityMode().
Sets the URI of the security policy to securityPolicy.
See also securityPolicy().
Sets the application description of the server to server.
See also server().
Sets the application instance certificate of the server to serverCertificate.
See also serverCertificate().
Sets the URI of the transport profile supported by the endpoint to transportProfileUri.
See also transportProfileUri().
Sets the user identity tokens to userIdentityTokens.
See also userIdentityTokens().
Returns the URI of the transport profile supported by the endpoint.
See also setTransportProfileUri().
Returns a list of user identity tokens the endpoint will accept.
Note: Getter function for property userIdentityTokens.
See also setUserIdentityTokens().
Returns a reference to a list of user identity tokens the endpoint will accept.
Returns true
if this endpoint description has the same value as rhs.