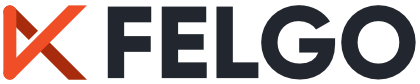
Provides a data store for raw data to later be used as vertices or uniforms. More...
Header: | #include <Qt3DCore/QBuffer> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS 3dcore) target_link_libraries(mytarget PRIVATE Qt6::3dcore) |
qmake: | QT += 3dcore |
Instantiated By: | Buffer |
Inherits: | Qt3DCore::QNode |
enum | AccessType { Write, Read, ReadWrite } |
enum | UsageType { StreamDraw, StreamRead, StreamCopy, StaticDraw, StaticRead, …, DynamicCopy } |
QBuffer(Qt3DCore::QNode *parent = nullptr) | |
Qt3DCore::QBuffer::AccessType | accessType() const |
QByteArray | data() const |
void | setData(const QByteArray &bytes) |
void | updateData(int offset, const QByteArray &bytes) |
Qt3DCore::QBuffer::UsageType | usage() const |
void | setAccessType(Qt3DCore::QBuffer::AccessType access) |
void | setUsage(Qt3DCore::QBuffer::UsageType usage) |
void | accessTypeChanged(Qt3DCore::QBuffer::AccessType access) |
void | dataAvailable() |
void | dataChanged(const QByteArray &bytes) |
void | usageChanged(Qt3DCore::QBuffer::UsageType usage) |
Data can be provided directly using QBuffer::setData().
Constant | Value | Description |
---|---|---|
Qt3DCore::QBuffer::Write |
0x1 |
Write access |
Qt3DCore::QBuffer::Read |
0x2 |
Read access |
Qt3DCore::QBuffer::ReadWrite |
Write|Read |
Write|Read |
The type of the usage.
Constant | Value | Description |
---|---|---|
Qt3DCore::QBuffer::StreamDraw |
0x88E0 |
GL_STREAM_DRAW |
Qt3DCore::QBuffer::StreamRead |
0x88E1 |
GL_STREAM_READ |
Qt3DCore::QBuffer::StreamCopy |
0x88E2 |
GL_STREAM_COPY |
Qt3DCore::QBuffer::StaticDraw |
0x88E4 |
GL_STATIC_DRAW |
Qt3DCore::QBuffer::StaticRead |
0x88E5 |
GL_STATIC_READ |
Qt3DCore::QBuffer::StaticCopy |
0x88E6 |
GL_STATIC_COPY |
Qt3DCore::QBuffer::DynamicDraw |
0x88E8 |
GL_DYNAMIC_DRAW |
Qt3DCore::QBuffer::DynamicRead |
0x88E9 |
GL_DYNAMIC_READ |
Qt3DCore::QBuffer::DynamicCopy |
0x88EA |
GL_DYNAMIC_COPY |
Returns the AccessType of the buffer.
Access functions:
Qt3DCore::QBuffer::AccessType | accessType() const |
void | setAccessType(Qt3DCore::QBuffer::AccessType access) |
Notifier signal:
void | accessTypeChanged(Qt3DCore::QBuffer::AccessType access) |
See also QBuffer::AccessType.
Holds the buffer usage.
Access functions:
Qt3DCore::QBuffer::UsageType | usage() const |
void | setUsage(Qt3DCore::QBuffer::UsageType usage) |
Notifier signal:
void | usageChanged(Qt3DCore::QBuffer::UsageType usage) |
Constructs a new QBuffer with parent.
[signal]
void QBuffer::dataAvailable()This signal is emitted when data becomes available.
[signal]
void QBuffer::dataChanged(const QByteArray
&bytes)This signal is emitted with bytes when data changes.
Returns the data.
See also setData().
Sets bytes as data.
See also data().
[invokable]
void QBuffer::updateData(int offset, const QByteArray &bytes)Updates the data by replacing it with bytes at offset.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.