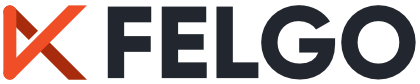
An example of reading and writing NFC Data Exchange Format (NDEF) messages to NFC Forum Tags.
The NDEF Editor example reads and writes NFC Data Exchange Format (NDEF) messages to NFC Forum Tags. NDEF messages can be composed by adding records of supported types. Additionally, NDEF messages can be loaded/saved from/into a file located in the file system of the device where the application is running.
The MainWindow
class is able to detect if an NFC Tag is in the range for read/write operations. It can also detect if the connection has been lost. This is achieved by connecting the MainWindow
class private handlers to the QNearFieldManager::targetDetected and QNearFieldManager::targetLost signals.
m_manager = new QNearFieldManager(this); connect(m_manager, &QNearFieldManager::targetDetected, this, &MainWindow::targetDetected); connect(m_manager, &QNearFieldManager::targetLost, this, &MainWindow::targetLost);
When Read or Write button is pressed, the detection of NFC tags is started by calling the QNearFieldManager::startTargetDetection method.
m_manager->startTargetDetection(QNearFieldTarget::NdefAccess);
Once the target is detected, the MainWindow
connects the following signals to its internal private slots: QNearFieldTarget::ndefMessageRead, QNearFieldTarget::NdefReadError, QNearFieldTarget::requestCompleted, QNearFieldTarget::NdefWriteError and QNearFieldTarget::error.
void MainWindow::targetDetected(QNearFieldTarget *target) { switch (m_touchAction) { case NoAction: break; case ReadNdef: connect(target, &QNearFieldTarget::ndefMessageRead, this, &MainWindow::ndefMessageRead); connect(target, &QNearFieldTarget::error, this, &MainWindow::targetError); m_request = target->readNdefMessages(); if (!m_request.isValid()) // cannot read messages targetError(QNearFieldTarget::NdefReadError, m_request); break; case WriteNdef: connect(target, &QNearFieldTarget::requestCompleted, this, &MainWindow::ndefMessageWritten); connect(target, &QNearFieldTarget::error, this, &MainWindow::targetError); m_request = target->writeNdefMessages(QList<QNdefMessage>() << ndefMessage()); if (!m_request.isValid()) // cannot write messages targetError(QNearFieldTarget::NdefWriteError, m_request); break; } }
If during the process of reading or writing to an NFC Tag the connection is lost, the MainWindow
reacts to this event by scheduling the target deletion (using QObject::deleteLater).
void MainWindow::targetLost(QNearFieldTarget *target) { target->deleteLater(); }
The main window of the NDEF Editor example manages the composition and creation of NFC records. The UI contains a QScrollArea, which is used to dynamically add the record editors. The
following methods of the MainWindow
class provide an interface towards each of the record editing classes managing the different types of records.
void addNfcTextRecord(); void addNfcUriRecord(); void addMimeImageRecord(); void addEmptyRecord();
The following sections explain each of the record editing classes.
The TextRecordEditor
is a QWidget that allows to edit the contents of the NDEF Text record. A new instance of this class is created for each text record.
class TextRecordEditor : public QWidget { Q_OBJECT public: explicit TextRecordEditor(QWidget *parent = 0); ~TextRecordEditor(); void setRecord(const QNdefNfcTextRecord &textRecord); QNdefNfcTextRecord record() const; private: Ui::TextRecordEditor *ui; };
The UriRecordEditor
is a QWidget that allows to edit the contents of the NDEF Uri record. A new instance of this class is created for each uri record.
class UriRecordEditor : public QWidget { Q_OBJECT public: explicit UriRecordEditor(QWidget *parent = 0); ~UriRecordEditor(); void setRecord(const QNdefNfcUriRecord &uriRecord); QNdefNfcUriRecord record() const; private: Ui::UriRecordEditor *ui; };
The MimeImageRecordEditor
is a QWidget that allows to edit the contents of the NDEF MIME record. In this example MIME record can be used to store an icon. A new instance of this
class is created for each MIME record.
class MimeImageRecordEditor : public QWidget { Q_OBJECT public: explicit MimeImageRecordEditor(QWidget *parent = 0); ~MimeImageRecordEditor(); void setRecord(const QNdefRecord &record); QNdefRecord record() const; public slots: void handleScreenOrientationChange(Qt::ScreenOrientation orientation); protected: void resizeEvent(QResizeEvent *) override; private: void updatePixmap(); Ui::MimeImageRecordEditor *ui; QNdefRecord m_record; QPixmap m_pixmap; bool m_imageSelected = false; bool m_screenRotated = false; private slots: void on_mimeImageOpen_clicked(); };
To run the example from Qt Creator, open the Welcome mode and select the example from Examples. For more information, visit Building and Running an Example.
See also Qt NFC.