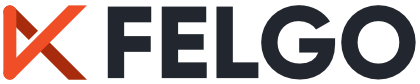
The base class for custom QML levels that are loaded in a game with LevelLoader. More...
Import Statement: | import Felgo 3.0 |
Inherits: |
The LevelBase is the base class for levels defined in QML that get loaded with LevelLoader from the LevelEditor.
It has the advantage that the levelData is available as a property after it got loaded and you can add custom data to be saved when the onLevelAboutToBeSaved handler occurs.
Also see the LevelLoader and LevelEditor for further information.
Every game will have different level properties. Whatever these are, you can use the loading functionality of LevelEditor and LevelEditor to simplify creation and loading of levels. The following example shows the definition of two different QML levels for a game:
// Level01.qml: LevelBase { property int startGold: 30 } // Level02.qml: LevelBase { property int startGold: 50 }
This property holds the loaded LevelData object once it got loaded with LevelEditor::loadSingleLevel().
You can access the levelData to display levelMetaData like the levelName for example. The following Level shows how to display the current loaded levelName with a Text item:
import Felgo 3.0 import QtQuick 2.0 LevelBase { Text { text: levelData.levelMetaData.levelName } }
This handler is called when the LevelBase has the chance to modify customData or levelMetaData of levelData. Thus you can store customData to the level by setting levelData.customData or changing levelData.levelMetaData.
Alternatively, customData can be added to LevelEditor::saveCurrentLevel with the saveProperties
parameter.
Note: The custom properties from saveProperties will overwrite the ones specified here! But usually it is more convenient to set the customData in the derived LevelBase, as you have direct access to the level properties.
The following order is applied when LevelEditor::saveCurrentLevel() is called:
The following example shows how to save and load the startGold property to customData when the level is stored:
LevelBase { property int startGold: 30 onLevelAboutToBeSaved: { // store the current startGold level that might have been changed by the game to the level levelData.customData = { startGoldLevel: startGold } } onLevelLoaded: { // load the stored startGold value startGold = levelData.customData.startGoldLevel } }
Note: The corresponding handler is onLevelAboutToBeSaved
.
See also levelLoaded.
This handler is called when customData and levelMetaData of levelData are loaded from LevelEditor.
The following order is applied when LevelEditor::saveCurrentLevel() is called:
See the onLevelAboutToBeSaved handler for an example how to store and load customData to a level.
Note: The corresponding handler is onLevelLoaded
.
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: