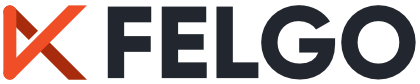
The BackgroundMusic element allows playing long-lasting and looping background sound in wav, mp3 or ogg file format. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
This component allows to play looping audio files, mostly used as background music in games. Background musics are usually longer-lasting tracks in encoded and compressed audio formats like ogg or MP3. However, the BackgroundMusic component can also play wav files. See Supported Audio Formats for more details.
Note: If you have multiple BackgroundMusic objects in your game, set the autoPlay property to false except for the first one you would like to play, to avoid automatically playing all of them when they get loaded.
For short notifications sounds with low latency, use GameSoundEffect. For videos use the Video component.
BackgroundMusic enhances the Qt component MediaPlayer in the Qt Multimedia 5.0 module with the following additions:
onStopped
handler.
settings.musicEnbaled
to true or false.This type allows you to play compressed audio files (typically ogg or MP3 files but also others like AAC or WAV are supported). Compared to GameSoundEffect, it takes less resources as it does not provide such a low latency to play a sound after calling play(). Thus use it to play longer-lasting tracks.
For free sounds, we recommend the following websites:
These sites have a great search feature, as you can search by categories or tags. Most of the sounds are available for free if you add the license to your game. There also are low-price premium version of the sounds, which allows you to use them without any license note.
The different supported platforms use different APIs for playing background music, therefore not all file formats are supported over all platforms. It's recommended to use the following file formats:
Platforms | Supported Audio Formats |
---|---|
Windows | wav, mp3 |
All other platforms | wav, mp3, ogg |
The music is preloaded (the autoLoad property is true by default) and is started automatically by default after loading it. You can change that auto-play behavior by setting autoPlay to false
.
For manual playback control, start the music with play().
Try setting the bitrate and the sampling rate as low as possible, so it still sounds reasonable. That helps in saving precious memory and runtime performance. Also mono channel sounds are preferred for performance reasons. The background musics used in the demo projects have a bitrate of 48kb/s and a 22kHz sampling rate.
Here is an example of a background music that is played when the qml file is loaded:
BackgroundMusic { id: backgroundMusic source: Qt.resolvedUrl("../assets/snd/backgroundMusic.mp3") // autoPlay: true - this is set by default }
autoPauseInBackground : bool |
Set this property to automatically pause this BackgroundMusic when the app is moved to background.
On mobile that happens if the user presses the home button and leaves the app. On desktop that happens when the app loses its focus or gets minimized.
By default, this value is true on mobile platforms and false on desktop platforms.
autoPlay : bool |
Set this property to play the background music automatically when this item is loaded, e.g. at application start.
By default, it is set to true. So when the settings.musicEnabled property is also set to true, the music is played after initialization of the Settings object.
See also GameWindowApplicationWindow::settings.
bufferProgress : real |
This property holds how much of the data buffer is currently filled, from 0.0 (empty) to 1.0 (full).
duration : int |
This property holds the duration of the media in milliseconds.
If the media doesn't have a fixed duration (a live stream for example) this will be 0.
error : enumeration |
This property holds the error state of the audio. It can be one of:
Value | Description |
---|---|
NoError | There is no current error. |
ResourceError | The audio cannot be played due to a problem allocating resources. |
FormatError | The audio format is not supported. |
NetworkError | The audio cannot be played due to network issues. |
AccessDeniedError | The audio cannot be played due to insufficient permissions. |
Note: For accessing the enum values like MediaPlayer.NoError
, add this import to your QML file: import QtMultimedia
.
errorString : string |
This property holds a string describing the current error condition in more detail.
hasAudio : bool |
This property holds whether the media contains audio.
hasVideo : bool |
This property holds whether the media contains video.
loops : alias |
This property provides a way to control the number of times to repeat the sound on each play().
By default, loops is set to MediaPlayer.Infinite
and therefore plays the sound infinitely until it gets stopped by calling stop().
Note: To have access to the MediaPlayer.Infinite
enum, make sure to call import QtMultimedia 6
in the header of your QML file.
metaData : alias |
See the MediaPlayer documentation which metaData properties are available.
See also QMediaMetaData.
muted : bool |
This property holds whether the background music is muted.
Defaults to false, and can be set to true or by setting the volume to 0.
playbackRate : real |
This property holds the rate at which audio is played at as a multiple of the normal rate.
Defaults to 1.0.
playbackState : enumeration |
This property holds the state of media playback. It can be one of:
Note: For accessing the enum values like MediaPlayer.PlayingState
, add this import to your QML file: import QtMultimedia
.
playing : bool |
This property holds whether the background music is currently playing.
This property is read-only.
See also play().
position : int |
seekable : bool |
This property holds whether position of the audio can be changed.
If true, calling the seek() method will cause playback to seek to the new position.
source : url |
This property holds the source URL of the media.
The default is an empty url, so this is a required property.
status : int |
This property indicates the current status of the BackgroundMusic. Possible statuses are listed below.
Value | Description |
---|---|
MediaPlayer.Null | No source has been set or the source is null. |
MediaPlayer.Loading | The Audio is trying to load the source. |
MediaPlayer.Ready | The source is loaded and ready for play. |
MediaPlayer.Error | An error occurred during operation, such as failure of loading the source. |
This property is read-only.
Note: For accessing one of the enum values like MediaPlayer.LoadedMedia
, add this import to your QML file: import QtMultimedia
.
volume : real |
This property holds the volume of the music output, from 0.0 (silent) to 1.0 (maximum volume).
Note: The volume property affects ALL Sounds and the BackgroundMusic instances on Windows, but not on mobile platforms. So the last volume setting that is defined is used for all sounds in the application.
The default value is 1.
See also muted.
paused() |
started() |
stopped() |
pause() |
Pauses playback of the media.
Sets the playing property to false. It also sets the playbackState property to
MediaPlayer.PausedState
.
See also play().
play() |
Starts playback of the media if the settings.musicEnabled property is true (which is the default).
Sets the playing property to true. It also sets the playbackState property to
MediaPlayer.PlayingState
.
seek(offset) |
stop() |
Stops playback of the media.
Sets the playing property to false. It also sets the playbackState property to
MediaPlayer.StoppedState
.
See also play().