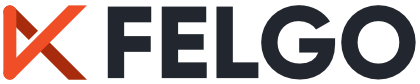
Offers an API similar to the Promises API in ES2017. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.18.1 |
The DuperAgent package, which brings the HttpRequest type, also contains an implementation of the Promises/A+ specification and offers an API
similar to the Promises API in ES2017. For more information, please see MDN. The Promise type works independently of DuperAgent's http features and does not require the Http
prefix.
It is available as a singleton item and usable from all QML components that import Felgo via import Felgo
.
This is how you can create a Promise in QML to handle the result of an HttpRequest:
import Felgo import QtQuick App { Component.onCompleted: { var p = Promise.create(function(resolve, reject) { // handle asynchronous code here // e.g. with asynchronous HttpRequest HttpRequest .get("http://httpbin.org/get") .timeout(5000) .end(function (err, res) { if(res.ok) resolve(res.body) else reject(err.message) }) }); // execute promise and handle result p.then(function(value) { // success console.log("Value: "+JSON.stringify(value)) }).catch(function(reason) { // failure console.log("Error: "+reason) }); } }
Promises are a convenient way to handle asynchronous aspects of your application. Each Promise wraps your asynchronous code, so you can handle the result in a flexible way.
For example, it is also possible to concurrently execute several promises and go on with your code if all promises resolved without issue:
import Felgo import QtQuick App { Component.onCompleted: { var p1 = Promise.resolve(3); var p2 = 1337; var p3 = HttpRequest .get("http://httpbin.org/get") .then(function(resp) { return resp.body; }); var p4 = Promise.all([p1, p2, p3]); p4.then(function(values) { console.log(values[0]); // 3 console.log(values[1]); // 1337 console.log(values[2]); // resp.body }); } }
If one of the original promises fail, the returned promise will be rejected with the same reason.
Concurrently executes several promises and returns another promise that is fulfilled when the original promises are fulfilled. The resolved value is an array containing the values of the original promises in the original order (not the order they were fulfilled).
If one of the original promises fail, the returned promise will be rejected with the same reason.
import Felgo import QtQuick App { Component.onCompleted: { var p1 = Promise.resolve(3); var p2 = 1337; var p3 = HttpRequest .get("http://httpbin.org/get") .then(function(resp) { return resp.body; }); var p4 = Promise.all([p1, p2, p3]); p4.then(function(values) { console.log(values[0]); // 3 console.log(values[1]); // 1337 console.log(values[2]); // resp.body }); } }
Creates a new Promise object.
Since the Javascript engine in QML does not allow us to expose new types with constructors, the new Promise(executor) syntax is not supported. Instead the create function can be used:
import Felgo import QtQuick App { Component.onCompleted: { var p = Promise.create(function(resolve, reject) { // handle asynchronous code here // e.g. with asynchronous HttpRequest HttpRequest .get("http://httpbin.org/get") .timeout(5000) .end(function (err, res) { if(res.ok) resolve(res.body) else reject(err.message) }) }); // execute promise and handle result p.then(function(value) { // success console.log("Value: "+JSON.stringify(value)) }).catch(function(reason) { // failure console.log("Error: "+reason) }); } }
Concurrently executes several promises and returns another promise that is fulfilled when the first of those promises is fulfilled.
import Felgo import QtQuick App { Component.onCompleted: { var p1 = HttpRequest .get("http://httpbin.org/delay/3") .then(function(resp) { return 3; }); var p2 = HttpRequest .get("http://httpbin.org/delay/1") .then(function(resp) { return 1; }); var p3 = Promise.race([p1, p2]); p3.then(function(value) { console.log(value); // 1 or 3 }); } }
Returns a rejected promise.
import Felgo import QtQuick App { Component.onCompleted: { var p = Promise.reject("error"); p.catch(function(reason) { console.log(reason); // "error" }); } }
Returns a resolved promise.
import Felgo import QtQuick App { Component.onCompleted: { var p = Promise.resolve(5); p.then(function(value) { console.log(value); // 5 }); } }