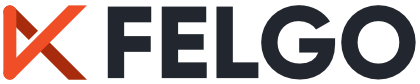
The Amplitude item provides functions for getting insights into your app's usage. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.13.0 |
Inherits: |
The Amplitude plugin provides functions for getting insights into your app's usage for iOS & Android.
[since Felgo 3.9.0] adSupportBlock : script |
Use this property to set the used device ID based on the advertising ID (Advertising Identifier (IDFA) on iOS) instead of using a unique, auto-generated UUID.
The property needs to be used in combination with useAdvertisingIdForDeviceId. Set this property to a QML script that returns the desired device/advertising ID.
The Amplitude item calls this QML script then directly from the native SDK's adSupportBlock.
You can use the Native Code Components to get the iOS device's advertising ID from ASIdentifierManager
.
Example implementation:
Amplitude { id: amplitude apiKey: "<your API key>" // enable to get IDFA for device ID, instead of device vendor ID (IDFV) useAdvertisingIdForDeviceId: true // called when the IDFA is requested adSupportBlock: { // Objective C: // auto idfa = [[[ASIdentifierManager sharedManager] advertisingIdentifier] UUIDString]; var ASIdentifierManager = NativeObjectUtils.getClass("ASIdentifierManager") var manager = ASIdentifierManager.getProperty("sharedManager") var idfa = manager.getProperty("advertisingIdentifier") var idfaUUID = idfa.getProperty("UUIDString") console.debug("IDFA is:", idfaUUID) return idfaUUID } }
Note: Calling ASIdentifierManager
requires linking the AdSupport
framework. For more information, see the plugin integration
guide.
Note: The adSupportBlock is only used on iOS.
You can read more about device IDs at the official Amplitude documentation.
This property was introduced in Felgo 3.9.0.
See also useAdvertisingIdForDeviceId, setDeviceId(), and getDeviceId().
[since Felgo 2.13.0] apiKey : string |
Your Amplitude API key for the set up project. Be sure to set this before calling any tracking functions. If you want separate tracking for iOS and Android, use the following example code:
import Felgo Amplitude { // a different API key is used for Android and iOS apiKey: Qt.platform.os === "ios" ? "<ios-api-key>" : "<android-api-key>" }
This property was introduced in Felgo 2.13.0.
[since Felgo 2.16.0] useAdvertisingIdForDeviceId : bool |
Use this property to set the device ID based on the advertising ID (Google Advertising ID on Android, Advertising Identifier (IDFA) on iOS), instead of using a unique, auto-generated UUID.
Note: The property must be set during the intialization of the plugin. Later changes won't have any effect.
The default value for this property is false
.
You can read more about device IDs at the official Amplitude documentation.
Note: Since iOS 14 changes in early spring of 2021, using the IDFA now requires authorization for Ad Tracking Transparency from the user. The plugin will automatically request this authorization, if this property
is set to true
.
This requires the following entry in your ios/Project-Info.plist
:
<key>NSUserTrackingUsageDescription</key> <string>This identifier will be used to deliver personalized ads to you.</string>
Note: The plugin does not include any AdSupport logic to get the actual IDFA
then, it just includes the logic to ask for permission to use it. You need to set the IDFA
in QML with the
help of the adSupportBlock.
This property was introduced in Felgo 2.16.0.
See also adSupportBlock, setDeviceId(), and getDeviceId().
[since Felgo 2.16.0] userId : string |
Use this property to set a custom user ID for Amplitude events. This allows merging events from the same user on multiple devices. To do so, keep track of your user's identity (for example using a username/password login) and then set a custom user ID based on the username that will be the same on each device where the same user logs in. Be careful to generate unique IDs for each user, so that events don't get merged falsely.
When a custom ID was set but the user logs out and you want to send IDs from an anonymous user again, set user ID to null.
The default value of this property is null
, which indicates that an auto-generated, unique user ID based on the user's device is used.
User IDs are generally not persisted between app starts, so if your app persists user sessions, be sure to set the user ID for the Amplitude item again on app start.
Example code for login and logout:
import Felgo Amplitude { id: amplitude apiKey: "<your-api-key>" function login(username) { //associate all future events from this device with this user ID amplitude.userId = userNameToUniqueId(username) } function logout() { //send future events from an anonymous user with automatic user ID amplitude.userId = null } //set user ID again on app start if already logged in Component.onCompleted: login(storedUserName) }
The Amplitude dashboard will group events coming from the same userId
, or from the same deviceId
if no userId
is set, like you can see in this image:
You can read more about user IDs at the official Amplitude documentation.
This property was introduced in Felgo 2.16.0.
[since Felgo 2.16.0] userProperties : var |
Additional properties for your user that will be shown in the amplitude dashboard. The properties belong to your user and will be shown for each session and event of the user. They persist between sessions and events. Changing the user properties even changes them for previously sent events of the same user.
The userProperties
should be a JavaScript object containing key-value pairs of properties. Supported types of properties are Number, String and Array.
Example code setting user properties (note the extra set of parentheses around the properties, so they are not interpreted as a property binding code block):
import Felgo Amplitude { apiKey: "<your-api-key>" userProperties: ({ age: 17, weight: 110.3, name: "Gregor", achievements: [1, 2, 4, 8] }) }
The Amplitude dashboard will show your custom user properties in addition to the ones it gathers on its own:
You can read more about user properties at the official Amplitude documentation.
This property was introduced in Felgo 2.16.0.
|
Use this method to retrieve the current ID that Amplitude uses to uniquely identify the user's device. This ID can be changed using setDeviceId().
The device ID is not a regular QML property as the native Amplitude SDK provides no callbacks when it is initialized or updated.
You can read more about device IDs at the official Amplitude documentation.
This method was introduced in Felgo 2.16.0.
See also useAdvertisingIdForDeviceId and setDeviceId().
Log a single event with name event and a JSON-encoded keyValueMap. For example:
{ amplitude.logEvent("Display.GameScene", {"level": 16, "numberDied": 2} ); }
This method was introduced in Felgo 2.13.0.
Log a single event with name event and key with value. For example:
{ amplitude.logEvent("Tower.Built", "eventType", "Flamethrower"); }
This method was introduced in Felgo 2.13.0.
|
Log a single event with name event. For example:
{ amplitude.logEvent("Display.CreditsScene"); }
This method was introduced in Felgo 2.13.0.
|
Log a revenue event from your app. This allows you to keep track of your app's revenue earnings. The amplitude dashboard will then show detailed revenue information, for example a graph like this:
The parameter revenueData should be a JavaScript object with the necessary information about the revenue event. Following properties are supported in this object:
productId
(optional): A string containing the identifier for the product, like the Google Play store product ID.quantity
(required): A number containing the quantity of products purchased:price
(required): A number containing the price of the products purchased, and this can be negative.revenueType
(optional): A string containing the type of revenue (e.g. tax, refund, income).eventProperties
(optional): A JavaScript object with additional properties to include in the revenue event that will show up in the Amplitude console.This example shows how to log a revenue event:
{ amplitude.logRevenue({ productId: "p17", quantity: 3, price: 10, //earned revenue = 3 * 10 = 30 revenueType: "income", eventProperties: { extraProp1: 178, extraProp2: "any text" } }); }
You can read more about revenue events at the official Amplitude documentation.
Note: Revenue verification is currently not supported.
This method was introduced in Felgo 2.16.0.
Use this method to set a custom deviceId for Amplitude events.
This is only for advanced use, if your app assigns custom IDs to devices. In most cases it can be left unchanged, in which case the Amplitude SDK assigns a unique, auto-generated UUID to each different device.
The device ID is not a regular QML property as the native Amplitude SDK provides no callbacks when it is initialized or updated.
You can read more about device IDs at the official Amplitude documentation.
This method was introduced in Felgo 2.16.0.
See also useAdvertisingIdForDeviceId and getDeviceId().