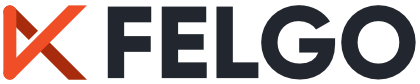
Felgo Apps is a QML module for developing feature-rich, cross-platform apps with the native look and feel of the underlying platform. It is part of the Felgo SDK. Felgo Apps components can be used to build cross-platform applications for Mobile, Desktop, Embedded and Web platforms.
QML stands for "Qt Markup Language" and is a declarative language designed to describe the user interface of a program: both what it looks like, and how it behaves. In QML, a user interface is specified as a tree of objects with properties. This tree is also often referred to as Scene Graph, because when the parent item moves all children will follow. JavaScript is used as a scripting language in QML. In this section we will cover the basics of QML and its advantages by an example. In addition QML components can be linked with C++ code, allowing you to implement more complex functionality and access third-party framework integrations.
As code speaks more than 1000 words, here is a QML example implementing the following:
How many lines of code would it take you to develop this application with the same functionality in another programming language? With QML, it is just about 30 lines of code:
import Felgo import QtQuick App { id: app property int clickCount: 0 // a property of type integer, to count our button clicks NavigationStack { // the NavigationStack displays the navigation bar and lets you push and pop pages AppPage { title: "QML Introduction" // the title of the active page is displayed in the navigation bar Rectangle { color: "#e0e2eb" width: parent.width // make this rectangle the same width as its parent, which is the page height: parent.height * slider.value // this property binding is automatically updated when the slider value changes } Column { anchors.centerIn: parent // center the column in its parent, which is the page AppButton { id: button text: "Click Me! " + app.clickCount // this property binding is automatically updated when the click count changes flat: false anchors.horizontalCenter: parent.horizontalCenter onClicked: { app.clickCount++ // increase the click count by 1 on every button click } } AppSlider { id: slider value: 0.2 } } //Column } //Page } //NavigationStack } //App
Felgo Apps makes it possible to write cross-platform apps that:
Felgo Apps includes components for developing cross-platform apps with a native look and feel to interfaces and user experience patterns.
In addition to Felgo Apps you most probably will also make use of components from the Qt Quick module provided by the Qt framework.
The current release of Felgo Apps is based on Qt 6 and QtQuick 2.