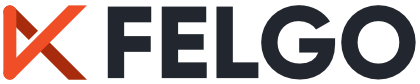
Lets your users sign in with their Apple account. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
The Apple sign in plugin lets your users authenticate with their Apple ID.
This is a logical item that handles Apple account sign in on iOS.
On unsupported platforms, method calls do nothing. The only supported platforms are iOS 13+.
Use the function signIn() to show the native sign in dialog.
When the sign in is successful, it emits the signal signInCompleted(). It also stores the user's ID, name and email. These properties are persisted in userId, userName and email.
On app start, this item checks if the Apple account is still authorized. If not, the properties userId, userName and email are cleared.
The following example code shows how to integrate AppleSignIn and access the current authorization state:
import QtQuick 2.0 import Felgo 4.0 App { NavigationStack { AppPage { title: "Apple Sign In" Column { anchors.centerIn: parent AppleSignIn { id: appleSignIn } // Note that you have to follow Apple's official interface guidelines for this button // We recommend to use the appleSignInButton component instead AppButton { anchors.horizontalCenter: parent.horizontalCenter enabled: appleSignIn.isAvailable text: "Sign in with Apple" onClicked: appleSignIn.signIn() } AppText { anchors.horizontalCenter: parent.horizontalCenter text: appleSignIn.isSignedIn ? "Signed in as: " + appleSignIn.userName : "Not signed in." } } } } }
Note: Using AppleSignIn by itself is for customized usage. Most apps can use the pre-configured AppleSignInButton. It confirms to Apple's Button Human Interface Guidelines.
[read-only] authorizationCode : string |
A short-lived token used by your app for proof of authorization when interacting with the app’s server counterpart.
[read-only] authorizedScopes : var |
The contact information the user authorized your app to access.
[read-only] email : string |
Contains the signed in user's e-mail address after a successful sign in.
email contains the Apple account e-mail. The user can choose to hide their actual e-mail address when signing in. In this case, it is an auto-generated relay e-mail address. You can still use it to send the user messages.
[read-only] identityToken : string |
A JSON Web Token (JWT) that securely communicates information about the user to your app.
[read-only] isAvailable : bool |
Contains true
if signIn() is supported on the current platform.
Supported platforms are only iOS 13+.
Contains false
on all other platforms.
isSignedIn : bool |
Contains true
while the user is authorized.
[read-only] userId : string |
Contains the signed in user's ID after a successful sign in.
[read-only] userName : string |
Contains the signed in user's full name after a successful sign in.
signInCompleted() |
Gets emitted on iOS after a successful sign in with signIn().
After this signal, the properties userId, userName, email, identityToken, authorizationCode and authorizedScopes contain their respective values.
Note: The corresponding handler is onSignInCompleted
.
See also signIn() and signInFailed().
signInFailed(string message) |
Gets emitted on iOS after an unsuccessful login with signIn().
The parameter message contains localized information why the sign in attempt failed.
Note: The corresponding handler is onSignInFailed
.
See also signIn() and signInCompleted().
signIn() |
Allows the user to sign in to your app with their Apple ID account.
This will show a native sign in dialog for the user to enter their Apple account credentials.
After a successful login, NativeUtils emits the signal signInCompleted(). The parameters contain the user's ID, name and email after a successful login. You can use these credentials to sign up or log in the user to your app backend.
The user usually stays logged in when they close and reopen the app. This item thus persists the user ID, name and email between app starts. It is however possible that the authorization is no longer active. In this case these values are discarded on startup.
If the user does not log in successfully, this item emits the signal signInFailed(). It provides additional information as parameters to the signal.
Note: This method is only supported on iOS 13+. It does nothing on all other platforms. You can check availability at runtime with isAvailable.
See also signInCompleted(), signInFailed(), and isAvailable.