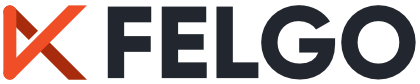
The basic item for any type of lists, with text, images, icons etc. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
The AppListItem is intended for use with models within an AppListView as well as custom lists e.g. with a Column. Examples for both will be given in this document.
iOS | Android |
---|---|
|
|
This is a visible representation of the most important content and layout properties of the AppListItem:
This is a very minimal example with just texts and listening to clicks on the item:
import Felgo import QtQuick App { NavigationStack { AppPage { title: "AppListItem" AppListItem { text: "Hi" detailText: "You look great!" onSelected: { console.debug("AppListItem selected") } } } } }
Here are 3 examples how to use an AppListItem with an AppListView, a Repeater and a completely custom list.
This example shows a very basic usage if AppListItem within an AppListView to display data in a list:
import Felgo import QtQuick App { id: app property var myModel: [ {label: "Item 1", info: "Some more info"}, {label: "Item 2", info: "Some more info"}, {label: "Item 3", info: "Some more info"}, {label: "Item 4", info: "Some more info"}, {label: "Item 5", info: "Some more info"}, ] NavigationStack { AppPage { title: "AppListView" AppListView { model: app.myModel delegate: AppListItem { text: modelData.label detailText: modelData.info } } } } }
This example uses a Repeater with a Column inside an AppFlickable. In certain use-cases, this offers more flexibility than using an AppListView:
import Felgo import QtQuick App { id: app property var myModel: [ {label: "Item 1", info: "Some more info"}, {label: "Item 2", info: "Some more info"}, {label: "Item 3", info: "Some more info"}, {label: "Item 4", info: "Some more info"}, {label: "Item 5", info: "Some more info"}, ] NavigationStack { AppPage { title: "Repeater" AppFlickable { anchors.fill: parent contentHeight: column.height Column { id: column width: parent.width Repeater { model: app.myModel delegate: AppListItem { text: modelData.label detailText: modelData.info } } } } } } }
This example simply puts custom list items in a column, without any model:
import Felgo import QtQuick App { id: app NavigationStack { AppPage { title: "Repeater" AppFlickable { anchors.fill: parent contentHeight: column.height Column { id: column width: parent.width AppListItem { text: "Some text" detailText: "And some detailText" } AppListItem { text: "Some other text" detailText: "And some totally different detailText" } } } } } }
This example uses a SortFilterProxyModel to sort a JsonListModel by its sections and then display the resulting list with visible sections:
import QtQuick import Felgo App { id: app property var jsonData: [ {id: 1, title: "Apple", type: "Fruit"}, {id: 2, title: "Ham", type: "Meat"}, {id: 3, title: "Bacon", type: "Meat"}, {id: 4, title: "Banana", type: "Fruit"}, {id: 5, title: "Strawberry", type: "Fruit"} ] // list model for json data JsonListModel { id: jsonModel source: jsonData keyField: "id" fields: ["id","title","type"] } SortFilterProxyModel { id: sortedModel // Note: when using JsonListModel, the sorters or filter might not be applied correctly when directly assigning sourceModel // use the Component.onCompleted handler instead to initialize SortFilterProxyModel Component.onCompleted: sourceModel = jsonModel sorters: LocaleAwareSorter { id: typeSorter; roleName: "type"; ascendingOrder: true } } NavigationStack { AppPage { title: "Sections" AppListView { model: sortedModel section.property: "type" section.delegate: SimpleSection { } delegate: AppListItem { text: model.title detailText: model.type } } } } }
You can also find a longer list of examples for customized list items here: Customize AppListItems for custom Lists.
This is a simple example where both leftItem and rightItem are replaced with custom QML items:
import Felgo import QtQuick App { id: app NavigationStack { AppPage { title: "Repeater" AppListItem { text: "General" leftItem: Rectangle { color: "grey" radius: dp(5) width: dp(26) height: width anchors.verticalCenter: parent.verticalCenter AppIcon { iconType: IconType.cog anchors.centerIn: parent color: "white" } } rightItem: Rectangle { color: "red" radius: width/2 width: dp(22) height: width anchors.verticalCenter: parent.verticalCenter AppText { anchors.centerIn: parent color: "white" text: "1" } } } } } }
Find more examples for frequently asked development questions and important concepts in the following guides:
active : bool |
The property indicates if a list item is currently active. This can be used to apply different colors.
The default value is false
See also activeBackgroundColor, activeTextColor, and activeDetailTextColor.
activeBackgroundColor : color |
The background color of the list item if it is active.
The default value is Theme.listItem.activeBackgroundColor
.
See also backgroundColor and selectedBackgroundColor.
activeDetailTextColor : color |
The color of the secondary text, if the list item is active.
The default value is Theme.listItem.activeDetailTextColor
See also detailText.
activeTextColor : color |
The color of the main text, if the list item is active.
The default value is Theme.listItem.tintColor
See also text.
backgroundColor : color |
The background color of the list item.
The default value is Theme.listItem.backgroundColor
.
See also selectedBackgroundColor and activeBackgroundColor.
bottomPadding : real |
The vertical padding added below the detailTextItem. Note that this is not used if the list item height would be lower than
dp(Theme.listItem.minimumHeight)
.
The default value is dp(10)
on iOS and dp(12)
on all other platforms.
detailText : string |
The secondary text displayed in the list item.
The default secondary text item can be replaced if you provide a detailTextItem.
See also detailTextColor, selectedDetailTextColor, activeDetailTextColor, detailTextFontSize, detailTextMaximumLineCount, textVerticalSpacing, and detailTextItem.
detailTextColor : color |
The color of the secondary text, in default state.
The default value is Theme.listItem.detailTextColor
See also detailText.
detailTextFontSize : real |
The size of the secondary text.
The default value is sp(Theme.listItem.fontSizeDetailText)
See also detailText.
detailTextItem : Item |
Use this property to put a custom QML item in the detailText slot of the list item. This will overwrite the default text item created if the detailText property is set.
See also detailText.
detailTextMaximumLineCount : int |
The maximum line count of the secondary text, before elide is applied.
The default value is 1000
See also detailText.
disclosureColor : color |
The color of the disclosure item.
The default value is Theme.listItem.disclosureColor
See also showDisclosure.
disclosureItem : Item |
Use this property to put a custom QML item in the disclosure slot of the list item. This will overwrite the default icon item created if the showDisclosure property is set.
See also showDisclosure.
dividerColor : color |
The color of the bottom divider line.
The default valie is Theme.listItem.dividerColor
See also showDivider.
dividerHeight : real |
The height of the bottom divider line.
The default valie is px(Theme.listItem.dividerHeight)
See also showDivider.
dividerLeftSpacing : real |
The left spacing of the bottom divider line.
By default, the divider will align with the left edge of the text in the list item on iOS. On all other platforms it will cover the full width of the list item.
If the list item is used within an AppListView, the last item in the list will also show a full-width divider. If the AppListView uses sections, the last list item of every section will show a full-width divider.
You can also set lastInSection to true
manually to use a full-width divider.
See also showDivider and lastInSection.
image : string |
Provide the url of an image to display it in the row.
AppListItem { text: "Item with Image" image: Qt.resolvedUrl("../assets/someImage.png") }
The default image item can be replaced if you provide a leftItem.
See also imageSize, imageRadius, and imageFillMode.
imageFillMode : real |
The fillMode of the image used in the list item.
The default value is Image.PreserveAspectCrop
imageRadius : real |
The radius of the image used in the list item.
By default, the image is a circle, thus the radius is imageSize/2
imageSize : int |
The size of the image used in the list item.
The default sizes are:
dp(26)
dp(46)
lastInSection : bool |
Setting this property on iOS will cause the bottom divider to be drawn as full-width. On other platform this property will have no visual effect.
If the list item is used within an AppListView, this property will be determined automatically. Else the default value is false
leftItem : Item |
leftPadding : real |
The horizontal padding in on the on the left side of the list item.
The default value is dp(Theme.contentPadding)
mouseArea : alias |
Alias to the internal RippleMouseArea. This can be used e.g. to disable the internal RippleMouseArea without disabling the whole list item, for example to put a switch there:
AppListItem { text: "This is a switch" showDisclosure: false mouseArea.enabled: false rightItem: AppSwitch { anchors.verticalCenter: parent.verticalCenter checked: true } }
muted : bool |
Set this property to visually mute/disable the item, causing all content to be translucent using mutedOpacity.
Setting this property to true
will also set enabled of the list item to false
, so it will not react to any clicks.
If you just want to disable clicks on the list item without any visual effect, set enabled of the AppListItem to false
. If you have controls inside the AppListItem that should stay enabled, while the rest of the list item should be disabled, you can use mouseArea.enabled: false
to only disable the internal RippleMouseArea.
The default value is false
See also mutedOpacity and mouseArea.
mutedOpacity : real |
rightItem : Item |
rightPadding : real |
The horizontal padding in on the on the right side of the list item.
The default value is dp(Theme.contentPadding)
rightText : string |
The text displayed on the right side of the list item. If a disclosure is shown, it will align with it.
The default right text item can be replaced if you provide a rightItem.
See also rightTextColor, rightTextFontSize, rightTextMaxWidth, and rightItem.
rightTextColor : color |
The color of the right text, in default state.
The default value is Theme.listItem.rightTextColor
See also rightText.
rightTextFontSize : real |
The size of the right text.
The default value is sp(Theme.listItem.fontSizeRightText)
See also rightText.
rightTextMaxWidth : real |
The maximum width of the right text, before elide is applied.
This is very important to set if the right text can be very long, else the text and detailText may be pushed out of the list item.
The default value is 0
which means there is no limit on the max width. Set a value >1 here to apply a limit.
See also rightText.
selectedBackgroundColor : color |
The background color of the list item if selected (while the internal RippleMouseArea is pressed).
The default value is Theme.listItem.selectedBackgroundColor
.
See also backgroundColor and activeBackgroundColor.
selectedDetailTextColor : color |
The color of the secondary text, when selected.
The default value is Theme.listItem.selectedDetailTextColor
See also detailText.
selectedTextColor : color |
The color of the main text, when selected.
The default value is Theme.listItem.selectedTextColor
See also text.
showDisclosure : bool |
Set this to false
to hide the disclosure icon of the row, which is displayed by default on iOS.
The default value is Theme.listItem.showDisclosure
See also disclosureColor and disclosureItem.
showDivider : bool |
Set this to false
to hide the bottom divider line of the row, which is displayed by default on all platforms.
The default value is Theme.listItem.showDivider
See also dividerColor, dividerHeight, and dividerLeftSpacing.
text : string |
The main text displayed in the list item.
The default main text item can be replaced if you provide a textItem.
See also textColor, selectedTextColor, activeTextColor, textFontSize, textMaximumLineCount, textVerticalSpacing, and detailTextItem.
textColor : color |
The color of the main text, in default state.
The default value is Theme.listItem.textColor
See also text.
textFontSize : real |
textItem : Item |
[read-only] textItemAvailableWidth : real |
This is a readonly helper property, that provides the available width for the textItem and detailTextItem. It can be used for custom QML items placed in those slots.
AppListItem { id: listItem showDisclosure: false mouseArea.enabled: false topPadding: 0 bottomPadding: 0 leftItem: AppIcon { iconType: IconType.moono width: sp(26) height: width anchors.verticalCenter: parent.verticalCenter } textItem: AppSlider { height: dp(45) width: listItem.textItemAvailableWidth value: 0.3 } rightItem: AppIcon { iconType: IconType.suno width: sp(26) height: width anchors.verticalCenter: parent.verticalCenter } }
The value will be calculated with respect to the leftItem, rightItem, disclosureItem width values and any paddings/spacings that are applied.
textMaximumLineCount : int |
The maximum line count of the main text, before elide is applied.
The default value is 1000
See also text.
textVerticalSpacing : real |
The vertical space between the textItem and the detailTextItem.
The default value is dp(Theme.listItem.textVerticalSpacing)
See also text and detailText.
topPadding : real |
The vertical padding added above the textItem. Note that this is not used if the list item height would be lower than Theme.listItem.minimumHeight.
The default value is dp(10)
on iOS and dp(12)
on all other platforms.
selected(int index) |
Emitted when this list item has been clicked. If the list item is used within a Repeater or AppListView, the index
of the item in the model will be passed as well.
Note: The corresponding handler is onSelected
.