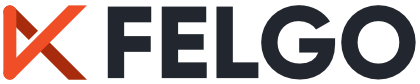
Let your users authorize with external OAuth 2.0 providers. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 4.2.0 |
Inherits: |
The OAuth 2.0 Plugin lets your users authorize with external OAuth 2.0 providers.
OAuth 2.0 is the industry-standard protocol for authorization. Read more at https://oauth.net/2/.
This item lets your users authorize with an OAuth 2.0-enabled API.
The following example sets up an OAuth2Client client to authorize a user with a GitHub app.
When the user clicks on "Login", it opens the GitHub login page in a system browser.
Afterwards, it provides the OAuth2Client::accessToken for REST API calls.
import QtQuick import Felgo App { id: app readonly property url userUrl: "https://api.github.com/user" property var userData: null OAuth2Client { id: oauth // adapt to your own GitHub app authUrl: "https://github.com/login/oauth/authorize" tokenUrl: "https://github.com/login/oauth/access_token" callbackUrl: "felgooauthtest://callback" clientId: "<your client ID>" clientSecret: "<your client secret>" // GitHub does not support PKCE pkceType: OAuth2Client.None // show consent dialog on every login promptType: OAuth2Client.Consent onAuthenticationError: message => { console.log("OAuth2: authentication error:", message) errorText.text = "Auth error: " + message } onIsAuthenticatedChanged: { if(isAuthenticated) { // we are now authenticated and can use the accessToken errorText.text = "" getUser() } } } ///////////////////////////// NavigationStack { FlickablePage { title: "GitHub OAuth Test" flickable.contentHeight: content.height Column { id: content width: parent.width AppText { width: parent.width wrapMode: Text.WrapAtWordBoundaryOrAnywhere text: "Access Token: " + oauth.accessToken } AppText { width: parent.width wrapMode: Text.WrapAtWordBoundaryOrAnywhere text: "Refresh Token: " + oauth.refreshToken } Flow { width: parent.width AppButton { text: "Login" enabled: !oauth.isAuthenticated onClicked: oauth.authenticate() } AppButton { text: "Refresh tokens" enabled: oauth.isAuthenticated && !!oauth.refreshToken onClicked: oauth.requestRefreshedTokens() } AppButton { text: "Logout" enabled: oauth.isAuthenticated onClicked: { oauth.logout() userData = null } } } AppText { id: errorText text: "" width: parent.width wrapMode: Text.WrapAtWordBoundaryOrAnywhere } AppText { visible: !!userData text: "Logged in as: " + userData?.login width: parent.width wrapMode: Text.WrapAtWordBoundaryOrAnywhere } AppImage { visible: !!userData source: userData?.avatar_url ?? "" width: parent.width fillMode: Image.PreserveAspectFit } } } } function getUser() { // Get GitHub user data: https://docs.github.com/en/rest/users HttpRequest .get(userUrl) .set("Accept", "application/vnd.github+json") // add access bearer token as HTTP authentication: .set("Authorization", "Bearer " + oauth.accessToken) .set("X-GitHub-Api-Version", "2022-11-28") .then(res => { userData = JSON.parse(res.text) console.log("User data:", JSON.stringify(userData, null, " ")) }) .catch(err => { console.log("Could not get user:", err.status, err.code, err.message, err.response.text) }) } }
[read-only] accessToken : string |
Read-only property containing the currently active access token. It contains a valid token as long as isAuthenticated is true.
For more information about access tokens, see https://oauth.net/2/access-tokens/.
See also refreshToken and accessTokenExpirationDate.
[read-only] accessTokenExpirationDate : date |
Read-only property containing the accessToken's expiration date.
See also accessToken.
[read-only] authStatus : int |
Read-only property containing current authentication status.
It can contain one of the following values:
OAuth2Client.NotAuthenticated
: No credentials have been retrieved.OAuth2Client.Granted
: A valid accessToken is available. Only in this case, isAuthenticated
contains true
.
OAuth2Client.RefreshingTokens
: The item is currently requesting new credentials via the refreshToken.
See also isAuthenticated.
[required] authUrl : url |
Set this property to the authorization endpoint URL.
A call to authenticate() opens this URL in a system browser. It adds the relevant parameters as specified by OAuth 2.0 automatically. Use the properties of this type to configure them.
This property is always required.
See also pkceType, promptType, scopes, tokenUrl, and authenticate().
[required] callbackUrl : url |
Set this property to your app's callback URL.
The flow uses this URL to return to the app after the user authenticated themselves.
Use any custom URL scheme with this property. Register your app to open via this URL scheme, via AndroidManifest.xml
on Android and Project-Info.plist
on iOS.
This property is always required.
[required] clientId : string |
Your apps client ID.
You usually obtain this ID from the backend. It identifies your application with the API.
This property is always required.
See also clientSecret.
clientIdentifier : string |
A unique identifier for this OAuth2Client instance.
You can use this property if you have multiple different OAuth2Clients in your application. The item persists its internal state via this identifier.
You can also omit setting this property. The item then uses a hash of the authUrl and clientId as a default value.
clientSecret : string |
Your apps client secret.
You usually obtain this secret from the backend. It authenticates your application with the API.
This property is not always required. It is added to the token endpoint call only when it is set.
See also clientId.
[read-only] grantedScopes : var |
Read-only property containing the scopes granted by the backend.
This is usually a the same or a subset of the requested scopes.
See also scopes and authenticate().
httpAuthenticationHeader : string |
Optionally sets the authentication header for the token retrieval.
Some APIs require HTTP authentication.
The default value of this property is an empty string. In this case, it uses no authentication header.
See also scopeDelimiter, pkceType, promptType, and authenticate().
httpContentType : string |
Optionally sets the content type for the token retrieval POST request.
The token endpoint may accept different content types.
This item supports the following types:
"application/json"
: The default value of this property. Sends data in JSON format."application/x-www-form-urlencoded
: Sends data in URL-encoded format.See also tokenUrl and authenticate().
[read-only] idToken : string |
Read-only property containing the OpenID Connect ID token.
It contains a value if the field id_token
was set in the token response.
For more information about ID tokens, see https://openid.net/specs/openid-connect-core-1_0.html#IDToken.
See also accessToken and idTokenData.
[read-only] idTokenData : var |
Read-only property containing the OpenID Connect ID token payload.
It contains the idToken's payload, parsed as a JSON object.
Note: This property only contains a value if the idToken was validated and decoded successfully. It supports the validation algorithms "SH256"
(SHA-256) and "none"
(no validation).
See also accessToken and idToken.
[read-only] isAuthenticated : bool |
Read-only property containing true
when the user is successfully authenticated. As long as it is true, accessToken contains a valid token.
This is true
whenver authStatus is OAuth2Client.Granted
.
See also authStatus.
pkceLength : int |
pkceType : int |
Sets the desired PKCE method to use during authorization.
Set it to one of the following values:
OAuth2Client.None
: The default value of this property. No PKCE challenge is used during authorization.OAuth2Client.Plain
: A plain PKCE challenge is used during authorization.OAuth2Client.SHA256
: A SHA-256 hash of the PKCE challenge is used during authorization.Note: Not all backends may support all PKCE types.
Read more about this functionality in the section PKCE Extension.
See also pkceLength, promptType, scopes, and authenticate().
promptType : int |
Optionally sets which user prompt to display during authentication.
Set it to one of the following values:
OAuth2Client.NotSet
: The default value of this property. Requests no specific prompt type.OAuth2Client.None
: Does request no user prompt. If already authenticated, the login may happen without user input.OAuth2Client.Consent
: Re-requests the user's consent to authorize, if already given.OAuth2Client.Login
: Re-requests the user to login, if already logged in.OAuth2Client.SelectAccount
: Requests the user to re-select an account.OAuth2Client.Create
: Requests the user to create a new account.Note: Not all backends may support all prompt types.
See also pkceType, scopes, and authenticate().
[read-only] refreshToken : string |
Read-only property containing the currently active refresh token.
A refresh token is generally longer-lived than an access token. The item uses it to request a new access token.
This property is only set if the token endpoint returns a refresh token in its response.
It is usually not necessary to use this token directly. You can call requestRefreshedTokens() to request a new access token via the refresh token.
For more information about refresh tokens, see https://oauth.net/2/grant-types/refresh-token/.
See also accessToken, refreshTokenExpirationDate, and requestRefreshedTokens().
[read-only] refreshTokenExpirationDate : date |
Read-only property containing the refreshToken's expiration date.
See also refreshToken and requestRefreshedTokens().
refreshTokensAutomatically : bool |
If set to true
, the item will automatically request refreshed tokens, if the current access token is expired.
Note: It only checks the accessTokenExpirationDate on startup, after the plugin is loaded. You can also request a refreshed token with a manual call to requestRefreshedTokens(), independent of this property's value.
The default value of this property is true
.
See also accessToken, refreshToken, and requestRefreshedTokens().
scopeDelimiter : string |
scopes : var |
Optionally sets the authorization scopes to request.
Set it to an array of strings, eg. scopes: ["users.read", "tweet.read"]
Note: The item sends the scopes as parameter via the authUrl. It joins them via the scopeDelimiter.
See also scopeDelimiter, pkceType, promptType, and authenticate().
[required] tokenUrl : url |
Set this property to the token endpoint URL.
After the user authorization via the authUrl is done, an access token is obtained via a REST call to this endpoint.
This property is always required.
See also authUrl, accessToken, and authenticate().
authenticationError(string message) |
The item emits this signal when there is an error during the authentication flow.
The parameter message contains information about the error.
Note: The corresponding handler is onAuthenticationError
.
See also authenticate().
authenticate() |
Starts the authentication flow.
If the authentication is successful, isAuthenticated will contain true and accessToken will contain a valid token.
Otherwise, it emits the authenticationError() signal.
See also accessToken, isAuthenticated, and authenticationError().
logout() |
Logs out the current user.
This deletes the current accessToken and refreshToken. It sets authStatus to OAuth2Client.NotAuthenticated
.
Note: It is possible that a subsequent call to authenticate() logs the same user in again automatically. You can use the promptType property to request manual login or consent again.
See also promptType and authenticate().
requestRefreshedTokens() |
Requests refreshed tokens from the token endpoint.
This method only has an effect if refreshToken contains a valid value.
Read more about this functionality in the section Token Refresh.
See also accessToken, refreshToken, and refreshTokensAutomatically.