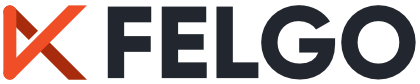
Embedded video player based on the YouTube Iframe-Player API. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.18.1 |
Inherits: |
This control allows to embed a YouTube player in your app. The component internally relies on a QML WebView to embed the player via HTML. The YouTubeWebPlayer component offers a QML API to configure and control the YouTube Iframe-Player.
Note: Qt does not support the QML WebView for the default minGW compiler on Windows. To use the Qt WebView module on Windows, you can build your app with the MSVC compiler.
For detailed information about the player capabilities, see the YouTube Iframe-Player API. On different target platforms or devices, autoplay may not be properly supported for embedded YouTube videos. This is due to browser restrictions with Qt WebView. In addition, a manual call to the play method only works after playback was previously started by a user action.
The YouTube Player Demo App, which is part of the Felgo SDK, is a YouTube channel browser to list and watch YouTube videos. It allows to watch videos and browse the playlists of the Felgo YouTube Channel.
Have a look at this demo to see how to integrate the Qt WebView module and use the YouTubeWebPlayer to play videos. The demo also shows how to load content from the YouTube Data API via http requests.
To use a WebView in your apps, it is required to link the Qt WebView module in your
*.pro
configuration:
QT += webview
You can now include and initialize the WebView in your main.cpp
:
// include WebView #include <QtWebView> // ... int main(int argc, char *argv[]) { // ... felgo.setMainQmlFileName(QStringLiteral("qml/YouTubePlayerMain.qml")); // ... // initialize WebView QtWebView::initialize(); engine.load(QUrl(felgo.mainQmlFileName())); return app.exec(); }
Note: Qt does not support the QML WebView for the default minGW compiler on Windows. To use the Qt WebView module on Windows, you can build your app with the MSVC compiler.
It is sufficient to configure the videoId property of the player.
import Felgo App { NavigationStack { AppPage { title: "YouTube Player" YouTubeWebPlayer { videoId: "KQgqTYCfJjM" } } } }
The following example uses the YouTubeWebPlayer configuration properties to create a custom player controlled from QML:
import Felgo import QtQuick App { NavigationStack { AppPage { title: "YouTube Player" YouTubeWebPlayer { id: player videoId: "KQgqTYCfJjM" playerVars: { "controls": 0, // hide player controls "showinfo": 0 // hide video title } enabled: false // no interaction with player possible } // controls Rectangle { anchors.top: player.bottom width: parent.width height: controls.height color: Theme.backgroundColor Column { id: controls anchors.horizontalCenter: parent.horizontalCenter AppButton { text: "Play" onClicked: player.play() } AppButton { text: "Pause" onClicked: player.pause() } AppButton { text: "Stop" onClicked: player.stop() } } } } } }
The following example uses the customHTML, playerErrorScript and playerStateChangeScript properties to show a customized HTML error message.
import Felgo App { NavigationStack { AppPage { title: "YouTube Player" YouTubeWebPlayer { id: player videoId: "*invalid!" // hide player iframe and display message on error playerErrorScript: " player.getIframe().style.display='none'; document.getElementById('videoIdError').style.display='block'; " // show player iframe and hide message when new video has loaded playerStateChangeScript: "if(event.data == YT.PlayerState.PLAYING) { document.getElementById('videoIdError').style.display='none'; player.getIframe().style.display='block'; }" // add custom HTML for showing the error customHTML: '<style> .videoError { background-color: black; color: white; width: 100%; height: 100%; position: absolute; display: none; } </style > <div id="videoIdError" class="videoError"> This video is not available. </div>' } AppButton { text: "Change Video" anchors.top: player.bottom anchors.horizontalCenter: parent.horizontalCenter onClicked: { if(player.videoId == "KQgqTYCfJjM") player.videoId = "*invalid!" else player.videoId = "KQgqTYCfJjM" } } } } }
autoplay : bool |
Configures whether the video should play automatically when loaded. Defaults to false
. The autoplay configuration is part of the playerVars, which are
passed to the embedded YouTube player.
On different target platforms or devices, autoplay may not be properly supported for embedded YouTube videos. This is due to browser restrictions with Qt WebView.
See also playerVars.
customHTML : string |
Allows to extend the WebView with additional HTML code. The content of this property is directly inserted at the end of the player HTML's body
tag. This allows to e.g. react to player errors by adding a playerErrorScript to show error messages you provided as customHTML.
See also playerReadyScript, playerStateChangeScript, playerPlaybackQualityChangeScript, playerPlaybackRateChangeScript, playerErrorScript, and customHTML.
loading : bool |
Holds true
if the WebView is currently in the process of loading new content, false
otherwise. Reloads happen whenever the HTML
for the WebView changes, e.g. when setting customHTML or player event scripts.
When the initial HTML load or a reload is finished, the loadingFinished signal fires.
See also loadingFinished and playerIsReady.
playerApiChangeScript : string |
Allows to extend the WebView with custom JavaScript to handle the onApiChange web player event. This can be useful when customizing the player with additional HTML elements and features. To add custom HTML items to the view, use the customHTML property.
See also playerReadyScript, playerStateChangeScript, playerPlaybackQualityChangeScript, playerPlaybackRateChangeScript, playerErrorScript, and customHTML.
playerErrorScript : string |
Allows to extend the WebView with custom JavaScript to handle the onError web player event. This can be useful when customizing the player with additional HTML elements and features. To add custom HTML items to the view, use the customHTML property.
See also playerReadyScript, playerStateChangeScript, playerPlaybackQualityChangeScript, playerPlaybackRateChangeScript, playerApiChangeScript, and customHTML.
[since Felgo 4.0.0] playerIsReady : bool |
Holds true
as soon as the YouTube player within the WebView is initialized and ready.
This property was introduced in Felgo 4.0.0.
See also loadingFinished and playerIsReady.
playerPlaybackQualityChangeScript : string |
Allows to extend the WebView with custom JavaScript to handle the onPlaybackQualityChange web player event. This can be useful when customizing the player with additional HTML elements and features. To add custom HTML items to the view, use the customHTML property.
See also playerReadyScript, playerStateChangeScript, playerPlaybackRateChangeScript, playerErrorScript, playerApiChangeScript, and customHTML.
playerPlaybackRateChangeScript : string |
Allows to extend the WebView with custom JavaScript to handle the onPlaybackRateChange web player event. This can be useful when customizing the player with additional HTML elements and features. To add custom HTML items to the view, use the customHTML property.
See also playerReadyScript, playerStateChangeScript, playerPlaybackQualityChangeScript, playerErrorScript, playerApiChangeScript, and customHTML.
playerReadyScript : string |
Allows to extend the WebView with custom JavaScript to handle the onReady web player event. This can be useful when customizing the player with additional HTML elements and features. To add custom HTML items to the view, use the customHTML property.
See also playerStateChangeScript, playerPlaybackQualityChangeScript, playerPlaybackRateChangeScript, playerErrorScript, playerApiChangeScript, and customHTML.
playerStateChangeScript : string |
Allows to extend the WebView with custom JavaScript to handle the onStateChange web player event. This can be useful when customizing the player with additional HTML elements and features. To add custom HTML items to the view, use the customHTML property.
See also playerReadyScript, playerPlaybackQualityChangeScript, playerPlaybackRateChangeScript, playerErrorScript, playerApiChangeScript, and customHTML.
playerVars : var |
A JSON object to describe player parameters, which you can use to configure the player. See the YouTube Player-Parameter docs for a list of all available settings.
For convenience, the autoplay
setting is available as an own property autoplay. Any configuration of autoplay
within the playerVars will be
overwritten.
See also autoplay.
videoId : string |
The ID that identifies the YouTube video, which the player will load.
loadingFinished() |
Fires when the initial HTML load or a reload is finished.
Note: This does not mean that the YouTube player and video within the video are initialized and loaded already.
Note: The corresponding handler is onLoadingFinished
.
See also loading and playerReady.
|
Fires when the YouTube player within the WebView is initialized and ready.
Note: The corresponding handler is onPlayerReady
.
This signal was introduced in Felgo 4.0.0.
See also playerIsReady.
Executes the loadVideoById function for the internal YouTube Iframe-Player.
The first parameter specifies the videoId
of the video to load. Setting the autoplay
parameter to true
will also start the video after loading it. The options
parameter
can be used to pass a JSON object of configuration options, as supported by the Iframe-Player's loadVideoById function.
void pause() |
Executes the pauseVideo function for the internal YouTube Iframe-Player.
void play() |
void runJavaScript(string code, function callback) |
Executes custom JavaScript code for the QML WebView which holds the YouTube Iframe-Player. This function is a wrapper for WebView::runJavaScript().
Your own scripts also have access to the YouTube Iframe-Player object using the player
variable. For example, you could pause the video using:
runJavaScript("player.pauseVideo()")
However, it is more convenient to directly use ready-made functions like pause for such features.
See also runPlayerScript.
Allows to run functions of YouTube Iframe-Player API from QML.
The first parameter specifies the targetFunction
to run for the player. As second parameter, additional arguments
can be passed as single value or array. You can then retrieve the return value of
the call by providing an optional callback
function.
For example, you can get the duration of the video using:
runPlayerScript("getDuration", function(duration){ console.log("Video Duration: "+duration) })
Or control the volume of the player with:
runPlayerScript("setVolume", 20)
See also runJavaScript.
Executes the seekTo function for the internal YouTube Iframe-Player.
The seconds
parameter specifies the target time the player should jump to. The allowSeekAhead
parameter defines, whether the player sends a new request to the server, in case the
seconds
parameter specifies a time that is not within the currently buffered video data.
void stop() |
Executes the stopVideo function for the internal YouTube Iframe-Player.