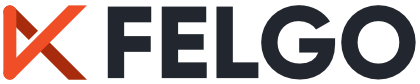
The QBluetoothUuid class generates a UUID for each Bluetooth service. More...
Header: | #include <QBluetoothUuid> |
qmake: | QT += bluetooth |
Since: | Qt 5.2 |
Inherits: | QUuid |
enum class | CharacteristicType { AerobicHeartRateLowerLimit, AerobicHeartRateUpperLimit, AerobicThreshold, Age, AnaerobicHeartRateLowerLimit, …, WindChill } |
enum class | DescriptorType { CharacteristicExtendedProperties, CharacteristicUserDescription, ClientCharacteristicConfiguration, ServerCharacteristicConfiguration, CharacteristicPresentationFormat, …, UnknownDescriptorType } |
enum class | ProtocolUuid { Sdp, Udp, Rfcomm, Tcp, TcsBin, …, L2cap } |
enum class | ServiceClassUuid { ServiceDiscoveryServer, BrowseGroupDescriptor, PublicBrowseGroup, SerialPort, LANAccessUsingPPP, …, ContinuousGlucoseMonitoring } |
QBluetoothUuid(const QUuid &uuid) | |
QBluetoothUuid(const QString &uuid) | |
QBluetoothUuid(quint128 uuid) | |
QBluetoothUuid(quint32 uuid) | |
QBluetoothUuid(quint16 uuid) | |
QBluetoothUuid(QBluetoothUuid::DescriptorType uuid) | |
QBluetoothUuid(QBluetoothUuid::CharacteristicType uuid) | |
QBluetoothUuid(QBluetoothUuid::ServiceClassUuid uuid) | |
QBluetoothUuid(QBluetoothUuid::ProtocolUuid uuid) | |
QBluetoothUuid() | |
int | minimumSize() const |
quint16 | toUInt16(bool *ok = nullptr) const |
quint32 | toUInt32(bool *ok = nullptr) const |
quint128 | toUInt128() const |
QString | characteristicToString(QBluetoothUuid::CharacteristicType uuid) |
QString | descriptorToString(QBluetoothUuid::DescriptorType uuid) |
QString | protocolToString(QBluetoothUuid::ProtocolUuid uuid) |
QString | serviceClassToString(QBluetoothUuid::ServiceClassUuid uuid) |
bool | operator!=(const QBluetoothUuid &a, const QBluetoothUuid &b) |
bool | operator==(const QBluetoothUuid &a, const QBluetoothUuid &b) |
[since 5.4]
enum class QBluetoothUuid::CharacteristicTypeThis enum is a convienience type for Bluetooth low energy service characteristics class UUIDs. Values of this type will be implicitly converted into a QBluetoothUuid when necessary. The detailed type descriptions can be found on bluetooth.org.
Constant | Value | Description |
---|---|---|
QBluetoothUuid::CharacteristicType::AerobicHeartRateLowerLimit |
0x2a7e |
The lower limit of the heart rate where the user improves his endurance while exercising. |
QBluetoothUuid::CharacteristicType::AerobicHeartRateUpperLimit |
0x2a84 |
The upper limit of the heart rate where the user improves his endurance while exercising. |
QBluetoothUuid::CharacteristicType::AerobicThreshold |
0x2a7f |
This characteristic states the first metabolic threshold. |
QBluetoothUuid::CharacteristicType::Age |
0x2a80 |
This characteristic states the age of the user. |
QBluetoothUuid::CharacteristicType::AnaerobicHeartRateLowerLimit |
0x2a81 |
The lower limit of the heart rate where the user enhances his anaerobic tolerance while exercising. |
QBluetoothUuid::CharacteristicType::AnaerobicHeartRateUpperLimit |
0x2a82 |
The upper limit of the heart rate where the user enhances his anaerobic tolerance while exercising. |
QBluetoothUuid::CharacteristicType::AnaerobicThreshold |
0x2a83 |
This characteristic states the second metabolic threshold. |
QBluetoothUuid::CharacteristicType::AlertCategoryID |
0x2a43 |
Categories of alerts/messages. |
QBluetoothUuid::CharacteristicType::AlertCategoryIDBitMask |
0x2a42 |
Categories of alerts/messages. |
QBluetoothUuid::CharacteristicType::AlertLevel |
0x2a06 |
The level of an alert a device is to sound. If this level is changed while the alert is being sounded, the new level should take effect. |
QBluetoothUuid::CharacteristicType::AlertNotificationControlPoint |
0x2a44 |
Control point of the Alert Notification server. Client can write the command here to request the several functions toward the server. |
QBluetoothUuid::CharacteristicType::AlertStatus |
0x2a3f |
The Alert Status characteristic defines the Status of alert. |
QBluetoothUuid::CharacteristicType::ApparentWindDirection |
0x2a73 |
The characteristic exposes the apparent wind direction. The apparent wind is experienced by an observer in motion. This characteristic states the direction of the wind with an angle measured clockwise relative to the observers heading. |
QBluetoothUuid::CharacteristicType::ApparentWindSpeed |
0x2a72 |
The characteristic exposes the apparent wind speed in meters per second. The apparent wind is experienced by an observer in motion. |
QBluetoothUuid::CharacteristicType::Appearance |
0x2a01 |
The external appearance of this device. The values are composed of a category (10-bits) and sub-categories (6-bits). |
QBluetoothUuid::CharacteristicType::BarometricPressureTrend |
0x2aa3 |
This characteristic exposes the trend the barometric pressure is taking. |
QBluetoothUuid::CharacteristicType::BatteryLevel |
0x2a19 |
The current charge level of a battery. 100% represents fully charged while 0% represents fully discharged. |
QBluetoothUuid::CharacteristicType::BloodPressureFeature |
0x2a49 |
The Blood Pressure Feature characteristic is used to describe the supported features of the Blood Pressure Sensor. |
QBluetoothUuid::CharacteristicType::BloodPressureMeasurement |
0x2a35 |
The Blood Pressure Measurement characteristic is a variable length structure containing a Flags field, a Blood Pressure Measurement Compound Value field, and contains additional fields such as Time Stamp, Pulse Rate and User ID as determined by the contents of the Flags field. |
QBluetoothUuid::CharacteristicType::BodyCompositionFeature |
0x2a9b |
This characteristic describes the available features in the BodyCompositionMeasurement characteristic. |
QBluetoothUuid::CharacteristicType::BodyCompositionMeasurement |
0x2a9c |
This characteristic describes the body composition such as muscle percentage or the body water mass. |
QBluetoothUuid::CharacteristicType::BodySensorLocation |
0x2a38 |
The Body Sensor Location characteristic describes the location of a sensor on the body (e.g.: chest, finger or hand). |
QBluetoothUuid::CharacteristicType::BootKeyboardInputReport |
0x2a22 |
The Boot Keyboard Input Report characteristic is used to transfer fixed format and length Input Report data between a HID Host operating in Boot Protocol Mode and a HID Service corresponding to a boot keyboard. |
QBluetoothUuid::CharacteristicType::BootKeyboardOutputReport |
0x2a32 |
The Boot Keyboard Output Report characteristic is used to transfer fixed format and length Output Report data between a HID Host operating in Boot Protocol Mode and a HID Service corresponding to a boot keyboard. |
QBluetoothUuid::CharacteristicType::BootMouseInputReport |
0x2a33 |
The Boot Mouse Input Report characteristic is used to transfer fixed format and length Input Report data between a HID Host operating in Boot Protocol Mode and a HID Service corresponding to a boot mouse. |
QBluetoothUuid::CharacteristicType::CSCFeature |
0x2a5c |
The CSC (Cycling Speed and Cadence) Feature characteristic is used to describe the supported features of the Server. |
QBluetoothUuid::CharacteristicType::CSCMeasurement |
0x2a5b |
The CSC Measurement characteristic (CSC refers to Cycling Speed and Cadence) is a variable length structure containing a Flags field and, based on the contents of the Flags field, may contain one or more additional fields as shown in the tables below. |
QBluetoothUuid::CharacteristicType::CurrentTime |
0x2a2b |
The Current Time characteristic shows the same information as the ExactTime256 characteristic and information on timezone, DST and the method of update employed. |
QBluetoothUuid::CharacteristicType::CyclingPowerControlPoint |
0x2a66 |
The Cycling Power Control Point characteristic is used to request a specific function to be executed on the receiving device. |
QBluetoothUuid::CharacteristicType::CyclingPowerFeature |
0x2a65 |
The CP Feature characteristic is used to report a list of features supported by the device. |
QBluetoothUuid::CharacteristicType::CyclingPowerMeasurement |
0x2a63 |
The Cycling Power Measurement characteristic is a variable length structure containing a Flags field, an Instantaneous Power field and, based on the contents of the Flags field, may contain one or more additional fields as shown in the table below. |
QBluetoothUuid::CharacteristicType::CyclingPowerVector |
0x2a64 |
The Cycling Power Vector characteristic is a variable length structure containing a Flags fieldand based on the contents of the Flags field, may contain one or more additional fields as shown in the table below. |
QBluetoothUuid::CharacteristicType::DatabaseChangeIncrement |
0x2a99 |
|
QBluetoothUuid::CharacteristicType::DateOfBirth |
0x2a85 |
This characteristic states the user's date of birth. |
QBluetoothUuid::CharacteristicType::DateOfThresholdAssessment |
0x2a86 |
|
QBluetoothUuid::CharacteristicType::DateTime |
0x2a08 |
The Date Time characteristic is used to represent time. |
QBluetoothUuid::CharacteristicType::DayDateTime |
0x2a0a |
The Day Date Time characteristic presents the date, time and day of the week. |
QBluetoothUuid::CharacteristicType::DayOfWeek |
0x2a09 |
The Day of Week characteristic describes the day of the week (Monday - Sunday). |
QBluetoothUuid::CharacteristicType::DescriptorValueChanged |
0x2a7d |
This characteristic is related to the Environmental Sensing Service. |
QBluetoothUuid::CharacteristicType::DeviceName |
0x2a00 |
The Device Name characteristic contains the name of the device. |
QBluetoothUuid::CharacteristicType::DewPoint |
0x2a7b |
This characteristic states the dew point in degree Celsius. |
QBluetoothUuid::CharacteristicType::DSTOffset |
0x2a0d |
The DST Offset characteristic describes the offset employed by daylight-saving time. |
QBluetoothUuid::CharacteristicType::Elevation |
0x2a6c |
The Elevation characteristic states the elevation above/below sea level. |
QBluetoothUuid::CharacteristicType::EmailAddress |
0x2a87 |
This characteristic states the email of the user. |
QBluetoothUuid::CharacteristicType::ExactTime256 |
0x2a0c |
The Exact Time 256 characteristic describes the data, day and time with an accuracy of 1/256th of a second. |
QBluetoothUuid::CharacteristicType::FatBurnHeartRateLowerLimit |
0x2a88 |
The lower limit of the heart rate where the user maximizes the fat burn while exercising. |
QBluetoothUuid::CharacteristicType::FatBurnHeartRateUpperLimit |
0x2a89 |
The upper limit of the heart rate where the user maximizes the fat burn while exercising. |
QBluetoothUuid::CharacteristicType::FirmwareRevisionString |
0x2a26 |
The value of this characteristic is a UTF-8 string representing the firmware revision for the firmware within the device. |
QBluetoothUuid::CharacteristicType::FirstName |
0x2a8a |
This characteristic exposes the user's first name. |
QBluetoothUuid::CharacteristicType::FiveZoneHeartRateLimits |
0x2a8b |
This characteristic contains the limits between the heart rate zones for the 5-zone heart rate definition. |
QBluetoothUuid::CharacteristicType::Gender |
0x2a8c |
This characteristic states the user's gender. |
QBluetoothUuid::CharacteristicType::GlucoseFeature |
0x2a51 |
The Glucose Feature characteristic is used to describe the supported features of the Server. When read, the Glucose Feature characteristic returns a value that is used by a Client to determine the supported features of the Server. |
QBluetoothUuid::CharacteristicType::GlucoseMeasurement |
0x2a18 |
The Glucose Measurement characteristic is a variable length structure containing a Flags field, a Sequence Number field, a Base Time field and, based upon the contents of the Flags field, may contain a Time Offset field, Glucose Concentration field, Type-Sample Location field and a Sensor Status Annunciation field. |
QBluetoothUuid::CharacteristicType::GlucoseMeasurementContext |
0x2a34 |
|
QBluetoothUuid::CharacteristicType::GustFactor |
0x2a74 |
The characteristic states a factor of wind speed increase between average wind speed in maximum gust speed. |
QBluetoothUuid::CharacteristicType::HardwareRevisionString |
0x2a27 |
The value of this characteristic is a UTF-8 string representing the hardware revision for the hardware within the device. |
QBluetoothUuid::CharacteristicType::MaximumRecommendedHeartRate |
0x2a91 |
This characteristic exposes the maximum recommended heart rate that limits exertion. |
QBluetoothUuid::CharacteristicType::HeartRateControlPoint |
0x2a39 |
|
QBluetoothUuid::CharacteristicType::HeartRateMax |
0x2a8d |
This characteristic states the maximum heart rate a user can reach in beats per minute. |
QBluetoothUuid::CharacteristicType::HeartRateMeasurement |
0x2a37 |
|
QBluetoothUuid::CharacteristicType::HeatIndex |
0x2a7a |
This characteristic provides a heat index in degree Celsius. |
QBluetoothUuid::CharacteristicType::Height |
0x2a8e |
This characteristic states the user's height. |
QBluetoothUuid::CharacteristicType::HIDControlPoint |
0x2a4c |
The HID Control Point characteristic is a control-point attribute that defines the HID Commands when written. |
QBluetoothUuid::CharacteristicType::HIDInformation |
0x2a4a |
The HID Information Characteristic returns the HID attributes when read. |
QBluetoothUuid::CharacteristicType::HipCircumference |
0x2a8f |
This characteristic states the user's hip circumference in meters. |
QBluetoothUuid::CharacteristicType::Humidity |
0x2a6f |
The characteristic states the humidity in percent. |
QBluetoothUuid::CharacteristicType::IEEE1107320601RegulatoryCertificationDataList |
0x2a2a |
The value of the characteristic is an opaque structure listing various regulatory and/or certification compliance items to which the device claims adherence. |
QBluetoothUuid::CharacteristicType::IntermediateCuffPressure |
0x2a36 |
This characteristic has the same format as the Blood Pressure Measurement characteristic. |
QBluetoothUuid::CharacteristicType::IntermediateTemperature |
0x2a1e |
The Intermediate Temperature characteristic has the same format as the Temperature Measurement characteristic. |
QBluetoothUuid::CharacteristicType::Irradiance |
0x2a77 |
This characteristic states the power of electromagnetic radiation in watt per square meter. |
QBluetoothUuid::CharacteristicType::Language |
0x2aa2 |
This characteristic contains the language definition based on ISO639-1. |
QBluetoothUuid::CharacteristicType::LastName |
0x2a90 |
This characteristic states the user's last name. |
QBluetoothUuid::CharacteristicType::LNControlPoint |
0x2a6b |
The LN Control Point characteristic is used to request a specific function to be executed on the receiving device. |
QBluetoothUuid::CharacteristicType::LNFeature |
0x2a6a |
The LN Feature characteristic is used to report a list of features supported by the device. |
QBluetoothUuid::CharacteristicType::LocalTimeInformation |
0x2a0f |
|
QBluetoothUuid::CharacteristicType::LocationAndSpeed |
0x2a67 |
The Location and Speed characteristic is a variable length structure containing a Flags field and, based on the contents of the Flags field, may contain a combination of data fields. |
QBluetoothUuid::CharacteristicType::MagneticDeclination |
0x2a2c |
The characteristic contains the angle on the horizontal plane between the direction of the (Geographic) True North and the Magnetic North, measured clockwise from True North to Magnetic North. |
QBluetoothUuid::CharacteristicType::MagneticFluxDensity2D |
0x2aa0 |
This characteristic states the magnetic flux density on an x and y axis. |
QBluetoothUuid::CharacteristicType::MagneticFluxDensity3D |
0x2aa1 |
This characteristic states the magnetic flux density on an x, y and z axis. |
QBluetoothUuid::CharacteristicType::ManufacturerNameString |
0x2a29 |
The value of this characteristic is a UTF-8 string representing the name of the manufacturer of the device. |
QBluetoothUuid::CharacteristicType::MeasurementInterval |
0x2a21 |
The Measurement Interval characteristic defines the time between measurements. |
QBluetoothUuid::CharacteristicType::ModelNumberString |
0x2a24 |
The value of this characteristic is a UTF-8 string representing the model number assigned by the device vendor. |
QBluetoothUuid::CharacteristicType::Navigation |
0x2a68 |
The Navigation characteristic is a variable length structure containing a Flags field, a Bearing field, a Heading field and, based on the contents of the Flags field. |
QBluetoothUuid::CharacteristicType::NewAlert |
0x2a46 |
This characteristic defines the category of the alert and how many new alerts of that category have occurred in the server device. |
QBluetoothUuid::CharacteristicType::PeripheralPreferredConnectionParameters |
0x2a04 |
|
QBluetoothUuid::CharacteristicType::PeripheralPrivacyFlag |
0x2a02 |
|
QBluetoothUuid::CharacteristicType::PnPID |
0x2a50 |
The PnP_ID characteristic returns its value when read using the GATT Characteristic Value Read procedure. |
QBluetoothUuid::CharacteristicType::PollenConcentration |
0x2a75 |
The characteristic exposes the pollen concentration count per cubic meter. |
QBluetoothUuid::CharacteristicType::PositionQuality |
0x2a69 |
The Position Quality characteristic is a variable length structure containing a Flags field and at least one of the optional data. |
QBluetoothUuid::CharacteristicType::Pressure |
0x2a6d |
The Pressure characteristic states the value of a pressure sensor. |
QBluetoothUuid::CharacteristicType::ProtocolMode |
0x2a4e |
The Protocol Mode characteristic is used to expose the current protocol mode of the HID Service with which it is associated, or to set the desired protocol mode of the HID Service. |
QBluetoothUuid::CharacteristicType::Rainfall |
0x2a78 |
This characteristic exposes the rainfall in meters. |
QBluetoothUuid::CharacteristicType::ReconnectionAddress |
0x2a03 |
The Information included in this page is informative. The normative descriptions are contained in the applicable specification. |
QBluetoothUuid::CharacteristicType::RecordAccessControlPoint |
0x2a52 |
This control point is used with a service to provide basic management functionality for the Glucose Sensor patient record database. |
QBluetoothUuid::CharacteristicType::ReferenceTimeInformation |
0x2a14 |
|
QBluetoothUuid::CharacteristicType::Report |
0x2a4d |
The Report characteristic is used to exchange data between a HID Device and a HID Host. |
QBluetoothUuid::CharacteristicType::ReportMap |
0x2a4b |
Only a single instance of this characteristic exists as part of a HID Service. |
QBluetoothUuid::CharacteristicType::RestingHeartRate |
0x2a92 |
This characteristic exposes the lowest heart rate a user can reach. |
QBluetoothUuid::CharacteristicType::RingerControlPoint |
0x2a40 |
The Ringer Control Point characteristic defines the Control Point of Ringer. |
QBluetoothUuid::CharacteristicType::RingerSetting |
0x2a41 |
The Ringer Setting characteristic defines the Setting of the Ringer. |
QBluetoothUuid::CharacteristicType::RSCFeature |
0x2a54 |
The RSC (Running Speed and Cadence) Feature characteristic is used to describe the supported features of the Server. |
QBluetoothUuid::CharacteristicType::RSCMeasurement |
0x2a53 |
RSC refers to Running Speed and Cadence. |
QBluetoothUuid::CharacteristicType::SCControlPoint |
0x2a55 |
The SC Control Point characteristic is used to request a specific function to be executed on the receiving device. |
QBluetoothUuid::CharacteristicType::ScanIntervalWindow |
0x2a4f |
The Scan Interval Window characteristic is used to store the scan parameters of the GATT Client. |
QBluetoothUuid::CharacteristicType::ScanRefresh |
0x2a31 |
The Scan Refresh characteristic is used to notify the Client that the Server requires the Scan Interval Window characteristic to be written with the latest values upon notification. |
QBluetoothUuid::CharacteristicType::SensorLocation |
0x2a5d |
The Sensor Location characteristic is used to expose the location of the sensor. |
QBluetoothUuid::CharacteristicType::SerialNumberString |
0x2a25 |
The value of this characteristic is a variable-length UTF-8 string representing the serial number for a particular instance of the device. |
QBluetoothUuid::CharacteristicType::ServiceChanged |
0x2a05 |
|
QBluetoothUuid::CharacteristicType::SoftwareRevisionString |
0x2a28 |
The value of this characteristic is a UTF-8 string representing the software revision for the software within the device. |
QBluetoothUuid::CharacteristicType::SportTypeForAerobicAnaerobicThresholds |
0x2a93 |
This characteristic is used to preset the various Aerobic and Anaerobic threshold characteristics based on the to-be-performed sport type. |
QBluetoothUuid::CharacteristicType::SupportedNewAlertCategory |
0x2a47 |
Category that the server supports for new alert. |
QBluetoothUuid::CharacteristicType::SupportedUnreadAlertCategory |
0x2a48 |
Category that the server supports for unread alert. |
QBluetoothUuid::CharacteristicType::SystemID |
0x2a23 |
If the system ID is based of a Bluetooth Device Address with a Company Identifier (OUI) is 0x123456 and the Company Assigned Identifier is 0x9ABCDE, then the System Identifier is required to be 0x123456FFFE9ABCDE. |
QBluetoothUuid::CharacteristicType::Temperature |
0x2a6e |
The value of this characteristic states the temperature in degree Celsius. |
QBluetoothUuid::CharacteristicType::TemperatureMeasurement |
0x2a1c |
The Temperature Measurement characteristic is a variable length structure containing a Flags field, a Temperature Measurement Value field and, based upon the contents of the Flags field, optionally a Time Stamp field and/or a Temperature Type field. |
QBluetoothUuid::CharacteristicType::TemperatureType |
0x2a1d |
The Temperature Type characteristic is an enumeration that indicates where the temperature was measured. |
QBluetoothUuid::CharacteristicType::ThreeZoneHeartRateLimits |
0x2a94 |
This characteristic contains the limits between the heart rate zones for the 3-zone heart rate definition. |
QBluetoothUuid::CharacteristicType::TimeAccuracy |
0x2a12 |
|
QBluetoothUuid::CharacteristicType::TimeSource |
0x2a13 |
|
QBluetoothUuid::CharacteristicType::TimeUpdateControlPoint |
0x2a16 |
|
QBluetoothUuid::CharacteristicType::TimeUpdateState |
0x2a17 |
|
QBluetoothUuid::CharacteristicType::TimeWithDST |
0x2a11 |
|
QBluetoothUuid::CharacteristicType::TimeZone |
0x2a0e |
|
QBluetoothUuid::CharacteristicType::TrueWindDirection |
0x2a71 |
The characteristic states the direction of the wind with an angle measured clockwise relative to (Geographic) True North. A wind coming from the east is given as 90 degrees. |
QBluetoothUuid::CharacteristicType::TrueWindSpeed |
0x2a70 |
The characteristic states the wind speed in meters per seconds. |
QBluetoothUuid::CharacteristicType::TwoZoneHeartRateLimits |
0x2a95 |
This characteristic contains the limits between the heart rate zones for the 2-zone heart rate definition. |
QBluetoothUuid::CharacteristicType::TxPowerLevel |
0x2a07 |
The value of the characteristic is a signed 8 bit integer that has a fixed point exponent of 0. |
QBluetoothUuid::CharacteristicType::UnreadAlertStatus |
0x2a45 |
This characteristic shows how many numbers of unread alerts exist in the specific category in the device. |
QBluetoothUuid::CharacteristicType::UserControlPoint |
0x2a9f |
|
QBluetoothUuid::CharacteristicType::UserIndex |
0x2a9a |
This characteristic states the index of the user. |
QBluetoothUuid::CharacteristicType::UVIndex |
0x2a76 |
This characteristic exposes the UV index. |
QBluetoothUuid::CharacteristicType::VO2Max |
0x2a96 |
This characteristic exposes the maximum Oxygen uptake of a user. |
QBluetoothUuid::CharacteristicType::WaistCircumference |
0x2a97 |
This characteristic states the user's waist circumference in meters. |
QBluetoothUuid::CharacteristicType::Weight |
0x2a98 |
This characteristic exposes the user's weight in kilograms. |
QBluetoothUuid::CharacteristicType::WeightMeasurement |
0x2a9d |
This characteristic provides weight related data such as BMI or the user's weight. |
QBluetoothUuid::CharacteristicType::WeightScaleFeature |
0x2a9e |
This characteristic describes the available data in the WeightMeasurement characteristic. |
QBluetoothUuid::CharacteristicType::WindChill |
0x2a79 |
This characteristic states the wind chill in degree Celsius |
This enum was introduced or modified in Qt 5.4.
[since 5.4]
enum class QBluetoothUuid::DescriptorTypeDescriptors are attributes that describe Bluetooth Low Energy characteristic values.
This enum is a convienience type for descriptor class UUIDs. Values of this type will be implicitly converted into a QBluetoothUuid when necessary. The detailed type specifications can be found on bluetooth.org.
Constant | Value | Description |
---|---|---|
QBluetoothUuid::DescriptorType::CharacteristicExtendedProperties |
0x2900 |
Descriptor defines additional Characteristic Properties. The existence of this descriptor is indicated by the QLowEnergyCharacteristic::ExtendedProperty flag. |
QBluetoothUuid::DescriptorType::CharacteristicUserDescription |
0x2901 |
Descriptor provides a textual user description for a characteristic value. |
QBluetoothUuid::DescriptorType::ClientCharacteristicConfiguration |
0x2902 |
Descriptor defines how the characteristic may be configured by a specific client. |
QBluetoothUuid::DescriptorType::ServerCharacteristicConfiguration |
0x2903 |
Descriptor defines how the characteristic descriptor is associated with may be configured for the server. |
QBluetoothUuid::DescriptorType::CharacteristicPresentationFormat |
0x2904 |
Descriptor defines the format of the Characteristic Value. |
QBluetoothUuid::DescriptorType::CharacteristicAggregateFormat |
0x2905 |
Descriptor defines the format of an aggregated Characteristic Value. |
QBluetoothUuid::DescriptorType::ValidRange |
0x2906 |
descriptor is used for defining the range of a characteristics. Two mandatory fields are contained (upper and lower bounds) which define the range. |
QBluetoothUuid::DescriptorType::ExternalReportReference |
0x2907 |
Allows a HID Host to map information from the Report Map characteristic value for Input Report, Output Report or Feature Report data to the Characteristic UUID of external service characteristics used to transfer the associated data. |
QBluetoothUuid::DescriptorType::ReportReference |
0x2908 |
Mapping information in the form of a Report ID and Report Type which maps the current parent characteristic to the Report ID(s) and Report Type (s) defined within the Report Map characteristic. |
QBluetoothUuid::DescriptorType::EnvironmentalSensingConfiguration |
0x290b |
Descriptor defines how multiple trigger settings descriptors are combined. Therefore this descriptor works together with the EnvironmentalSensingTriggerSetting descriptor to define the conditions under which the associated characteristic value can be notified. |
QBluetoothUuid::DescriptorType::EnvironmentalSensingMeasurement |
0x290c |
Descriptor defines the additional information for the environmental sensing server such as the intended application, sampling functions or measurement period and uncertainty. |
QBluetoothUuid::DescriptorType::EnvironmentalSensingTriggerSetting |
0x290d |
Descriptor defines under which conditions an environmental sensing server (ESS) should trigger notifications. Examples of such conditions are certain thresholds being reached or timers having expired. This implies that the ESS characteristic supports notifications. |
QBluetoothUuid::DescriptorType::UnknownDescriptorType |
0x0 |
The descriptor type is unknown. |
This enum was introduced or modified in Qt 5.4.
This enum is a convienience type for Bluetooth protocol UUIDs. Values of this type will be implicitly converted into a QBluetoothUuid when necessary.
Constant | Value | Description |
---|---|---|
QBluetoothUuid::ProtocolUuid::Sdp |
0x0001 |
SDP protocol UUID |
QBluetoothUuid::ProtocolUuid::Udp |
0x0002 |
UDP protocol UUID |
QBluetoothUuid::ProtocolUuid::Rfcomm |
0x0003 |
RFCOMM protocol UUID |
QBluetoothUuid::ProtocolUuid::Tcp |
0x0004 |
TCP protocol UUID |
QBluetoothUuid::ProtocolUuid::TcsBin |
0x0005 |
Telephony Control Specification UUID |
QBluetoothUuid::ProtocolUuid::TcsAt |
0x0006 |
Telephony Control Specification AT UUID |
QBluetoothUuid::ProtocolUuid::Att |
0x0007 |
Attribute protocol UUID |
QBluetoothUuid::ProtocolUuid::Obex |
0x0008 |
OBEX protocol UUID |
QBluetoothUuid::ProtocolUuid::Ip |
0x0009 |
IP protocol UUID |
QBluetoothUuid::ProtocolUuid::Ftp |
0x000A |
FTP protocol UUID |
QBluetoothUuid::ProtocolUuid::Http |
0x000C |
HTTP protocol UUID |
QBluetoothUuid::ProtocolUuid::Wsp |
0x000E |
WSP UUID |
QBluetoothUuid::ProtocolUuid::Bnep |
0x000F |
Bluetooth Network Encapsulation Protocol UUID |
QBluetoothUuid::ProtocolUuid::Upnp |
0x0010 |
Extended Service Discovery Profile UUID |
QBluetoothUuid::ProtocolUuid::Hidp |
0x0011 |
Human Interface Device Profile UUID |
QBluetoothUuid::ProtocolUuid::HardcopyControlChannel |
0x0012 |
Hardcopy Cable Replacement Profile UUID |
QBluetoothUuid::ProtocolUuid::HardcopyDataChannel |
0x0014 |
Hardcopy Cable Replacement Profile UUID |
QBluetoothUuid::ProtocolUuid::HardcopyNotification |
0x0016 |
Hardcopy Cable Replacement Profile UUID |
QBluetoothUuid::ProtocolUuid::Avctp |
0x0017 |
Audio/Video Control Transport Protocol UUID |
QBluetoothUuid::ProtocolUuid::Avdtp |
0x0019 |
Audio/Video Distribution Transport Protocol UUID |
QBluetoothUuid::ProtocolUuid::Cmtp |
0x001B |
Common ISDN Access Profile |
QBluetoothUuid::ProtocolUuid::UdiCPlain |
0x001D |
UDI protocol UUID |
QBluetoothUuid::ProtocolUuid::McapControlChannel |
0x001E |
Multi-Channel Adaptation Protocol UUID |
QBluetoothUuid::ProtocolUuid::McapDataChannel |
0x001F |
Multi-Channel Adaptation Protocol UUID |
QBluetoothUuid::ProtocolUuid::L2cap |
0x0100 |
L2CAP protocol UUID |
See also QBluetoothServiceInfo::ProtocolDescriptorList.
This enum is a convienience type for Bluetooth service class and profile UUIDs. Values of this type will be implicitly converted into a QBluetoothUuid when necessary. Some UUIDs refer to service class ids, others to profile ids and some can be used as both. In general, profile UUIDs shall only be used in a QBluetoothServiceInfo::BluetoothProfileDescriptorList attribute and service class UUIDs shall only be used in a QBluetoothServiceInfo::ServiceClassIds attribute. If the UUID is marked as profile and service class UUID it can be used as a value for either of the above service attributes. Such a dual use has historical reasons but is no longer permissible for newer UUIDs.
The list below explicitly states as what type each UUID shall be used. Bluetooth Low Energy related values starting with 0x18 were introduced by Qt 5.4
Constant | Value | Description |
---|---|---|
QBluetoothUuid::ServiceClassUuid::ServiceDiscoveryServer |
0x1000 |
Service discovery server UUID (service) |
QBluetoothUuid::ServiceClassUuid::BrowseGroupDescriptor |
0x1001 |
Browser group descriptor (service) |
QBluetoothUuid::ServiceClassUuid::PublicBrowseGroup |
0x1002 |
Public browse group service class. Services which have the public browse group in their browse group list are discoverable by the remote devices. |
QBluetoothUuid::ServiceClassUuid::SerialPort |
0x1101 |
Serial Port Profile UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::LANAccessUsingPPP |
0x1102 |
LAN Access Profile UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::DialupNetworking |
0x1103 |
Dial-up Networking Profile UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::IrMCSync |
0x1104 |
Synchronization Profile UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::ObexObjectPush |
0x1105 |
OBEX object push service UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::OBEXFileTransfer |
0x1106 |
File Transfer Profile (FTP) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::IrMCSyncCommand |
0x1107 |
Synchronization Profile UUID (profile) |
QBluetoothUuid::ServiceClassUuid::Headset |
0x1108 |
Headset Profile (HSP) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::AudioSource |
0x110a |
Advanced Audio Distribution Profile (A2DP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::AudioSink |
0x110b |
Advanced Audio Distribution Profile (A2DP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::AV_RemoteControlTarget |
0x110c |
Audio/Video Remote Control Profile (AVRCP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::AdvancedAudioDistribution |
0x110d |
Advanced Audio Distribution Profile (A2DP) UUID (profile) |
QBluetoothUuid::ServiceClassUuid::AV_RemoteControl |
0x110e |
Audio/Video Remote Control Profile (AVRCP) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::AV_RemoteControlController |
0x110f |
Audio/Video Remote Control Profile UUID (service) |
QBluetoothUuid::ServiceClassUuid::HeadsetAG |
0x1112 |
Headset Profile (HSP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::PANU |
0x1115 |
Personal Area Networking Profile (PAN) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::NAP |
0x1116 |
Personal Area Networking Profile (PAN) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::GN |
0x1117 |
Personal Area Networking Profile (PAN) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::DirectPrinting |
0x1118 |
Basic Printing Profile (BPP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::ReferencePrinting |
0x1119 |
Related to Basic Printing Profile (BPP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::BasicImage |
0x111a |
Basic Imaging Profile (BIP) UUID (profile) |
QBluetoothUuid::ServiceClassUuid::ImagingResponder |
0x111b |
Basic Imaging Profile (BIP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::ImagingAutomaticArchive |
0x111c |
Basic Imaging Profile (BIP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::ImagingReferenceObjects |
0x111d |
Basic Imaging Profile (BIP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::Handsfree |
0x111e |
Hands-Free Profile (HFP) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::HandsfreeAudioGateway |
0x111f |
Hands-Free Audio Gateway (HFP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::DirectPrintingReferenceObjectsService |
0x1120 |
Basic Printing Profile (BPP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::ReflectedUI |
0x1121 |
Basic Printing Profile (BPP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::BasicPrinting |
0x1122 |
Basic Printing Profile (BPP) UUID (profile) |
QBluetoothUuid::ServiceClassUuid::PrintingStatus |
0x1123 |
Basic Printing Profile (BPP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::HumanInterfaceDeviceService |
0x1124 |
Human Interface Device (HID) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::HardcopyCableReplacement |
0x1125 |
Hardcopy Cable Replacement Profile (HCRP) (profile) |
QBluetoothUuid::ServiceClassUuid::HCRPrint |
0x1126 |
Hardcopy Cable Replacement Profile (HCRP) (service) |
QBluetoothUuid::ServiceClassUuid::HCRScan |
0x1127 |
Hardcopy Cable Replacement Profile (HCRP) (service) |
QBluetoothUuid::ServiceClassUuid::SIMAccess |
0x112d |
SIM Access Profile (SAP) UUID (service and profile) |
QBluetoothUuid::ServiceClassUuid::PhonebookAccessPCE |
0x112e |
Phonebook Access Profile (PBAP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::PhonebookAccessPSE |
0x112f |
Phonebook Access Profile (PBAP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::PhonebookAccess |
0x1130 |
Phonebook Access Profile (PBAP) (profile) |
QBluetoothUuid::ServiceClassUuid::HeadsetHS |
0x1131 |
Headset Profile (HSP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::MessageAccessServer |
0x1132 |
Message Access Profile (MAP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::MessageNotificationServer |
0x1133 |
Message Access Profile (MAP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::MessageAccessProfile |
0x1134 |
Message Access Profile (MAP) UUID (profile) |
QBluetoothUuid::ServiceClassUuid::GNSS |
0x1135 |
Global Navigation Satellite System UUID (profile) |
QBluetoothUuid::ServiceClassUuid::GNSSServer |
0x1136 |
Global Navigation Satellite System Server (UUID) (service) |
QBluetoothUuid::ServiceClassUuid::Display3D |
0x1137 |
3D Synchronization Display UUID (service) |
QBluetoothUuid::ServiceClassUuid::Glasses3D |
0x1138 |
3D Synchronization Glasses UUID (service) |
QBluetoothUuid::ServiceClassUuid::Synchronization3D |
0x1139 |
3D Synchronization UUID (profile) |
QBluetoothUuid::ServiceClassUuid::MPSProfile |
0x113a |
Multi-Profile Specification UUID (profile) |
QBluetoothUuid::ServiceClassUuid::MPSService |
0x113b |
Multi-Profile Specification UUID (service) |
QBluetoothUuid::ServiceClassUuid::PnPInformation |
0x1200 |
Device Identification (DID) UUID (service & profile) |
QBluetoothUuid::ServiceClassUuid::GenericNetworking |
0x1201 |
Generic networking UUID (service) |
QBluetoothUuid::ServiceClassUuid::GenericFileTransfer |
0x1202 |
Generic file transfer UUID (service) |
QBluetoothUuid::ServiceClassUuid::GenericAudio |
0x1203 |
Generic audio UUID (service) |
QBluetoothUuid::ServiceClassUuid::GenericTelephony |
0x1204 |
Generic telephone UUID (service) |
QBluetoothUuid::ServiceClassUuid::VideoSource |
0x1303 |
Video Distribution Profile (VDP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::VideoSink |
0x1304 |
Video Distribution Profile (VDP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::VideoDistribution |
0x1305 |
Video Distribution Profile (VDP) UUID (profile) |
QBluetoothUuid::ServiceClassUuid::HDP |
0x1400 |
Health Device Profile (HDP) UUID (profile) |
QBluetoothUuid::ServiceClassUuid::HDPSource |
0x1401 |
Health Device Profile Source (HDP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::HDPSink |
0x1402 |
Health Device Profile Sink (HDP) UUID (service) |
QBluetoothUuid::ServiceClassUuid::GenericAccess |
0x1800 |
Generic access service for Bluetooth Low Energy devices UUID (service). It contains generic information about the device. All available Characteristics are readonly. |
QBluetoothUuid::ServiceClassUuid::GenericAttribute |
0x1801 |
|
QBluetoothUuid::ServiceClassUuid::ImmediateAlert |
0x1802 |
Immediate Alert UUID (service). The service exposes a control point to allow a peer device to cause the device to immediately alert. |
QBluetoothUuid::ServiceClassUuid::LinkLoss |
0x1803 |
Link Loss UUID (service). The service defines behavior when a link is lost between two devices. |
QBluetoothUuid::ServiceClassUuid::TxPower |
0x1804 |
Transmission Power UUID (service). The service exposes a device’s current transmit power level when in a connection. |
QBluetoothUuid::ServiceClassUuid::CurrentTimeService |
0x1805 |
Current Time UUID (service). The service defines how the current time can be exposed using the Generic Attribute Profile (GATT). |
QBluetoothUuid::ServiceClassUuid::ReferenceTimeUpdateService |
0x1806 |
Reference Time update UUID (service). The service defines how a client can request an update from a reference time source from a time server. |
QBluetoothUuid::ServiceClassUuid::NextDSTChangeService |
0x1807 |
Next DST change UUID (service). The service defines how the information about an upcoming DST change can be exposed. |
QBluetoothUuid::ServiceClassUuid::Glucose |
0x1808 |
Glucose UUID (service). The service exposes glucose and other data from a glucose sensor for use in consumer and professional healthcare applications. |
QBluetoothUuid::ServiceClassUuid::HealthThermometer |
0x1809 |
Health Thermometer UUID (service). The Health Thermometer service exposes temperature and other data from a thermometer intended for healthcare and fitness applications. |
QBluetoothUuid::ServiceClassUuid::DeviceInformation |
0x180a |
Device Information UUID (service). The Device Information Service exposes manufacturer and/or vendor information about a device. |
QBluetoothUuid::ServiceClassUuid::HeartRate |
0x180d |
Heart Rate UUID (service). The service exposes the heart rate and other data from a Heart Rate Sensor intended for fitness applications. |
QBluetoothUuid::ServiceClassUuid::PhoneAlertStatusService |
0x180e |
Phone Alert Status UUID (service). The service exposes the phone alert status when in a connection. |
QBluetoothUuid::ServiceClassUuid::BatteryService |
0x180f |
Battery UUID (service). The Battery Service exposes the state of a battery within a device. |
QBluetoothUuid::ServiceClassUuid::BloodPressure |
0x1810 |
Blood Pressure UUID (service). The service exposes blood pressure and other data from a blood pressure monitor intended for healthcare applications. |
QBluetoothUuid::ServiceClassUuid::AlertNotificationService |
0x1811 |
Alert Notification UUID (service). The Alert Notification service exposes alert information on a device. |
QBluetoothUuid::ServiceClassUuid::HumanInterfaceDevice |
0x1812 |
Human Interface UUID (service). The service exposes the HID reports and other HID data intended for HID Hosts and HID Devices. |
QBluetoothUuid::ServiceClassUuid::ScanParameters |
0x1813 |
Scan Parameters UUID (service). The Scan Parameters Service enables a GATT Server device to expose a characteristic for the GATT Client to write its scan interval and scan window on the GATT Server device. |
QBluetoothUuid::ServiceClassUuid::RunningSpeedAndCadence |
0x1814 |
Runnung Speed and Cadence UUID (service). The service exposes speed, cadence and other data from a Running Speed and Cadence Sensor intended for fitness applications. |
QBluetoothUuid::ServiceClassUuid::CyclingSpeedAndCadence |
0x1816 |
Cycling Speed and Cadence UUID (service). The service exposes speed-related and cadence-related data from a Cycling Speed and Cadence sensor intended for fitness applications. |
QBluetoothUuid::ServiceClassUuid::CyclingPower |
0x1818 |
Cycling Speed UUID (service). The service exposes power- and force-related data and optionally speed- and cadence-related data from a Cycling Power sensor intended for sports and fitness applications. |
QBluetoothUuid::ServiceClassUuid::LocationAndNavigation |
0x1819 |
Location Navigation UUID (service). The service exposes location and navigation-related data from a Location and Navigation sensor intended for outdoor activity applications. |
QBluetoothUuid::ServiceClassUuid::EnvironmentalSensing |
0x181a |
Environmental sensor UUID (service). The service exposes data from an environmental sensor for sports and fitness applications. |
QBluetoothUuid::ServiceClassUuid::BodyComposition |
0x181b |
Body composition UUID (service). The service exposes data about the body composition intended for consumer healthcare applications. |
QBluetoothUuid::ServiceClassUuid::UserData |
0x181c |
User Data UUID (service). The User Data service provides user-related data such as name, gender or weight in sports and fitness environments. |
QBluetoothUuid::ServiceClassUuid::WeightScale |
0x181d |
Weight Scale UUID (service). The Weight Scale service exposes weight-related data from a scale for consumer healthcare, sports and fitness applications. |
QBluetoothUuid::ServiceClassUuid::BondManagement |
0x181e |
Bond Management UUID (service). The Bond Management service enables user to manage the storage of bond information on Bluetooth devices. |
QBluetoothUuid::ServiceClassUuid::ContinuousGlucoseMonitoring |
0x181f |
Continuous Glucose Monitoring UUID (service). The Continuous Glucose Monitoring service exposes glucose data from a monitoring sensor for use in healthcare applications. |
Constructs a new Bluetooth UUID that is a copy of uuid.
Creates a QBluetoothUuid object from the string uuid, which must be formatted as five hex fields separated by '-', e.g., "{xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx}" where 'x' is a hex digit. The curly braces shown here are optional, but it is normal to include them. If the conversion fails, a null UUID is created. See QUuid::toString() for an explanation of how the five hex fields map to the public data members in QUuid.
Constructs a new Bluetooth UUID from the 128 bit uuid.
Note that uuid must be in big endian order.
Constructs a new Bluetooth UUID from the 32 bit uuid.
Constructs a new Bluetooth UUID from the 16 bit uuid.
[since 5.4]
QBluetoothUuid::QBluetoothUuid(QBluetoothUuid::DescriptorType uuid)Constructs a new Bluetooth UUID from the descriptor type uuid.
This function was introduced in Qt 5.4.
[since 5.4]
QBluetoothUuid::QBluetoothUuid(QBluetoothUuid::CharacteristicType uuid)Constructs a new Bluetooth UUID from the characteristic type uuid.
This function was introduced in Qt 5.4.
Constructs a new Bluetooth UUID from the service class uuid.
Constructs a new Bluetooth UUID from the protocol uuid.
Constructs a new null Bluetooth UUID.
[static, since 5.4]
QString QBluetoothUuid::characteristicToString(QBluetoothUuid::CharacteristicType uuid)Returns a human-readable and translated name for the given characteristic type represented by uuid.
This function was introduced in Qt 5.4.
See also QBluetoothUuid::CharacteristicType.
[static, since 5.4]
QString QBluetoothUuid::descriptorToString(QBluetoothUuid::DescriptorType uuid)Returns a human-readable and translated name for the given descriptor type represented by uuid.
This function was introduced in Qt 5.4.
See also QBluetoothUuid::DescriptorType.
Returns the minimum size in bytes that this UUID can be represented in. For non-null UUIDs 2, 4 or 16 is returned. 0 is returned for null UUIDs.
See also isNull(), toUInt16(), toUInt32(), and toUInt128().
[static, since 5.4]
QString QBluetoothUuid::protocolToString(QBluetoothUuid::ProtocolUuid uuid)Returns a human-readable and translated name for the given protocol represented by uuid.
This function was introduced in Qt 5.4.
See also QBluetoothUuid::ProtocolUuid.
[static, since Qt 5.4]
QString QBluetoothUuid::serviceClassToString(QBluetoothUuid::ServiceClassUuid uuid)Returns a human-readable and translated name for the given service class represented by uuid.
This function was introduced in Qt 5.4.
See also QBluetoothUuid::ServiceClassUuid.
Returns the 16 bit representation of this UUID. If ok is passed, it is set to true if the conversion is possible, otherwise it is set to false. The return value is undefined if ok is set to false.
Returns the 32 bit representation of this UUID. If ok is passed, it is set to true if the conversion is possible, otherwise it is set to false. The return value is undefined if ok is set to false.
Returns the 128 bit representation of this UUID.
Returns true
if a is not equal to b, otherwise false
.
Returns true
if a is equal to b, otherwise false
.