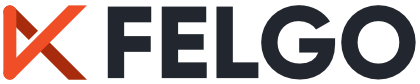
The FirebaseStorage item allows uploading files to the Firebase Storage cloud. It uploads local files to the cloud file system and returns the public download URL. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
FirebaseStorage uploads local files to your Firebase Storage bucket. You can then access the uploaded files via a public download URL. Use uploadFile() to upload local files.
Examples for local files you can upload are:
The following QML example shows how to upload a picture directly from the device camera. It captures a picture using NativeUtils::displayCameraPicker(). After
NativeUtils::cameraPickerFinished() is emitted, it uploads the stored picture using uploadFile(). It
then displays the uploaded file using the downloadUrl
in an AppImage item.
While uploading, the status is displayed in an AppText item.
import QtQuick import Felgo App { NavigationStack { FlickablePage { title: "Firebase Storage" flickable.contentHeight: column.height FirebaseStorage { id: storage } Column { id: column width: parent.width anchors.margins: dp(12) AppButton { text: "Capture image + upload" onClicked: NativeUtils.displayCameraPicker() } AppText { id: status text: "Idle" } // this will display the image after it's uploaded AppImage { id: img width: parent.width fillMode: AppImage.PreserveAspectFit autoTransform: true } } } } Connections { target: nativeUtils onCameraPickerFinished: (accepted, path) => { if(accepted) { //picture taken with camera is stored at path - upload to Firebase Storage storage.uploadFile(path, "test-image" + Date.now() + ".png", function(progress, finished, success, downloadUrl) { if(!finished) { status.text = "Uploading... " + progress.toFixed(2) + "%" } else if(success) { img.source = downloadUrl status.text = "Upload completed." } else { status.text = "Upload failed." } }) } } } }
Here is a similar example that features cancelling the upload with a button using FirebaseStorageUploadTask::cancel().
import QtQuick import Felgo App { property FirebaseStorageUploadTask uploadTask FirebaseStorage { id: storage config: FirebaseConfig { id: customConfig //get these values from the firebase console projectId: "<FB project ID>" storageBucket: "<fb project>.appspot.com/" //platform dependent - get these values from the google-services.json / GoogleService-info.plist apiKey: Qt.platform.os === "android" ? "<android API key>" : "<ios API key>" applicationId: Qt.platform.os === "android" ? "<android app ID>" : "<ios app ID>" } } //UI AppFlickable { anchors.fill: parent anchors.topMargin: Theme.statusBarHeight Column { anchors.left: parent.left anchors.right: parent.right anchors.margins: dp(12) AppButton { text: "Capture image + upload" onClicked: NativeUtils.displayCameraPicker() } AppButton { text: "Cancel upload" onClicked: if(uploadTask) uploadTask.cancel() } AppText { id: status text: "Idle" } AppImage { id: img defaultSource: "vplay-logo.png" autoTransform: true } } } Connections { target: nativeUtils onCameraPickerFinished: (accepted, path) => { if(accepted) { //picture taken with camera is stored at path - upload to Firebase Storage uploadTask = storage.uploadFile(path, "test-image.png", function(progress, finished, success, downloadUrl) { if(!finished) { status.text = "Uploading... " + progress.toFixed(2) + "%" } else if(success) { img.source = downloadUrl status.text = "Upload completed." } else { status.text = "Upload failed." } }) } } } }
Note: Check out the Firebase Plugin page for more examples!
config : FirebaseConfig |
This property defines the account configuration to use for this item. It includes the Firebase project ID
, app ID
, API key
, database URL
and more.
To use the default Firebase account defined in google-services.json and GoogleService-info.plist, do not assign this property or assign null.
To be able to use FirebaseStorage, be sure to set the FirebaseConfig::storageBucket property. You can find the
storageBucket
URL at the Firebase console under Storage.
firebaseReady() |
This signal gets emitted when the plugin is ready to use.
Use this signal instead of PluginItem::pluginLoaded(), as the QML item referenced by config might not yet be loaded when PluginItem::pluginLoaded() is called.
Note: The corresponding handler is onFirebaseReady
.
FirebaseStorageUploadTask uploadFile(url localUrl, string remotePath, var callback) |
Uploads a local file to the Firebase Storage cloud.
localUrl
is a file://
URL representing the local file to upload. You can use one of the FileUtils functions to obtain an URL to a local file you wish to upload.
You can also obtain a file relative to the current QML file with Qt::resolvedUrl().
remotePath
is a relative path in your Firebase Storage bucket to store the file at.
You can listen to the progress of the upload by supplying a JavaScript function as the callback
parameter. This function will be called with four parameters when the upload progress changes:
int progress
: The upload progress, in percent.bool finished
: true
if the upload is no longer ongoing.bool success
: true
if the upload has finished successfully. false
if the upload finished with an error and was not completed.string downloadUrl
: The URL where the uploaded file is accessible. Only present if finished
and success
are both true
.Example call:
FirebaseStorage { id: storage config: FirebaseConfig {} //add config parameters here Component.onCompleted: { var uploadFileUrl = Qt.resolvedUrl("local-image.png") //image in same folder as this QML file var remoteFilePath = "images/remote-image.png" storage.uploadFile(uploadFileUrl, remoteFilePath, function (progress, finished, success, downloadUrl) { if(!finished) { console.log("Upload progress:", progress, "%") } else if(success) { console.log("Upload finished! Download URL:", downloadUrl) } else { console.log("Upload failed!") } } ) } }
The return value of uploadFile() is a QML item of type FirebaseStorageUploadTask. You can use this item to control the upload. Call FirebaseStorageUploadTask::cancel() to cancel the upload.
Example:
FirebaseStorage { id: storage config: FirebaseConfig {} //add config parameters here property FirebaseStorageUploadTask uploadTask Component.onCompleted: { var uploadFileUrl = Qt.resolvedUrl("local-image.png") //image in same folder as this QML file var remoteFilePath = "images/remote-image.png" uploadTask = storage.uploadFile(uploadFileUrl, remoteFilePath, function (progress, finished, success, downloadUrl) { // handle progress } ) } Component.onDestruction: { if(uploadTask) { //cancel ongoing upload uploadTask.cancel() } } }