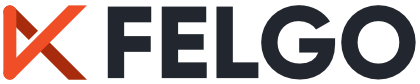
Highlights Blog Post: Release 2.18.3: QML JSON ListModel
Felgo 2.18.3 adds the JsonListModel as a performant and feature-rich QML ListModel enhancement. It helps you transform your JSON data to a model and enables you to detect changes to individual items in your model. You can then only update the changed items in your UI instead of updating the full list. This update also adds several improvements and fixes.
Felgo 2.18.3 comes as a free update for all Felgo developers.
The JsonListModel type implements the full QML ListModel API and fires individual events for all changes in the data. The list view can thus only update relevant entries or apply transition animations. This is super useful, as you can e.g. fetch new data and simply replace the old JSON. The JsonListModel will detect all changes, and the ListView updates its items accordingly - without a full redraw.
You thus get much better performance and scrolling stays smooth when the list is updated:
|
|
Json Model: List jumps to the top after an update. |
JsonListModel: The list keeps its scroll position.
(the GIF only jumps to the top when it restarts) |
With the JsonListModel you do not require to implement a custom model in C++ anymore. The JsonListModel itself is your C++ model, which is fully usable from QML and can work with JSON list items of any format.
Apart from list views, the model also supports the GridView and Repeater types to display model data.
The following example shows how to use JsonListModel together with AppListView. When adding a new item to the JSON, the JsonListModel detects the change. The AppListView can thus use a transition animation when adding the entry. It is not required to fully redraw the list and existing items in the view are not affected.
import Felgo import QtQuick App { NavigationStack { Page { id: page title: "JSONListModel" // property with json data property var jsonData: [ { "id": 1, "title": "Entry 1" }, { "id": 2, "title": "Entry 2" }, { "id": 3, "title": "Entry 3" } ] // list model for json data JsonListModel { id: jsonModel source: page.jsonData keyField: "id" } // list view AppListView { anchors.fill: parent model: jsonModel delegate: SimpleRow { text: model.title } // transition animation for adding items add: Transition { NumberAnimation { property: "opacity"; from: 0; to: 1; duration: 1000 easing.type: Easing.OutQuad; } } } // Button to add a new entry AppButton { anchors.horizontalCenter: parent.horizontalCenter anchors.bottom: parent.bottom text: "Add Entry" onClicked: { var newItem = { "id": jsonModel.count + 1, "title": "Entry "+(jsonModel.count + 1) } page.jsonData.push(newItem) // manually emit signal that jsonData property changed // JsonListModel thus synchronizes the list with the new jsonData page.jsonDataChanged() } } } // Page } }
Please see the documentation of JsonListModel for more information and examples. The JsonListModel is now also used for all JSON models of the Felgo SDK app demos.
You can find the MVC Architecture Demo App along with other app demos in the Felgo SDK and as a project wizard in Qt Creator. The basic app is the best starting point for new applications and combines many features that used to be part of individual wizards before. It is also available for you on GitHub.
It uses list transition animations and shows how to integrate PullToRefreshHandler, VisibilityRefreshHandler or SortFilterProxyModel. The demo supports fetching of todos, creation and storing of drafts, offline caching, paging, sorting and filtering.
Use PRODUCT_VERSION_CODE
and PRODUCT_VERSION_NAME
variables in your *.pro file to update version information for all platforms. The benefit of this new solution is, that you now need update the
version data just at one place instead at multiple places. Version information is then automatically set in your iOS Info.plist file and Android AndroidManifest.xml file every time you run qmake for
your project.
To make use of this new Felgo feature you need to modify existing projects. Totally modify three files to make use of the new variables:
Note: After installing the update, no modification is required for new created projects (wizard).
In the *.pro project configuration add two new properties:
PRODUCT_VERSION_CODE
: Number of the build, a positive integer. Must be higher than the last code used. (e.g. 1,2,3,4)PRODUCT_VERSION_NAME
: Public version number shown to users. (e.g. 1.5, 1.6, 2.0.1)In the build.gradle
file in your projects android folder replace your applicationId
assignment. You can keep the existing defaultConfig
block, but remove the
applicationId
line above and add:
defaultConfig { applicationId = productIdentifier versionCode = productVersionCode.toInteger() versionName = productVersionName }
In the Project-Info.plist
file in your projects ios folder replace the value in the line below the following keys:
CFBundleShortVersionString
-> ${PRODUCT_VERSION_NAME}
CFBundleVersion
-> ${PRODUCT_VERSION_CODE}
Next time you want to update the version data, just change the PRODUCT_VERSION_
properties in your project configuration file and build the app.
You can also specify a custom transition using StackViewDelegate:
import Felgo import QtQuick import QtQuick.Controls App { // NavigationStack NavigationStack { // custom transition delegate transitionDelegate: StackViewDelegate { pushTransition: StackViewTransition { NumberAnimation { target: enterItem property: "opacity" from: 0 to: 1 duration: 1000 } } popTransition: StackViewTransition { NumberAnimation { target: exitItem property: "opacity" from: 1 to: 0 duration: 1000 } } } initialPage: pageComponent } // Component for pages Component { id: pageComponent Page { id: page title: "Page 1" Rectangle { anchors.centerIn: parent color: Qt.rgba(Math.random(255), Math.random(255), Math.random(255)) width: parent.width / 2 height: parent.height / 2 } AppButton { anchors.horizontalCenter: parent.horizontalCenter text: "Push" onClicked: { var properties = { title: "Page " + (page.navigationStack.depth + 1) } page.navigationStack.push(pageComponent, properties) } } } // Page } // Component }
id: textEdit
.
Highlights Blog Post: Release 2.18.2: MVC, MVVM, Flux with QML
Felgo 2.18.2 adds the latest Qt Creator 4.7, as well as many improvements and fixes. A new comprehensive guide helps you to structure your code, and separate logic from UI (MVC, MVVM, Flux).
Felgo 2.18.2 comes as a free update for all Felgo developers.
Update Note: For using the Qt Quick Compiler, which is the default for building in Release Mode with Qt Creator 4.7, it is required to list each qml
file with an own entry in your resources.qrc
. Otherwise, the compiler does not find the QML source files.
To deactivate the QML compiler, you can use the CONFIG -= qtquickcompiler
setting in your *.pro
configuration. This only protects the QML resources and bundles them as assets within your binary.
You can also skip a specific .qrc
configuration with e.g. QTQUICK_COMPILER_SKIPPED_RESOURCES += bundle_only.qrc
.
The guide offers a best-practice solution how to separate model, view and logic components in your Felgo apps:
A clean component architecture and data-flow helps to keep create readable code, avoids bugs and makes maintenance or refactoring easy.
You can find the full example of the guide on GitHub:
It is available as a project wizard template in Qt Creator as well:
All Felgo Apps Examples and Demos now also use this best-practice solution.
This example hides the effect for a single button:
import Felgo App { AppButton { text: "No Ripple Effect" onClicked: console.log("clicked") rippleEffect: false } }
You can also use Theme.appButton.rippleEffect to change your app's default setting:
import Felgo App { onInitTheme: { Theme.appButton.rippleEffect = false } AppButton { text: "No Ripple Effect" onClicked: console.log("clicked") } }
You can use more than one FirebaseConfig instance by assigning a different unique name to each instance. These names are used for persistence and should be the same between app starts.
Highlights Blog Post: Release 2.18.1: JavaScript Promises for REST Services, Tinder Swipe Material Cards, QML QSortFilterProxyModel, QML YouTube Player
Felgo 2.18.1 introduces new components for embedding YouTube videos, for creating material cards and Tinder-like swipe cards. It also simplifies connecting to REST services, with the new HttpRequest component. Felgo 2.18.1 also adds several other fixes and improvements.
Felgo 2.18.1 comes as a free update for all Felgo developers.
The component uses a WebView internally and is based on the YouTube Iframe-Player API. To show how you can use the player in your app, you can have a look at the YouTube Video Player App. It uses the YouTube Data API to browse playlists and videos of a configured channel.
This is how you can use the player in QML:
import Felgo App { NavigationStack { Page { title: "YouTube Player" YouTubeWebPlayer { videoId: "KQgqTYCfJjM" autoplay: true } } } }
New Features
section below and in the documentation.
import Felgo App { NavigationStack { Page { title: "Page" // this code and manual checks of isCurrentStackPage are no longer required onIsCurrentStackPageChanged: { if(isCurrentStackPage) console.log("Page appeared") else console.log("Page disappeared") } // instead, you can use the new signals onAppeared: console.log("Page appeared") onDisappeared: console.log("Page disappeared") } } }
This example shows how all the Page lifecycle events trigger when working with NavigationStack:
Use this snippet to test the signals yourself:
import Felgo import QtQuick App { // navigation stack for pages NavigationStack { initialPage: pageComponent } // page component Component { id: pageComponent Page { id: page title: "Page 1" // allow to push and pop pages dynamically Column { anchors.centerIn: parent // push additional page AppButton { text: "Push" onClicked: page.navigationStack.push(pageComponent, { title: "Page "+(navigationStack.depth + 1) }) } // pop current page AppButton { text: "Pop" onClicked: page.navigationStack.pop() } } // handle lifecycle signals onAppeared: console.log(title+" appeared") onDisappeared: console.log(title+ " disappared") onPushed: console.log(title+ " pushed") onPopped: console.log(title+ " popped") } } }
It is available as a singleton item for all components that use import Felgo
:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .get("http://httpbin.org/get") .timeout(5000) .then(function(res) { console.log(res.status); console.log(JSON.stringify(res.header, null, 4)); console.log(JSON.stringify(res.body, null, 4)); }) .catch(function(err) { console.log(err.message) console.log(err.response) }); } }
Similar to HttpRequest, which matches the DuperAgent Request
type, other DuperAgent features are also available in Felgo with the Http
prefix:
attach
function.
The HttpRequest type also supports response caching of your requests out-of-the-box. To change cache behavior, you can use the HttRequest::config() method. It is also possible to change cache settings for individual requests:
import Felgo import QtQuick App { Component.onCompleted: { HttpRequest .get("http://httpbin.org/get") .cacheSave(true) // cache the result of this request, regardless of global cache setting .cacheLoad(HttpCacheControl.PreferNetwork) // use network if possible, otherwise load from cache .then(function(res) { console.log(JSON.stringify(res.body)) }) .catch(function(err) { console.log(err.message) }); } }
Http
prefix:
import Felgo import QtQuick App { Component.onCompleted: { var p1 = Promise.resolve(3); var p2 = 1337; var p3 = HttpRequest .get("http://httpbin.org/get") .then(function(resp) { return resp.body; }); var p4 = Promise.all([p1, p2, p3]); p4.then(function(values) { console.log(values[0]); // 3 console.log(values[1]); // 1337 console.log(values[2]); // resp.body }); } }
Promises are a convenient and flexible way to handle asynchronous aspects of your application.
The following example shows the configured entries of the ListModel in a ListPage, and allows to sort the list using the name
property:
import Felgo import QtQuick App { // data model ListModel { id: fruitModel ListElement { name: "Banana" cost: 1.95 } ListElement { name: "Apple" cost: 2.45 } ListElement { name: "Orange" cost: 3.25 } } // sorted model for list view SortFilterProxyModel { id: filteredTodoModel sourceModel: fruitModel // configure sorters sorters: [ StringSorter { id: nameSorter roleName: "name" }] } // list page NavigationStack { ListPage { id: listPage title: "SortFilterProxyModel" model: filteredTodoModel delegate: SimpleRow { text: name detailText: "cost: "+cost style.showDisclosure: false } // add checkbox to activate sorter as list header listView.header: AppCheckBox { text: "Sort by name" checked: nameSorter.enabled updateChecked: false onClicked: nameSorter.enabled = !nameSorter.enabled anchors.horizontalCenter: parent.horizontalCenter height: dp(48) } } // ListPage } // NavigationStack } // App
Please see the documentation of SortFilterProxyModel for more details and relevant types.
true
only after a short delay. This is required, as otherwise the network adapter might not be
ready yet, which can cause immediate network requests to fail.
Highlights Blog Post: Release 2.18.0: Update to Qt 5.11.1 with QML Compiler and Massive Performance Improvements
Felgo 2.18.0 updates to Qt 5.11.1 and adds several improvements and fixes.
Felgo 2.18.0 comes as a free update for all Felgo developers.
CONFIG += qtquickcompiler
Highlights Blog Post: Release 2.17.1: Use 3D with Live Reloading and Test Plugin Code Examples from Browser
Felgo 2.17.1 adds several improvements and fixes for Felgo components. It also includes a new app example, how to create custom listview delegates.
Felgo 2.17.1 comes as a free update for all Felgo developers.
|
|
Here is an example how to display an ad banner with the AdMob plugin:
import Felgo App { NavigationStack { Page { title: "AdMob Banner" AdMobBanner { adUnitId: "ca-app-pub-3940256099942544/6300978111" // banner test ad by AdMob banner: AdMobBanner.Smart } } } }
The following example displays a floating action button above the keyboard, that also adapts to size changes of the keyboard:
import Felgo import QtQuick App { id: app // We unset the focus from the AppTextField after the keyboard was dismissed from the screen onKeyboardVisibleChanged: if(!keyboardVisible) textField.focus = false NavigationStack { Page { id: page title: qsTr("Keyboard Height") AppTextField { id: textField width: parent.width font.pixelSize: sp(25) } FloatingActionButton { // Add the keyboard height as bottom margin, so the button floats above the keyboard anchors.bottomMargin: app.keyboardHeight + dp(15) // We only show the button if the AppTextField has focus and the keyboard is expanded visible: textField.focus && app.keyboardHeight != 0 icon: IconType.check backgroundColor: Theme.tintColor iconColor: "white" onClicked: textField.focus = false } } } }
.txt
or .json
anymore. This allows working with shaders in
the Felgo Hot Reload Client without any custom C++ code from QML.
itemId
as parameter that triggered the
signal.
The new phoneNumber property now works with Android run-time permissions.
Note: The methods getContacts() and getPhoneNumber() are deprecated as of Felgo 2.17.1. Use the new properties instead.
startAt
and endAt
on iOS.
Highlights Blog Post: Release 2.17.0: Firebase Storage, Downloadable Resources at Runtime and Native File Access on All Platforms
Felgo 2.17.0 adds two new demos that show how to integrate C++ code with QML and add the new DownloadableResource and FileUtils items.
Felgo 2.17.0 comes as a free update for all Felgo developers.
With the new FirebaseStorage item, you can upload files to the Firebase Cloud Storage. It uploads local files to the cloud file system and provides a public download URL.
You can upload files like the following:
In addition, the text and icon of tabs for TabControl or AppTabButton may now use a different color while being pressed. To take advantage of this feature in your app's navigation drawer entries and tabs, the Theme provides the following new settings:
true
.
import Felgo import QtQuick App { onInitTheme: { Theme.colors.tintColor = "red" // drawer + item bg Theme.navigationAppDrawer.backgroundColor = "lightgrey" Theme.navigationAppDrawer.itemBackgroundColor = Theme.tintColor Theme.navigationAppDrawer.itemSelectedBackgroundColor = "orange" Theme.navigationAppDrawer.itemActiveBackgroundColor = "white" // item text Theme.navigationAppDrawer.textColor = "white" Theme.navigationAppDrawer.selectedTextColor = "black" Theme.navigationAppDrawer.activeTextColor = Theme.tintColor // tab text Theme.tabBar.titleColor = Theme.tintColor Theme.tabBar.titlePressedColor = "orange" } Navigation { navigationMode: navigationModeTabsAndDrawer NavigationItem { title: "Home" icon: IconType.home NavigationStack { Page { title: "Home" } } } NavigationItem { title: "Favorites" icon: IconType.star NavigationStack { Page { title: "Favorites" } } } NavigationItem { title: "Help" icon: IconType.question NavigationStack { Page { title: "Help" } } } } }
false
. If you hide the button or use a customized profile, you can still provide this feature with the FelgoGameNetwork::deleteUser() function.
true
and running is also set to true
). The overall performance
also increased, as frames are no longer re-drawn if the animation is not running or paused.
You can do this either by assigning currentFrame or by calling advance().
file://
URLs on Android & iOS.
Please note that if your targetSdkVersion
in AndroidManifest.xml
is 24 or higher and you want to open file://
URLs with another application on Android devices, you need to enable
the paths you intend to use in the android/res/xml/file_paths.xml
file.
Highlights Blog Post: Release 2.16.1: Live Code Reloading with Custom C++ and Native Code for Qt
Felgo 2.16.1 features QML code reloading with custom C++ and native code for Felgo and Qt projects.
Starting with Felgo 2.16.1, the app's bundle identifier for iOS is set using the PRODUCT_IDENTIFIER
setting in your *.pro
project configuration. To adapt to the new setting perform the following
steps:
1. Open your *.pro
project file and add the following block:
# configure the product's bundle identifier # this identifier is used for the app on iOS PRODUCT_IDENTIFIER = com.your.company.YourApp
where com.your.company.YourApp
matches the CFBundleIdentifier
setting of your existing Project-Info.plist
configuration.
2. Open your Project-Info.plist
in the ios
subfolder and replace the value of CFBundleIdentifier
with $(PRODUCT_BUNDLE_IDENTIFIER)
, so that it looks like the following:
<key>CFBundleIdentifier</key> <string>$(PRODUCT_BUNDLE_IDENTIFIER)</string>
3. After running qmake, your iOS build uses the new setting.
Note: Make sure to transition your project to this new format, as otherwise app store build uploads from Xcode 9 might fail.
All Felgo Project Wizards already use the new project setting.
Felgo version 2.16.1 introduces the Felgo Hot Reload Module. You can use it to add QML code reloading features to your own application. This allows you to use custom C++ and native code together with code reloading for QML and JavaScript. You can find a quick integration guide in the update blog post.
You can style the badge globally with the Theme::listItem properties or specifically for a row instance with the SimpleRow::style property:
You can style the badge with the Theme::navigationAppDrawer properties:
Highlights Blog Post: Release 2.16.0: iPhone X Support and Runtime Screen Orientation Changes
Felgo 2.16.0 brings iPhone X support and runtime screen orientation changes.
The iPhone X comes with new UI challenges, as the screen is now bigger and includes reserved areas that hold native buttons or overlays. The Felgo Navigation components automatically adjust their style to match these new requirements. In addition, the AppPage::useSafeArea setting takes care of keeping your UI elements within the safe area of the screen.
Alternatively, you can also disable the setting and manually align your content to the AppPage::safeArea item. The exact pixel insets of the screen are available with the NativeUtils::safeAreaInsets property.
To support existing games on devices with reserved screen areas, the Scene::useSafeArea setting keeps your scene content within the safe area. The Scene::gameWindowAnchorItem reflects this safe area of the screen.
To provide the best look and experience, you can take advantage of the new Scene::fullWindowAnchorItem. You can then e.g. let your background fill the whole screen, without affecting other parts of the game.
The exact pixel insets of the screen are available with the NativeUtils::safeAreaInsets property. If used within a Scene, keep in mind that these insets do not take scene-scaling into consideration.
To speed up development, you can test your UI for iPhone X on Desktop without having to build and deploy for the iPhone Simulator.
The Desktop simulation for iPhone X also covers the native safe area. You can quickly switch between different devices to see how your app looks like with and without safe area insets.
To specify the global orientation you can use the new property NativeUtils::preferredScreenOrientation. E.g. to lock and unlock your app or game to portrait orientation, you can use the following lines of code:
import Felgo import QtQuick App { NavigationStack { Page { title: "Orientation Lock" Column { AppButton { text: "Lock to portrait" onClicked: NativeUtils.preferredScreenOrientation = NativeUtils.ScreenOrientationPortrait } AppButton { text: "Reset default orientation" // Resets to the orientation defined in AndroidManifest.xml / Project-Info.plist onClicked: NativeUtils.preferredScreenOrientation = NativeUtils.ScreenOrientationDefault } } } } }
You can also specify the orientation per page in apps, using AppPage::preferredScreenOrientation. Use this example code to show a page fixed to portrait mode:
import Felgo import QtQuick App { id: app NavigationStack { id: stack Page { id: page title: "Screen orientation test" AppButton { text: "Push portrait page" onClicked: stack.push(portraitPage) } } } Component { id: portraitPage Page { id: page title: "Portrait page" preferredScreenOrientation: NativeUtils.ScreenOrientationPortrait } } }
These properties only have an effect on iOS and Android and override the default screen orientation defined in your project's Project-Info.plist
and AndroidManifest.xml
.
false
for phablets and phones with bigger screens like the iPhone X or Samsung Galaxy S8+.
import Felgo
only. for NativeUtils anymore if you are only using app
components).
false
.
false
.
targetSdkVersion >= 24
in AndroidManifest.xml
, it needs to use a content provider so the camera app can save the image to the app.
For new projects created in Qt Creator, everything is already configured properly.
If you have an existing project and are updating targetSdkVersion
to 23 or greater, you need to add the following code to android/AndroidManifest.xml
inside the <application>
tag:
<!-- file provider needed for letting external apps (like camera) write to a file of the app --> <provider android:name="android.support.v4.content.FileProvider" android:authorities="${applicationId}.fileprovider" android:exported="false" android:grantUriPermissions="true"> <meta-data android:name="android.support.FILE_PROVIDER_PATHS" android:resource="@xml/file_paths"/> </provider>
Additionally you need to create a file android/res/xml/file_paths.xml
with the following contents:
<?xml version="1.0" encoding="utf-8"?> <paths> <external-files-path name="images" path="Pictures"/> </paths>
Finally, add the following dependencies to android/build.gradle
:
dependencies { ... implementation 'com.android.support:support-core-utils:27.1.0' implementation 'com.android.support:appcompat-v7:27.1.0' }
getPrice()F
method in log output when using Soomla Plugin.
Highlights Blog Post: Release 2.15.1: Upgrade to Qt 5.10.1 & Qt Creator 4.5.1 | Firebase Improvements and New Live Code Reloading Apps
Felgo 2.15.1 updates to Qt 5.10.1 & Qt Creator 4.5.1 and adds new APIs for the Firebase Plugin.
Highlights Blog Post: Release 2.15.0: Upgrade to Qt 5.10 & Qt Creator 4.5 | Firebase Data Structures and Queries & Many Improvements
Felgo 2.15.0 updates to Qt 5.10
Example:
FirebaseDatabase { id: firebaseDb Component.onCompleted: getValue("public/path/to/my/object") onReadCompleted: { if(success) { //signal parameter "value" can be a nested object/array as read from your database console.debug("Read value " + value.subarray[3].subproperty.text) } } }
queryProperties
to the functions FirebaseDatabase::getValue() and FirebaseDatabase::getUserValue().
Example:
//use query parameter: firebaseDb.getValue("public/bigqueryobject", { orderByKey: true, //order by key before limiting startAt: "c", //return only keys alphabetically after "c" endAt: "m", //return only keys alphabetically before "m" limitToFirst: 5, //return only first 5 sub-keys })
See more details about the available query parameters at the documentation of FirebaseDatabase::getValue().
source
property for NavigationItem.
Highlights Blog Post: Release 2.14.2: Live Code Reloading with Native Cross-Platform Plugins
Felgo 2.14.0 introduced Felgo Live Code Reloading, which reduces your deployment time from minutes to seconds. Felgo 2.14.2 now adds support for using native cross-platform plugins with live code reloading.
Felgo 2.14.2 adds new SocialView types, fixes the App Navigation issue with signal handlers and updates Felgo QML Hot Reload Client apps to the latest versions. With this update, Live Code Reloading allows to run and reload your projects on iOS and Android from all desktop platforms. No native iOS and Android build tools required.
For a guide how to best use the SocialView in your projects, please see How to Add a Messenger or Leaderboard to your Mobile App.
The updated Qt World Summit 2017 app on GitHub also includes a BusinessMeet search. It shows how to use the new features to search and find users based on stored custom data like the main Qt interest or company of the user.
The new ThemeNavigationBar::backgroundImageFillMode and NavigationBar::backgroundImageFillMode properties allow to define different fillModes for the background image.
Highlights Blog Post: Release 2.14.1: Update to Qt 5.9.3 | Use Live Code Reloading on macOS and Linux
Felgo 2.14.1 adds Live Code Reloading Support for macOS & Linux Desktop. With this addition, Live Code Reloading now allows to run and reload your projects on iOS and Android from all desktop platforms. No native iOS and Android build tools required.
Felgo 2.14.1 also upgrades to Qt 5.9.3, introduces the new SocialView item for social features like user profile, leaderboard or even a full-featured messenger, and extends the App Navigation capabilities with NavigationItem::showItem.
This update adds the Felgo Hot Reload extension for macOS and Linux.
To show a default UI for these social services, the GameNetworkView and MultiplayerView offer with a simple state-based approach. However, the SocialView type uses a different approach to integrate such services in your apps or games and has many advantages:
For a full SocialView integration example, please see the updated source code of the Qt World Summit 2017 demo on GitHub.
NavigationItem { title: "Only on iOS" icon: IconType.apple showItem: Theme.isIos // ... }
Highlights Blog Post: Release 2.14.0: Live Code Reloading for Desktop, iOS & Android
Felgo 2.14.0 adds Live Code Reloading for Desktop, iOS & Android. It allows to run and reload your projects within a second on iOS and Android from Windows. No Mac and Android SDKs required.
As already done with Desktop platforms and Felgo 2.13, we now use custom Android & iOS packages that make future updates easier. To switch to the new packages, perform these steps after updating the Felgo SDK:
Unselect the Qt 5.9.2 package like shown below:
With update 2.14.0, we updated all our wizards to be compatible with iOS apps submitted to the App Store with Xcode 9.
Note: If you still use a previous version of Xcode (e.g. Xcode 8), you can either optionally perform these steps now or perform them as soon as you're updating to Xcode 9. You can still use Xcode 8 or earlier after migrating your project.
To migrate your existing project to the new format, perform the following steps:
1. Create a new "Felgo Apps Empty Application" project from the "New Project" wizard within Qt Creator
2. Copy over the folder ios/Assets.xcassets
from the new empty project to your project's ios
subfolder.
3. Replace all the icon files and launch images found within Assets.xcassets/AppIcon.appiconset/
and Assets.xcassets/LaunchImage.launchimage/
with your previous project's icons and launch images.
Make sure to match all filenames and images sizes.
Note: To get the correct names and sizes you can use the online service https://www.appicon.build.
4. You can now remove all AppIcon*.png
and Def*.png
files from your ios
folder, they are not used anymore.
5. As a last step, open the ios/Project-Info.plist
file and remove the keys (and their respective values) CFBundleIconFile
, CFBundleIconFiles
, UILaunchImageFile
and
UILaunchImages
.
Your project now uses the asset catalog and you're ready for submitting your apps to the App Store with Xcode 9 again.
Highlights Blog Post: Release 2.13.2: Update to Qt 5.9.2 & Qt Creator 4.4.1 | Bug Fixes & Performance Improvements
Felgo 2.13.2 adds support for Qt Creator 4.4.1 and Qt 5.9.2 which fixes Android deployment issues with the latest Android SDK and NDK versions. It also stores your previous window position and size to accelerate the development process.
As Felgo 2.13.2 is based on Qt 5.9.2, perform these steps after updating the Felgo SDK:
Add the Qt 5.9.2. packages for Android and iOS. iOS is not listed in this screenshot as it is not available on Windows. Unselect the Qt 5.9 package like shown in this image:
In addition, if you are using Felgo Plugins in your project, update the following files after updating Felgo SDK:
In your android/build.gradle
file, change the following lines from
buildscript { repositories { mavenCentral() } dependencies { classpath 'com.android.tools.build:gradle:3.6.0' } } ... allprojects { repositories { mavenCentral() maven { url 'https://install.felgo.com/maven/' } } }
to
buildscript { repositories { mavenCentral() } dependencies { classpath 'com.android.tools.build:gradle:3.6.0' } } ... allprojects { repositories { mavenCentral() google() maven { url 'https://install.felgo.com/maven/' } } }
Changes: com.android.tools.build:gradle
version changes from 2.1.0 to 2.3.3 and the maven.google.com
maven repository is added.
If your project includes a android/gradle/wrapper/gradle-wrapper.properties
file, make sure to update the version from
distributionUrl=https\://services.gradle.org/distributions/gradle-2.10-all.zip
to
distributionUrl=https\://services.gradle.org/distributions/gradle-3.3-all.zip
If you use the mutli-language translation features of Felgo, add the following snippet to your ios/Project-Info.plist
file:
<key>CFBundleAllowMixedLocalizations</key> <true/>
Unless you add that flag, your app will only be translated to the default iOS project language on iOS 11 (usually English).
If you use GPS in your app app, for example if you use the AppMap component, make sure that the following two keys exist in your ios/Project-Info.plist
file (adapt the string
values to your use case in your app):
<key>NSLocationWhenInUseUsageDescription</key> <string>Display your location on the map.</string> <key>NSLocationAlwaysAndWhenInUseUsageDescription</key> <string>Display your location on the map.</string>
Note: These changes are required to use the latest available features with Android Oreo & iOS 11. As usual, you can create a new empty Felgo project from our wizards or use the Felgo demos to compare your project settings with the expected ones.
Highlights Blog Post: Release 2.13.1: Qt Creator 4.4 Upgrade, Mac & Linux Improvements
Felgo 2.13.1 adds support for Qt Creator 4.4 and brings improvements for developers using Mac & Linux. There are also various other fixes and new improvements, for example for the Flurry and OneSignal Push Notification plugin.
Felgo 2.13.1 is a free update for all Felgo developers.
Follow these steps on macOS and Linux because otherwise you will not be able to use the new features of this update. This is a one-time step required to make updating Felgo versions easier in the future:
1.) After you updated Felgo, open Qt Creator and click on the "Projects" icon on the left. You will now see the new "Felgo Desktop" kit greyed out. Then double-click on the greyed out Felgo kit on the left.
After double-clicking on the (new) grey kit, it will be used by default for your project:
2.) To also set the new Felgo kit as the default for your future projects, click on Qt Creator / Preferences on macOS or Tools / Options on Linux from the menu bar and select Build & Run. Then select the Felgo Kit and click on the "Make Default" button. Now the new Felgo kit will be the default for all your future projects and it will look like this:
Also, the nickname users can enter in the Felgo Game Network ProfileView, will now be stored as a push notification tag for this user. This allows you to send messages to users based on this additional information.
You can see these new additions in the OneSignal Dashboard. In this screenshot, you can see the "Player ID" which is now available with the OneSignal::userId property. And the new tag "_vpgnnickname" stored for users who entered a manual nickname:
Highlights Blog Post: Release 2.13.0: Free Rewarded Videos & Native Ads for AdMob and Qt
Felgo 2.13.0 adds rewarded videos and native ads as two more ad types to the free AdMob Plugin. This allows you to better monetize your app or game and earn more revenue from your app! This update also adds support to get the phone contact list and to query the phone number of the device on Android.
Follow these steps on Windows, because otherwise you will not be able to use the new features of this update but still are on the previous Felgo version 2.12.2. This is a one-time step required to make updating Felgo versions easier in the future:
1.) After you updated Felgo, open Qt Creator and click on the "Projects" icon on the left. You will now see the new “Felgo Desktop” kit greyed out. Then double-click on the greyed out Felgo kit on the left.
After double-clicking on the (new) grey kit, it will be used by default for your project:
2.) To also set the new Felgo kit as the default for your future projects, click on Tools / Options and select Build & Run. Then select the Felgo Kit and click on the "Make Default" button. Now the new Felgo kit will be the default for all your future projects and it will look like this:
Highlights Blog Post: Release 2.12.2: Firebase Realtime Listeners & Qt Creator Designer Icons
Felgo 2.12.2 adds support for Realtime Database Listeners to the Firebase Plugin, which allows your app to get notified whenever your data changes. In addition, the recently improved Qt Creator Designer now comes with icons for all Felgo types to let you distinguish the different components more easily.
The Firebase Realtime Database is a cloud-hosted database. Data is stored as JSON and changes to the database are propagated to other clients in real time. This update adds new properties and signals to the FirebaseDatabase item, which you can use to add listeners for certain database entries. Your app is then notified immediately after your data in the cloud gets changed - no matter which device, user or application changed the value.
The following example uses the FirebaseDatabase::realtimeValueKeys property and FirebaseDatabase::realtimeValueChanged signal to keep track of a public database entry newstext
:
import Felgo App { FirebaseDatabase { realtimeValueKeys: ["public/newstext"] onRealtimeValueChanged: { if(success) { console.debug("Public Realtime Value '" + key + "' changed to '" + value + "'") // if newstext got changed -> update AppText to show news if(key === "newstext") news.text = value } else { console.debug("Error with message: " + value) } } } AppText { id: news text: "" // no news by default, news is loaded from database and always up-to-date } }
This example already shows how powerful this new feature can be. As soon as the newstext
entry changes in the database, all clients will get notified and replace the old news. As Firebase also supports authentication and user access rules, you could even create a real-time chat with this new feature. The possibilities are limitless!
For a quick guide how to use the new Qt Quick Designer with Felgo, have a look at our tutorial video:
This tutorial video shows how to create mobile apps with Felgo using the Qt Quick Designer of Qt Creator.
Get Started | 14min | Essential
Highlights Blog Post: Release 2.12.1: Improved Visual Editor & Qt Quick Designer
Felgo 2.12.1 adds improved support for the new Qt Quick Designer of Qt Creator 4.3, which allows to write QML code side-by-side with a visual representation of the code. The designer now better supports rendering Felgo Components and all core components are available for drag'n'drop in the side menu.
This is why we now improved compatibility of Felgo Components with the Qt Creator Designer, which allows all Felgo developers to fully utlize these new IDE features! All Felgo core components are now available in the side menu of the designer for easy drag'n'drop as soon as you add the Felgo import to your QML file. In addition, the designer now better supports rendering Felgo components. Especially the Felgo Apps Navigation Components have undergone a big improvement regarding designer support.
Creating amazing user interfaces with Felgo has never been easier!
Highlights Blog Post: Release 2.12.0: Qt 5.9 & Qt Creator 4.3 Support
Felgo 2.12.0 adds support for Qt 5.9 and the latest Qt Creator 4.3. Qt 5.9 adds many great features like support for gamepads and 3D animations. Qt Creator 4.3 adds a much improved design mode, which allows you to write QML code side-by-side with a visual representation of the code. This allows rapid prototyping of UIs. Some of the Felgo components now also support the Qt Quick Designer and visual development to further accelerate the development process.
Felgo 2.12.0 upgrades to Qt 5.9 and Qt Creator 4.3, which allows you to use all latest Qt features in your Felgo projects.
Please note the following points for a successful switch to Qt 5.9:
ios/Project-Info.plist
now includes the NSContactsUsageDescription
entry, which is
required for NativeUtils::storeContacts() since iOS 10.
Highlights Blog Post: Release 2.11.0: All Monetization Plugins now Free, Plugin Trial & Firebase Qt Plugin
Felgo 2.11.0 adds many changes to Felgo Plugins: all monetization plugins for in-app purchases and ads are now free to use in all licenses! This allows all developers in the Personal license to earn revenue with your app or game, no paid license required.
You can now also use all the other plugins for evaluation in a Trial mode. Integration of plugins is now a lot easier thanks to new wizards for adding plugins with Qt Creator.
The Google Firebase Plugin is a new plugin for user authentication and to access the Firebase Realtime Database.
When you add a plugin a Felgo Plugin Trial Watermark is displayed and an alert dialog is shown when the plugin is used. Specify a GameWindow::licenseKey or App::licenseKey to remove this trial with your Pro plan and to activate the used plugins.
Choose your plugins and create a license key for your application here. To gain access to all Felgo Plugins, upgrade to Felgo Pro.
To create a project using this wizard, open Qt Creator and choose "New Project", then select Plugin Application in the Felgo Apps section or Game with Plugins in the Felgo Games section.
After choosing the plugins to include, please make sure to perform all integration steps described in the plugin documentation.
In a similar way, the existing project wizards for Felgo Games and Apps now also support adding Felgo Plugins.
To create a new Plugin Item, choose "New File or Project" and choose Felgo Plugin in the Felgo Games or Felgo Apps files section.
Choose a name for the new item and select which plugin you want to use. Then add the new item to your main QML and run the project to view the plugin example.
Before you can use the plugin, it is required to perform all integration steps described in the plugin documentation.
If players connect with Facebook and allow access to their friends list, they can directly compare scores with their Facebook friends or challenge them to multiplayer matches without the hassle of manually searching for their friends in the game.
accepted
parameter.
Highlights Blog Post: Release 2.10.1: Felgo Apps, TexturePacker & Qt 5.8 Improvements
Felgo 2.10.1 improves the OpenGL shader implementation on macOS when using TexturePacker and adds better compatibility with Qt 5.8.
The update also solves a possible issue on iOS and macOS that blocked the network access of the application after turning Wifi off and on again.
The threshold for setting tablet to true
also increases to 5.3
. This prevents tablet layout detection on bigger phones like the iPhone 7 Plus.
Highlights Blog Post: Release 2.10.0: New App Showcase, Multiplayer & Multi-Language Improvements, Qt 5.8
Felgo 2.10.0 adds a new Showcase App which combines all app demos in one application and adds a new demo with full source code for the most common requirements in mobile apps. Furthermore, Felgo Multiplayer now supports to invite players to running games. You can now add support for multiple languages easier than before. And use all the features like text-to-speech introduced in Qt 5.8 with Felgo.
Felgo 2.10.0 upgrades to Qt 5.8 and Qt Creator 4.2, which allows you to use all latest Qt features in your Felgo projects.
Please note the following points for a successful switch to Qt 5.8:
New Showcase App
iOS | Android |
---|---|
|
|
The improved component showcase covers the most useful Felgo features you might want to include in your own app. Have a look at the full source code in the updated Felgo Sample Launcher or download the app from the stores for iOS or Android.
The updated Component Showcase App combines the most important Felgo Apps features in a single app with native user experience (UX) and fluid UI animations:
iOS |
|
|
Android |
|
|
New Slider Controls
dp(3000)
which matches
the AppListView flick velocity.
false
.
true
.
If players connect with Facebook and allow access to their friends list, they can directly compare scores with their Facebook friends or challenge them to multiplayer matches without the hassle of manually searching for their friends in the game. In addition, the player profile with all scores, achievements and friends is synchronized if a player uses the same account on multiple devices.
Blog Post: Release 2.9.2: Qt World Summit 2016 App Showcase and New App Components
Felgo 2.9.2 comes with the Qt World Summit 2016 app showcase demo, adds a lot of new app components and updates the Facebook Plugin to use the latest available version.
Qt World Summit 2016 App Showcase
iOS | Android |
---|---|
|
|
We made a full featured conference management app for the recent Qt World Summit Conference that comes with many new components. Have a look at the full source code in the updated Felgo Sample Launcher or download the app from the stores for iOS or Android.
The Qt World Summit 2016 Conference App allows to:
The app also shows best practices how to use the Felgo Apps Components to create apps that:
iOS |
|
|
|
|
Android |
|
|
|
|
New Felgo Apps Components
While working on the Qt World Summit 2016 Conference App we also made many new components you can easily use in your own apps:
false
.
AndroidManifest.xml
file.
Blog Post: Release 2.9.1: Multiplayer Enhancements & Plugin Updates
Felgo 2.9.1 is an incremental maintenance update.
Rebranded Multiplayer Card Game
We rebranded our Multiplayer Card Game "ONU". This includes updated graphics for the whole game, custom cards that match the Felgo style and a new game title "One Card!". See the game overview page to learn where to find it in the stores or to have a look at the source code.
The new game version also includes many additional features like a single player mode, medals for high-level players and an increased max player level. It now also showcases the integration of In-App Purchases and Advertisements with the Soomla Plugin and the AdMob Plugin.
|
|
New Felgo Multiplayer Features
gameRestarted
parameter, which is set to true
for a restarted game. The signal gameEnded() informs all players that a game has ended. This feature requires two additional reserved messages codes: restartGameMessageId and endGameMessageId.
true
.
For a sample implementation of these new features, have a look at the "One Card!" Multiplayer Demo.
Multiplayer Introduction Blog Post: Release 2.9.0: Introducing the Improved Felgo Multiplayer Feature
Felgo 2.9.0 adds Felgo Multiplayer for easily creating real-time & turn-based multiplayer games with Felgo.
Felgo 2.9.0 upgrades to Qt 5.7 and Qt Creator 4.0, which allows you to use all latest Qt features also with Felgo projects.
Please note the following points for a successful switch to Qt 5.7:
minSdkVersion >= 16
setting. To successfully build your projects for Android, open the file android/AndroidManifest.xml of your project and make sure to
set at least:<uses-sdk android:minSdkVersion="16" ... />
Felgo Multiplayer is officially released.
Following its successful beta release, you can now access Felgo Multiplayer when you update Felgo – even as a free user. This new feature can be integrated into your game with less than 100 lines of code! For a quick preview of the Felgo Multiplayer features check out the Felgo Multiplayer YouTube Video.
Felgo Multiplayer Features
Component Overview
The following table gives an overview of all relevant Felgo Multiplayer components and what they are used for:
Component | Description |
---|---|
FelgoMultiplayer | Felgo Game Network component to add multiplayer features to your game. |
MultiplayerView | The default UI for FelgoMultiplayer matchmaking, friend selection, chat and game invites. |
NotificationBar | Base type for creating a notification bar to show incoming FelgoMultiplayer push notifications in-game. |
MultiplayerState | Item for handling the possible states of FelgoMultiplayer. |
MultiplayerUser | Represents a single Felgo Multiplayer user. |
NoRanking | Can be used as the rankingStrategy to disable the ranking feature. |
SimpleRanking | Defines a simple multiplayer ranking strategy. It is the default rankingStrategy. |
Felgo Multiplayer also integrates seamlessly with the FelgoGameNetwork. For showing leaderboards that include a Friends section or to let the users customize their profile it is sufficient to add a GameNetworkView like you would for games without multiplayer features. Each MultiplayerUser is essentially also a GameNetworkUser with leaderboard scores and achievements.
|
|
New Demo Game and Examples
Felgo Multiplayer has already been used to launch "One Card!", a successful 4-player card game based on the popular UNO game. "One Card!" is available on the App Store and Google Play Store and has garnered 100,000+ downloads in the first month. The player retention rates and engagement metrics are also way above the industry standard, thanks to the multiplayer features. Thus you as developers can use the full source code as a best practice for multiplayer integration and create your own multiplayer games within a few days.
|
|
The full source code for "One Card!" is available for free in the Felgo SDK. See One Card! - Multiplayer Card Game for an overview of all game features and to find the full source code of the game.
The new Felgo release also contains two more Multiplayer Examples:
All of the demos and examples are included in the Felgo Sample Launcher.
Highlights Blog Post: Release 2.8.6: Multiplayer Game "One Card!" & New Native Features
Felgo 2.8.6 adds a new multiplayer card game and new native features like storing a contact in the address book and opening an apps page in the native App Store to help get app store ratings for your app or cross-link to other apps.
vCard
) or launch apps on the device with
NativeUtils::openApp(launchParam
). The NativeUtils::openApp() function is useful to get an app store
rating for your app or cross-link to other apps.
system.osType
and system.osVersion
and retrieve the device model identifier with NativeUtils::deviceModel().
Highlights Blog Post: Release 2.8.5: Image Upload to Server and Native Image Picker
Felgo 2.8.5 adds the functionality to upload pictures to our web backend and adds a native image picker from camera or the picture gallery.
For changing the user image in your own views use the following FelgoGameNetwork functions:
2.0
on desktop to match the default App::uiScale.
Highlights Blog Post: Release 2.8.4: Google Cloud Messaging Integration & Felgo Games Improvements
Felgo 2.8.4 adds the GoogleCloudMessaging Plugin and fixes several minor bugs.
Highlights Blog Post: Release 2.8.3: New GameCenter Plugin and Bug Fixes
Felgo 2.8.3 adds the GameCenter Plugin and fixes several minor bugs.
Highlights Blog Post: Release 2.8.2: Easier Plugin Integration & Debug Menu Improvements
Felgo 2.8.2 drastically improves integration of Felgo Plugins, as all plugins are now part of Felgo. If you want to use the new plugin integrations please have a look at our migration guide.
Note: Important note for Android users: If you're using our existing projects with Felgo 2.8.2, please perform the changes to your
AndroidManifest.xml
file as described here.
navigationModeNone
.
Highlights Blog Post: Release 2.8.1: Spine Animations with Felgo & Qt
Felgo 2.8.1 adds support for Spine animations, offers new file wizards and fixes some compatibility issues with Qt 5.6.
There's also a new Spine example in the Felgo Sample Launcher that shows how to use the converted Spine entities. You can find in it the Examples/Sprites
category.
CONFIG += felgo_debug
in your project file).true
.
Highlights Blog Post: Release 2.8.0: New Getting Started Tutorial, Sample Game & App Example
This Felgo release uses Qt 5.6. If you update your Felgo installation through the Felgo MaintenanceTool, Qt 5.6 will be automatically installed in parallel to your 5.5 installation.
If you don't need Qt 5.5 for your custom Qt projects anymore, you should uninstall it by opening MaintenanceTool, select "Add or remove components", uncheck all Qt 5.5 packages in the package tree and continue until finished:
Afterwards, make sure that you are using Qt 5.6 kits for you Felgo projects when opening in Qt Creator.
See here to learn more about the Qt 5.6 features.
Just browse the latest Felgo SampleLauncher to find the full source code or try the game.
These properties should be used instead of width and height, which refer to the total window size that may also include the Felgo debug menu bar.
These properties should be used instead of width and height, which refer to the total window size that may also include the Felgo debug menu bar.
popAll
and popAllAndPush
got renamed to popAllExceptFirst
and popAllExceptFirstAndPush
.
white
for the Android theme.
Highlights Blog Post: Release 2.7.1: IDE Improvements, More Code Snippets and Updated Documentation
Highlights Blog Post: Release 2.7.0: New App Components, Qt Maps Demo & Project Templates
Felgo 2.7.0 comes with lots of improvements for Felgo Apps components: You get new components, wizards and a brand new demo showing how to use map-based features within your apps.
It also adds a platformer level editor that enables you to create your own platform game within days.
With the new Platformer with Level Editor, you can see the full source code of a platformer game with integrated level editor and use it to create your own platformer.
Thanks to the in-game level editing support, the demo game also includes support for user-generated content: Players can create and share their own levels with other players around the world. This enables you to create a game like Super Mario Maker or Minecraft.
The new clearsOnBeginEditing property allows clearing out existing content as soon as the input control gains focus (as an example, this is a common pattern for password input fields).
The new property showClearButton displays a button to quickly clear out entered content by the user without the need of using the backspace key.
PictureViewer provides two new signal handlers opened and closed and the new property isOpen to handle state changes when opening or closing the picture viewer.
Highlights Blog Post: Release 2.6.2: Android Material Design, New Documentation
Felgo Games
Additional Features:
Fixes:
Felgo Apps
Additional Features:
Fixes:
Highlights Blog Post: Release 2.6.1: Change Native UI At Runtime & Felgo Demos in Qt Creator
Additional Features:
Highlights Blog Post: Release 2.6.0: Multiplayer, Felgo Apps, TexturePacker, RUBE
Additional Features:
Highlights Blog Post: Announcing Improved App Development with Felgo Apps!
Additional Features:
Highlights Blog Post: Release 2.5.1: New Puzzle Game like 2048, New Slot Game and Slot Game API & Advanced Match-3 Game
Additional Features:
system.felgoVersion
property for getting the runtime library version of Felgo.Fixes:
LSRequiresIPhone
flag to .plist
files.Highlights Blog Post: 3D Games with Qt 5.5 and New Felgo Release 2.5.0
Additional Features:
API Changes:
Highlights Blog Post: Update 2.4.2: New Action & Falldown Sample Games, License Update
Additional Features:
API Changes:
Fixes:
Additional Features:
system.pauseGameForObject()
and system.resumeGameForObject()
to pause all timers and animations for all children of the given object.API Changes:
NonConsumableGood
was removed -
use goods of type LifetimeGood instead.
Fixes:
Highlights Blog Post: Update 2.4.0: Felgo Sample Launcher, Game Tour & New Platformer Game
Additional Features:
Fixes:
Highlights Blog Post: Update 2.3.1: Felgo Qt 5 Plugin Import Changes, RayCast Support
Additional Features:
API Changes:
import Felgo.plugins.*
to import Felgo.*
.
Fixes:
Highlights Blog Post: Update 2.3.0: Full Support for Qt 5.4, Windows Phone & Windows Runtime
Additional Features:
accepted
parameter will be false.API Changes:
Fixes:
Highlights Blog Post: Update 2.2.0: Density Independence & Multi-Screen Support, New Flappy Bird Tutorial & Android Back Button Handling
Additional Features:
API Changes:
Fixes:
Highlights Blog Post: Update 2.1.0: Major Qt 5 Release, Felgo 2 for All Developers
Additional Features:
Fixes:
felgo.qmltypes
file.Highlights Blog Post: Windows Phone 8 and Windows Runtime Support in Felgo and Qt, Gamescom & GDC Meetup
Additional Features:
API Changes:
Fixes:
Q_EXPORT_PLUGIN2
from static Felgo library to allow combining it with other QQmlExtensionPlugin objects in one project.Highlights Blog Post: Update 2.0.3: Big Squaby 2 Update, Qt 5.3.1 Support, AI & Particle Components
Additional Features:
felgoScheduler.schedulingMethod
to fixed timestep bound to frame rate which has much better visual appearance for MovementAnimation and physics system than
previous default value (FelgoScheduler.Accumulated).
API Changes:
Qt.resolvedUrl()
.
Fixes:
zoomToBiggerSide
and zoomNonUniform
.
Highlights Blog Post: Update 2.0.2: In-Game Level Editor & more Sample Games
Additional Features:
mirrorX
and mirrorY
properties to GameAnimatedSprite, GameSpriteSequence and MultiResolutionImage.
Fixes:
Highlights Blog Post: Big Felgo Update with Qt 5.3 Support
Additional Features:
Ctrl
(or Cmd
on Mac) + 1-7
and quick quit of application with the Escape
key.Highlights Blog Post: Felgo 2.0 Release with Full Qt 5 Support
Additional Features:
API Changes:
import Felgo
and import QtQuickx
to import Felgo
and import QtQuick
Note: For a list of all changes between Felgo 1.x and Felgo 2.0 see the Forum Thread How to port existing Qt 4 / Felgo 1.0 games to Felgo 2.0.
Highlights Blog Post: Update 1.5.6: New Sample Game "Chicken Outbreak 2", Last Update in Version 1
Additional Features:
Fixes:
Highlights Blog Post: Update 1.5.5: User-Generated Content, Level Sharing & Level Store
Additional Features:
posChange
and valueChange
to
FelgoGameNetwork::onNewHighscoreAfterServerApproval.
API Changes:
Fixes:
Highlights Blog Post: How to Increase Player Retention with Felgo Game Network
Additional Features:
translation.systemLanguage
property which stores the system language and locale.Fixes:
info.plist
given.Additional Features:
Fixes:
Highlights Blog Post: Update 1.5.2: Android Background Fixes
Additional Features:
Fixes:
Highlights Blog Post: Update 1.5.1: Performance Improvements & Customer Request Additions
Additional Features:
mirrorX
and mirrorY
property to SingleSpriteFromFile and SingleSpriteFromSpriteSheet.API Changes:
Fixes:
musicEnabled
setting in GameWindow::settings into consideration when resuming and pausing background music for apps going into foreground/background.Highlights Blog Post: Update 1.5: Chartboost & AdMob, MinGW, Ubuntu13 and More
Additional Features:
Ctrl + F
.translation.useSystemLanguage
property to allow to always set the system language and overwrite the custom language setting.API Changes:
1-7
to Ctrl(Cmd) + 1-7
.Fixes:
false
to avoid collisions when created from EntityManager::createPooledEntitiesFromComponent() or EntityManager::dynamicCreationEntityList.
Highlights Blog Post: Update 1.4: BlackBerry 10, DownloadablePackages, Desktop Publishing and More
Additional Features:
platforms
property for config.json to limit the builds performed on the Felgo Build Server.API Changes:
Fixes:
Highlights Blog Post: Heavy Loading Time Improvements, New Synmod Showcase Game, Demo Game Overhaul
Additional Features:
import Felgo
.
accessToken
and update to latest Android SDK 3.0.2.declarativeView()
method to FelgoApplication C++ class to be able to register custom items and declare custom context properties on desktop platforms.API Changes:
Fixes:
Highlights Blog Post: Support for Multiple Languages and In-App Purchases
Additional Features:
API Changes:
Fixes:
Additional Features:
Additional Features:
API Changes:
Fixes:
settings.particlesEnabled
for GameWindow::settings is now possible.Additional Features:
clip
property in the Flickable
element.
Examples/FelgoTests/basicTests/TextInputTest.qml
.
API Changes:
entityType_variationType_entityCount
instead of the verbose entity qml url
before.
Fixes:
Free
now also works if the Particle element is rotated, not only when its parent is rotated.
Additional Features:
CONFIG += felgo
in the pro file.Fixes:
Additional Features:
API Changes:
transformOrigin
of EntityBase is changed to Item.TopLeft
from the default value Item.TopCenter
, because children of items will always use
TopLeft
as their default (see the examples and demos).
placeholder
parameter which gets overwritten when the user starts typing and a
text
property for pre-filled text the user can modify. In previous version, only the placeholder text was possible.
Fixes:
Additional Features:
sdk
property to Deploying Felgo Games & Apps documentation.
orientation
options to auto-rotate the display by 180 degrees on iOS & Android: sensorPortrait
and sensorLandscape
to Deploying Felgo
Games & Apps documentation.
API Changes:
Fixes:
Additional Features:
Additional Features:
API Changes:
Fixes:
Limitations:
Additional Features:
API Changes:
Fixes:
Limitations:
Additional Features:
Fixes:
Limitations: